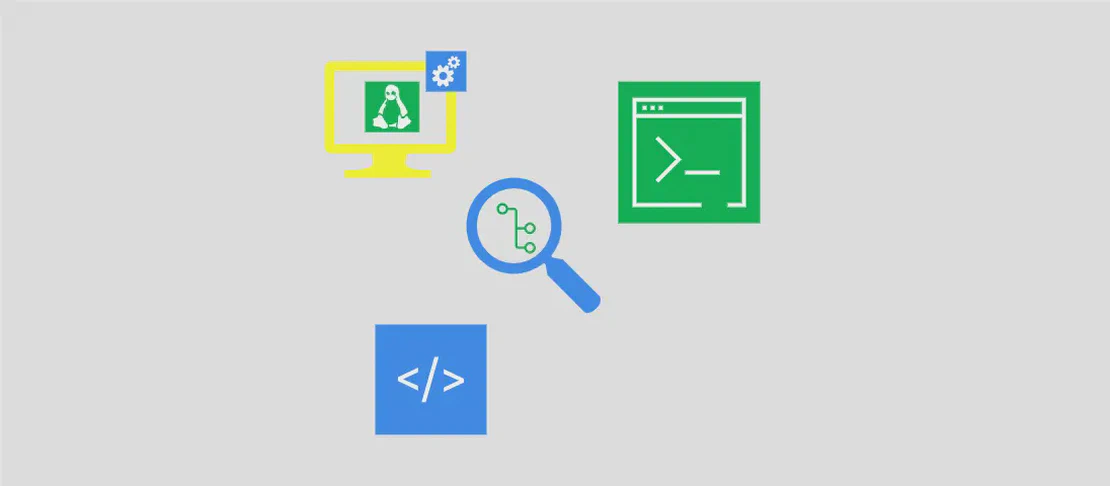
cp Command (with examples)
- Linux
- November 5, 2023
Using the cp command to copy files and directories.
The cp
command is used to copy files and directories in Linux. It allows you to create copies of files and directories, either in the same location or in a different destination. In this article, we will explore various use cases of the cp
command with code examples.
1: Copy a file to another location
The cp
command can be used to copy a file to another location. This can be useful in scenarios where you want to create a backup or make a duplicate of a file.
Code:
cp path/to/source_file.ext path/to/target_file.ext
Explanation:
path/to/source_file.ext
is the path to the file you want to copy.path/to/target_file.ext
is the path where you want to copy the file.
Example:
Suppose you have a file named document.txt
in the directory /home/user/documents
. You want to create a copy of this file in the directory /backup
.
cp /home/user/documents/document.txt /backup/document.txt
Output:
A copy of the file document.txt
will be created in the directory /backup
.
2: Copy a file into another directory, keeping the filename
Sometimes, you may want to copy a file into another directory while keeping the same filename. This can be useful when organizing files or moving them to a different directory without changing their names.
Code:
cp path/to/source_file.ext path/to/target_parent_directory
Explanation:
path/to/source_file.ext
is the path to the file you want to copy.path/to/target_parent_directory
is the path to the directory where you want to copy the file. The copied file will have the same name as the source file.
Example:
Suppose you have a file named image.jpg
in the directory /home/user/pictures
. You want to copy this file to the directory /backup/pictures
.
cp /home/user/pictures/image.jpg /backup/pictures
Output:
A copy of the file image.jpg
will be created in the directory /backup/pictures
.
3: Recursively copy a directory’s contents to another location
The cp
command can also be used to copy the contents of a directory to another location. This includes all files and subdirectories within the source directory. If the destination directory already exists, the source directory is copied inside it.
Code:
cp -r path/to/source_directory path/to/target_directory
Explanation:
-r
is an option that stands for “recursive”. It tells thecp
command to copy the directory and its contents recursively.path/to/source_directory
is the path to the directory you want to copy.path/to/target_directory
is the path to the directory where you want to copy the source directory and its contents.
Example:
Suppose you have a directory named photos
in the directory /home/user
. You want to create a backup of this directory in the directory /backup
.
cp -r /home/user/photos /backup
Output:
A copy of the directory photos
, along with all its contents, will be created in the directory /backup
.
4: Copy a directory recursively, in verbose mode
When copying a directory with a large number of files, it can be useful to see the files as they are being copied. The -v
option can be used to enable verbose mode, which provides more information during the copy process.
Code:
cp -vr path/to/source_directory path/to/target_directory
Explanation:
-v
is an option that stands for “verbose”. It tells thecp
command to operate in verbose mode, showing the files as they are copied.path/to/source_directory
is the path to the directory you want to copy.path/to/target_directory
is the path to the directory where you want to copy the source directory and its contents.
Example:
Suppose you have a directory named files
in the directory /home/user
. You want to create a backup of this directory in the directory /backup
, while seeing the files as they are being copied.
cp -vr /home/user/files /backup
Output:
The cp
command will display the names of the files as they are being copied, providing an overview of the copy process.
5: Copy multiple files at once to a directory
The cp
command allows you to copy multiple files at once to a directory. This can be useful when you have a list of files that you want to copy to a specific location.
Code:
cp -t path/to/destination_directory path/to/file1 path/to/file2 ...
Explanation:
-t
is an option that stands for “target directory”. It tells thecp
command that the destination directory is specified explicitly.path/to/destination_directory
is the path to the directory where you want to copy the files.path/to/file1
,path/to/file2
, and so on, are the paths to the files you want to copy.
Example:
Suppose you have three files named file1.txt
, file2.txt
, and file3.txt
in the directory /home/user/documents
. You want to copy these files to the directory /backup
.
cp -t /backup /home/user/documents/file1.txt /home/user/documents/file2.txt /home/user/documents/file3.txt
Output:
The three files file1.txt
, file2.txt
, and file3.txt
will be copied to the directory /backup
.
6: Copy text files to another location, in interactive mode
To prevent accidental overwriting of files, you can use the -i
option to enable interactive mode. This prompts the user for confirmation before overwriting any existing files in the target directory.
Code:
cp -i *.txt path/to/target_directory
Explanation:
-i
is an option that stands for “interactive”. It tells thecp
command to operate in interactive mode, prompting the user for confirmation before overwriting files.*.txt
is a wildcard pattern that specifies all files with the.txt
extension.path/to/target_directory
is the path to the directory where you want to copy the text files.
Example:
Suppose you have multiple text files with the .txt
extension in the directory /home/user/documents
. You want to copy these files to the directory /backup
, but you want to be prompted for confirmation before overwriting any existing files.
cp -i /home/user/documents/*.txt /backup
Output:
The cp
command will prompt you for confirmation before overwriting any existing files in the directory /backup
.
7: Follow symbolic links before copying
By default, the cp
command does not follow symbolic links when copying files. However, you can use the -L
option to follow symbolic links and copy the linked files instead.
Code:
cp -L link path/to/target_directory
Explanation:
-L
is an option that stands for “dereference symbolic links”. It tells thecp
command to follow symbolic links and copy the linked files instead of the links themselves.link
is the path to the symbolic link you want to copy.path/to/target_directory
is the path to the directory where you want to copy the linked files.
Example:
Suppose you have a symbolic link named mylink
that points to a file myfile.txt
in the directory /home/user/documents
. You want to copy the content of the linked file to the directory /backup
.
cp -L /home/user/documents/mylink /backup
Output:
The content of the linked file myfile.txt
will be copied to the directory /backup
.
8: Use the full path of source files, creating missing intermediate directories
The cp
command allows you to specify the full path of source files, creating any missing intermediate directories in the target location. This ensures that the file is copied to the desired location, even if the intermediate directories do not exist.
Code:
cp --parents source/path/to/file path/to/target_file
Explanation:
--parents
is an option that tells thecp
command to create any missing intermediate directories in the target location.source/path/to/file
is the full path of the file you want to copy.path/to/target_file
is the path where you want to copy the file.
Example:
Suppose you have a file document.txt
in the directory /home/user/documents/source
. You want to copy this file to the directory /backup/target
, creating any missing intermediate directories.
cp --parents /home/user/documents/source/document.txt /backup/target
Output:
The file document.txt
will be copied to the directory /backup/target
, and any missing intermediate directories (/backup
and /backup/target
) will be created if necessary.
Conclusion
In this article, we explored various use cases of the cp
command. We learned how to copy files to different locations, recursively copy directories, copy multiple files at once, use interactive mode, follow symbolic links, and create missing intermediate directories. The cp
command is a versatile tool that allows you to efficiently manage file and directory copies in Linux.