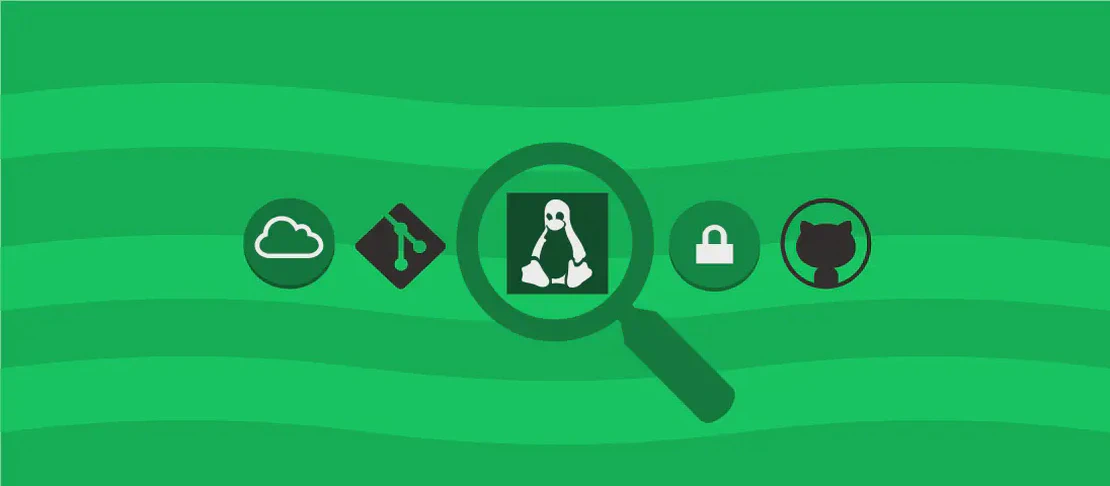
Mastering the Command 'cppcheck' (with Examples)
Cppcheck is a powerful static analysis tool used primarily for C and C++ code. Unlike traditional compilers that focus on identifying syntax errors, Cppcheck delves deeper to uncover potential bugs that are often overlooked. This tool aims to enhance your code by identifying issues that can affect performance, portability, and overall code quality. Its capabilities provide insights that can help developers preemptively address problems before they manifest during runtime. Below, we will explore several practical use cases to demonstrate its wide range of functionalities.
Use case 1: Recursively checking the current directory, showing progress and logging errors to a file
Code:
cppcheck . 2> cppcheck.log
Motivation: Developers often work on large projects with numerous files nested in different directories. In such scenarios, running a recursive check of the entire codebase can help identify issues spread across various files. The capability to log error messages into a separate file ensures that developers don’t miss any critical issues, as they can review them at their convenience.
Explanation:
.
: The dot represents the current directory. Cppcheck will recursively analyze all files within this directory and its subdirectories.2> cppcheck.log
: Redirects all error messages to a file namedcppcheck.log
. The2>
indicates redirection of standard error (stderr) stream, which carries error messages.
Example Output:
While executing this command, progress messages will be displayed in the terminal window, showcasing which files are currently being analyzed. No errors will be displayed on the terminal as they will be logged in cppcheck.log
. Opening the log file might show entries like:
[./src/main.cpp:42]: (performance) Function call in loop.
[./include/utils.h:7]: (style) Class 'Helper' does not have a destructor.
Use case 2: Recursively check a given directory, and don’t print progress messages
Code:
cppcheck --quiet path/to/directory
Motivation:
In many situations, developers may find the continuous updates on the scan process distracting, especially during analysis of large codebases. To combat this, the --quiet
option allows them to suppress the progress messages, leading to a cleaner terminal output.
Explanation:
--quiet
: Suppresses the progress of the file checks. Useful for getting an uncluttered command line output.path/to/directory
: Specifies the targeted directory for the analysis. All subdirectories within this directory will also be checked.
Example Output: The output will be silent during execution. If issues are found, they will be briefly summarized without ongoing updates on the files currently being checked.
Use case 3: Check a given file, specifying which tests to perform
Code:
cppcheck --enable=error,warning,style path/to/file.cpp
Motivation: Given the diverse types of checks Cppcheck offers, there are times when developers need to focus on specific aspects of the code. For example, checking only for potential style issues, warnings, and errors can optimize the analysis time and efforts in focusing on immediate concerns.
Explanation:
--enable=error,warning,style
: This option specifies the categories of tests to run.error
searches for critical issues,warning
looks for potential risks, andstyle
highlights improvements in code aesthetics.path/to/file.cpp
: The path to the specific file being analyzed, allowing a targeted approach.
Example Output:
[main.cpp:25]: (style) Unread variable: 'flag'.
[main.cpp:47]: (warning) Possible division by zero.
Use case 4: List available tests
Code:
cppcheck --errorlist
Motivation: Understanding the available tests can profoundly inform developers about what they can diagnose with Cppcheck. This knowledge equips them to tailor their analysis to specific areas of concern.
Explanation:
--errorlist
: Produces a list of all possible checks that Cppcheck can perform, guiding developers in choosing the right checks for their code analysis.
Example Output: The output will be a comprehensive list:
error: Critical issues like syntax errors.
warning: Module specific warnings.
style: Issues like code style problems.
performance: Performance related issues.
portability: Code portability across platforms.
information: General information about the code.
all: Enables all checks.
Use case 5: Check a given file, ignoring specific tests
Code:
cppcheck --suppress=test_id1 --suppress=test_id2 path/to/file.cpp
Motivation: There are times when certain warnings or errors are irrelevant to a specific project context or have been acknowledged already. Suppressing these specific tests can lead to a more focused and meaningful analysis.
Explanation:
--suppress=test_id1 --suppress=test_id2
: Suppresses specified tests. Developers use this to skip known issues or concerns that are managed by other means.path/to/file.cpp
: Represents the file you wish to analyze while ignoring the suppressed tests.
Example Output:
If test_id1
pertains to style checks, these will not appear in the output:
[file.cpp:50]: (performance) Consider pre-allocating memory.
Use case 6: Check the current directory, providing paths for include files located outside it
Code:
cppcheck -I include/directory_1 -I include/directory_2 .
Motivation: Many projects include external libraries or headers that reside outside the main directory. Specifying the include paths ensures that Cppcheck can resolve all dependencies during analysis, resulting in accurate diagnostics.
Explanation:
-I include/directory_1 -I include/directory_2
: Provides additional include paths. Essential for projects with dependencies scattered across different directories..
: Designates the current directory for recursive checking.
Example Output: While running, Cppcheck would provide error messages considering the external includes:
[utils.cpp:30]: (error) Unresolved reference due to incorrect include path.
Use case 7: Check a Microsoft Visual Studio project (*.vcxproj
) or solution (*.sln
)
Code:
cppcheck --project path/to/project.sln
Motivation: Visual Studio projects typically have complex structures. This command automates the dependency handling by analyzing the project’s settings, making it simpler for developers involved in such environments to leverage Cppcheck’s functionalities without manual setup.
Explanation:
--project
: Specifies that an entire Visual Studio project or solution should be analyzed.path/to/project.sln
: Indicates the path to the solution file. Cppcheck will resolve all files and settings inside this solution.
Example Output: The result will cover multiple files involved in the solution:
[proj1/file1.cpp:75]: (portability) Code not portable due to specific OS usage.
[proj2/file2.cpp:22]: (information) Inconsistent use of spaces and tabs.
Conclusion
Cppcheck provides a sophisticated arsenal of tools for static code analysis, allowing developers to catch a broad spectrum of issues early in the development process. By understanding its capabilities and applying various use cases, developers can harness this tool to produce efficient, high-quality code. From recursive directory checks to tailoring analysis through selective enabling and suppression, Cppcheck’s adaptability ensures it can fit into any C/C++ developer’s workflow efficiently.