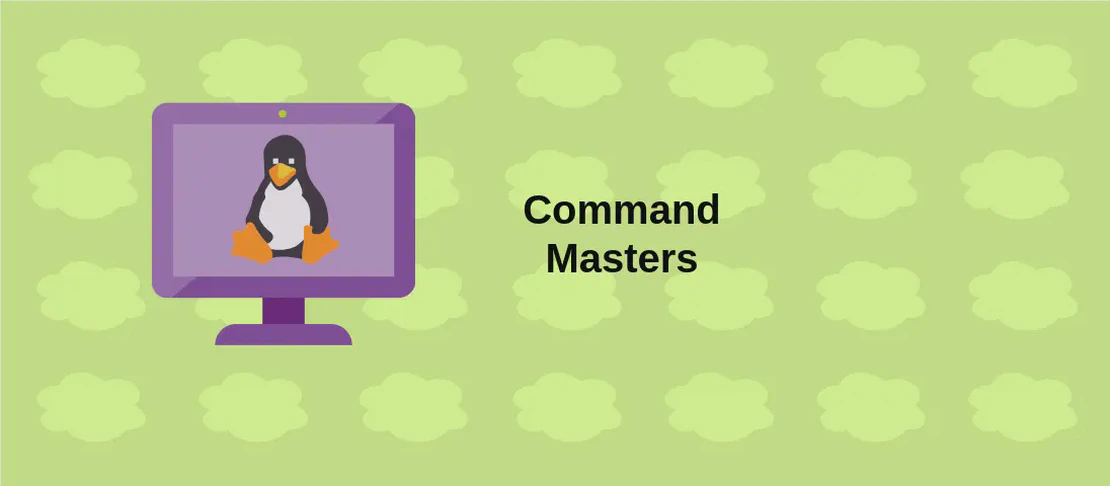
How to Use the 'crystal' Command (with Examples)
Crystal is a programming language that resembles Ruby in syntax but offers statically typed operations with the crucial advantage of high performance. It’s beneficial for developers who need the readability of Ruby but with better speed and efficiency for applications. The Crystal command-line interface serves as a tool for managing Crystal source code; it allows you to run scripts, compile files, generate documentation, manage specifications, and more. Below, we explore various use cases and their significance.
Use case 1: Run a Crystal file
Code:
crystal path/to/file.cr
Motivation: Running a Crystal file directly from the command line is useful during development and testing phases. By doing so, you can immediately observe the behavior of your code and make necessary adjustments rather than waiting for the compilation process to complete.
Explanation:
crystal
: This invokes the Crystal command-line interface, responsible for interpreting and processing the subsequent commands.path/to/file.cr
: The path indicating the Crystal file that you want to execute. This file (with a.cr
extension) contains the Crystal source code intended for execution.
Example Output:
Suppose the file hello_world.cr
contains puts "Hello, World!"
. Running the command outputs:
Hello, World!
Use case 2: Compile a file and all dependencies to a single executable
Code:
crystal build path/to/file.cr
Motivation: Compiling Crystal source code into a standalone executable improves performance by eliminating interpretation overhead. This is particularly advantageous for deployment, where execution speed is critical and resources are managed efficiently.
Explanation:
crystal
: Starts the Crystal interface.build
: The command specifically for compiling a Crystal file into an executable.path/to/file.cr
: The file path to the Crystal source code being compiled.
Example Output:
Commencing with the hello_world.cr
file, a new executable hello_world
is created in the working directory.
Use case 3: Read Crystal source code from the command-line or stdin
, and execute it
Code:
crystal eval 'code'
Motivation: This use case is advantageous when you want to test small snippets of Crystal code without creating a new file. It can also facilitate quick experimentation in environments where scripting and rapid iteration are key.
Explanation:
crystal
: Activates the Crystal command interface.eval
: Signifies the execution of code provided directly in quotes or throughstdin
.'code'
: A string containing the Crystal code you wish to evaluate.
Example Output:
Executing crystal eval 'puts "Hello from eval!"'
generates:
Hello from eval!
Use case 4: Generate API documentation from inline docstrings in Crystal files
Code:
crystal docs
Motivation: Generating comprehensive API documentation is crucial for code maintenance and collaboration in professional environments. This ensures that future developers, or even yourself at a later date, can understand the functions and structure of your code quickly without having to delve into the source.
Explanation:
crystal
: Activates the Crystal command interface.docs
: Directs the Crystal interface to create documentation from the inline comments and docstrings.
Example Output:
A folder, often ./docs
, is created containing HTML files that illustrate the API documentation for your project.
Use case 5: Compile and run a Crystal specification suite
Code:
crystal spec
Motivation: Running specification tests is integral to validating the functionality of code and ensuring it behaves as expected. By using this command, developers can catch bugs early in the development process, saving time and resources.
Explanation:
crystal
: Initiates the Crystal interface.spec
: This command compiles and executes test cases defined within the Crystal project’sspec
directory.
Example Output: Executing tests results in output reflecting successful or failed test cases, akin to:
....
Finished in 0.0024 seconds
4 examples, 0 failures
Use case 6: Start a local interactive server for testing the language
Code:
crystal play
Motivation:
An interactive server launched using crystal play
is helpful for live coding sessions, tutorials, or even interactive experiments with the language. This allows you to see real-time results and foster a better understanding of code behavior.
Explanation:
crystal
: Initiates the Crystal command interface.play
: Launches a web-based IDE environment where developers can write and test Crystal code.
Example Output:
The command starts a server, accessible through a web browser, bearing an address like http://localhost:8080
.
Use case 7: Create a project directory for a Crystal application
Code:
crystal init app application_name
Motivation: Starting a new project can often involve repetitive setup tasks. Automating this process with the command simplifies it by generating a standardized project structure, allowing developers to focus on writing functional code from the start.
Explanation:
crystal
: This is the command to launch the Crystal interface.init
: Indicates project initialization.app
: Specifies that the project involves an application.application_name
: Replace with your desired project name for directory creation.
Example Output:
The command establishes a new directory named after the application with necessary subdirectories and files for a Crystal app, such as src
, spec
, shard.yml
, etc.
Use case 8: Display all help options
Code:
crystal help
Motivation: Understanding all available commands and options can greatly improve a developer’s efficiency with Crystal. It is an essential resource for both new and seasoned Crystal developers to become more adept with various functionalities.
Explanation:
crystal
: Engages the Crystal command interface.help
: Command to display a comprehensive list of all commands, options, and administrative details for using the Crystal language and its interface.
Example Output: Displays helpful information about the crystal tool and subcommands:
Usage: crystal [command] [options]
Commands:
- build Build a Crystal source file.
- docs Generate documentation.
- eval Eval code from command line.
- ...
...
Conclusion:
The ‘crystal’ command offers a rich suite of capabilities that empower developers to efficiently manage Crystal projects, ranging from basic execution and compilation to more advanced operations like documentation and interactive testing. Understanding and utilizing these commands streamline your workflow and enhance your productivity when working with the Crystal programming language.