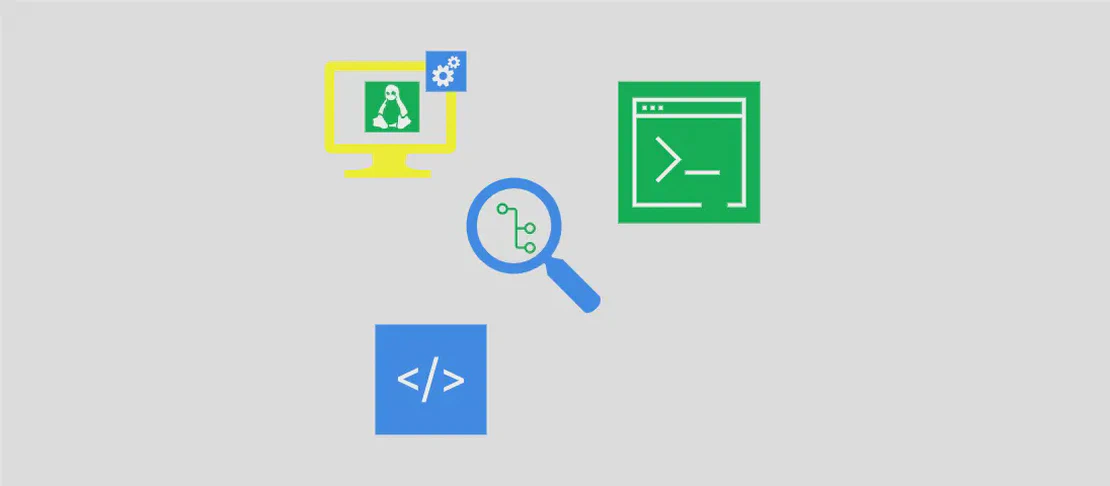
Understanding the 'cs fetch' Command (with examples)
The cs fetch
command is a utility from the Coursier suite of tools, primarily used to download JAR files for specified dependencies. It streamlines the process of fetching Java library dependencies, making it a valuable tool for developers working with the Java ecosystem. This article explores various use cases of the cs fetch
command, demonstrating its versatility through illustrative examples.
Use case 1: Fetch a specific version of a jar
Code:
cs fetch group_id:artifact_id:artifact_version
Motivation:
A common need in software development is to fetch a specific version of a dependency. This is particularly useful when starting a new project or maintaining compatibility with a specific library version. Fetching a particular version ensures that your application behaves consistently, as library updates can sometimes introduce breaking changes.
Explanation:
group_id
: Represents the group that the library belongs to, usually in reverse domain name notation (e.g.,org.apache
).artifact_id
: The name of the library/artifact you want to fetch.artifact_version
: Specifies the exact version of the library to download.
Example Output:
Downloaded artifact: /path/to/local/cache/group_id/artifact_id/artifact_version/artifact_id-artifact_version.jar
Use case 2: Fetch a package and evaluate the classpath corresponding to the selected package in an env var
Code:
CP="$(cs fetch --classpath org.scalameta::scalafmt-cli:latest.release)"
Motivation:
Managing dependencies and classpaths is a critical aspect of Java development. This use case illustrates how to set an environment variable with the classpath for a package. It is particularly useful when configuring build tools or running Java applications that require specific dependencies.
Explanation:
--classpath
: A flag that fetches the classpath instead of just the JAR.org.scalameta::scalafmt-cli:latest.release
: Indicates using the latest available release ofscalafmt-cli
fromorg.scalameta
.
Example Output:
/path/to/cached/jars/org/scalameta/scalafmt-cli.jar:/path/to/dependency/jars
Use case 3: Fetch a source of a specific jar
Code:
cs fetch --sources group_id:artifact_id:artifact_version
Motivation:
Fetching the source code of a JAR is invaluable for debugging and understanding how a library works. This use case is beneficial for developers who need access to the internal workings of a library and want to explore or modify it directly.
Explanation:
--sources
: This option fetches the source code associated with the specified JAR version.
Example Output:
Downloaded sources: /path/to/local/cache/group_id/artifact_id/artifact_version/artifact_id-artifact_version-sources.jar
Use case 4: Fetch the javadoc jars
Code:
cs fetch --javadoc group_id:artifact_id:artifact_version
Motivation:
Java developers often rely on comprehensive documentation to understand and effectively use libraries. This use case demonstrates how to fetch the Javadoc for a specified library, providing access to its API documentation offline.
Explanation:
--javadoc
: Fetches the Javadoc associated with the specified JAR version.
Example Output:
Downloaded javadoc: /path/to/local/cache/group_id/artifact_id/artifact_version/artifact_id-artifact_version-javadoc.jar
Use case 5: Fetch dependency with javadoc jars and source jars
Code:
cs fetch --default=true --sources --javadoc group_id:artifact_id:artifact_version
Motivation:
For a comprehensive understanding of a library, obtaining both its compiled code, source code, and documentation is often necessary. This use case elaborates on fetching everything required for full insight and development convenience concerning a library.
Explanation:
--default=true
: Ensures the default behavior of including the binary JAR.--sources
: Fetches the source JAR.--javadoc
: Fetches the Javadoc JAR.
Example Output:
Downloaded artifact: /path/to/local/cache/artifact.jar
Downloaded sources: /path/to/local/cache/sources.jar
Downloaded javadoc: /path/to/local/cache/javadoc.jar
Use case 6: Fetch jars coming from dependency files
Code:
cs fetch --dependency-file path/to/file1 --dependency-file path/to/file2 ...
Motivation:
Managing multiple dependencies can become complex, especially in large projects. This use case shows how to fetch JARs listed in dependency files, simplifying the process by maintaining lists that are easy to update and manage. It enhances the maintainability of project configurations.
Explanation:
--dependency-file
: Specifies the path to files that list dependencies. Each file can contain multiple dependencies ingroup_id:artifact_id:artifact_version
format.
Example Output:
Downloaded from file1: /path/to/local/cache/dependency1.jar
Downloaded from file2: /path/to/local/cache/dependency2.jar
Conclusion:
The cs fetch
command is a versatile and powerful tool within the Coursier suite, simplifying the management of Java dependencies by providing a range of options to fetch binaries, sources, and documentation. Whether you’re setting up a new project, maintaining existing ones, or require in-depth insights into library code, the examples above illustrate how cs fetch
can meet a wide array of developer needs.