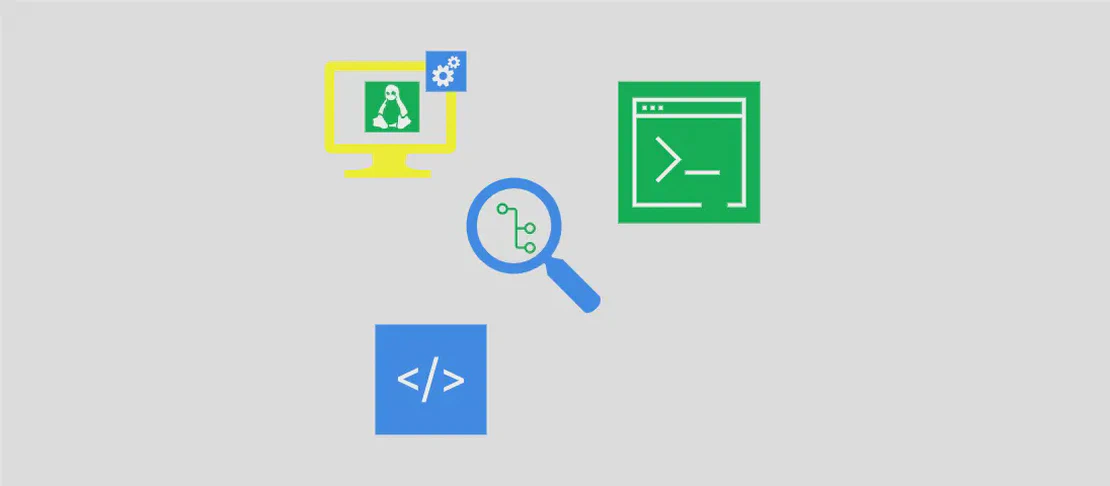
How to Use the Command 'cs resolve' (with Examples)
The cs resolve
command is a powerful tool provided by Coursier, which is primarily used in Scala and Java dependency management. It helps developers and engineers resolve, analyze, and troubleshoot dependencies of software projects. This tool is particularly essential in managing transitive dependencies—that is, dependencies that are themselves dependencies of other components—and provides insights into the structure and potential issues within a project’s dependency graph.
Use Case 1: Resolve Lists of Transitive Dependencies of Two Dependencies
Code:
cs resolve group_id1:artifact_id1:artifact_version1 group_id2:artifact_id2:artifact_version2
Motivation:
In any sizable project, managing dependencies can get complex. Imagine you are working on a project where two major libraries or frameworks are used, and you want to grasp which transitive dependencies are involved. Resolving the transitive dependencies for these two components can help you ensure that there are no conflicts or missed updates in your dependency management.
Explanation:
group_id1:artifact_id1:artifact_version1
: This represents the first dependency, wheregroup_id1
is the identifier for the organization or group that publishes the artifact,artifact_id1
is the specific library or project name, andartifact_version1
is the version.group_id2:artifact_id2:artifact_version2
: This signifies the second dependency in the same format as the first.
Example Output:
When you run this command, you might see a list of dependencies, showing you the full tree of what each of the specified dependencies relies on. This can also include version numbers and other relevant metadata.
Use Case 2: Resolve Lists of Transitive Dependencies of a Package by the Dependency Tree
Code:
cs resolve --tree group_id:artifact_id:artifact_version
Motivation:
This use case becomes essential when you want a hierarchical view of how a particular dependency is structured, including all its transitive dependencies. This tree view helps developers understand the entire dependency graph and spot redundant or conflicting dependencies.
Explanation:
--tree
: This flag instructs the command to display the dependencies in a tree-like structure, making it easier to visualize parent-child relationships between the dependencies.group_id:artifact_id:artifact_version
: Specifies the dependency you want to analyze.
Example Output:
The output will be a tree structure, displaying the root dependency at the top and its transitive dependencies indented below it. This structured view provides clarity on how different libraries and tools are interconnected.
Use Case 3: Resolve Dependency Tree in Reverse Order (From a Dependency to its Dependencies)
Code:
cs resolve --reverse-tree group_id:artifact_id:artifact_version
Motivation:
This scenario is useful when you need to track back from a specific module or package to its direct dependencies. It can be beneficial for debugging purposes when particular final dependencies are known to cause issues, and you want to trace their origins.
Explanation:
--reverse-tree
: This option flips the dependency tree, allowing you to see dependencies from the bottom up.group_id:artifact_id:artifact_version
: Determines the artifact in question.
Example Output:
In the output, dependencies that lead up to the designated artifact are listed, which can help you trace specific paths in your dependency graph.
Use Case 4: Print All the Libraries that Depend on a Specific Library
Code:
cs resolve group_id:artifact_id:artifact_version --what-depends-on searched_group_id:searched_artifact_id
Motivation:
This application is beneficial when a particular library needs updating, or if a deprecation notice is issued, and you want to know every place it is used directly or indirectly. It helps in planning modifications.
Explanation:
group_id:artifact_id:artifact_version
: Represents the target dependencies.--what-depends-on
: A flag to focus on what other libraries depend on the specified artifact.searched_group_id:searched_artifact_id
: The specific library you are querying about.
Example Output:
The output will list all the dependencies which rely on the searched artifact, providing you with an effective inventory of dependencies.
Use Case 5: Print All the Libraries that Depend on a Specific Library Version
Code:
cs resolve group_id:artifact_id:artifact_version --what-depends-on searched_group_id:searched_artifact_idsearched_artifact_version
Motivation:
This allows for even more granular control, specifying not just a library but a particular version of it. This can be indispensable when a specific version has a vulnerability or bug and requires updating across your entire project.
Explanation:
--what-depends-on
: Focuses the query on dependencies related to the specified version of the artifact.searched_group_id:searched_artifact_idsearched_artifact_version
: Details the exact version of the library being examined.
Example Output:
You’ll receive a list showing all dependencies that explicitly rely on that specific library version, which is crucial for precise dependency management.
Use Case 6: Print Eventual Conflicts Between a Set of Packages
Code:
cs resolve --conflicts group_id1:artifact_id1:artifact_version1 group_id2:artifact_id2:artifact_version2 ...
Motivation:
Dependencies can sometimes have clashes, especially when multiple versions of a library are included inadvertently. This command identifies such conflicts, allowing developers to address compatibility or redundancy issues effectively.
Explanation:
--conflicts
: This option triggers a check for conflicts between the specified packages.- A list of
group_id:artifact_id:artifact_version
: The collection of dependencies among which you want to detect potential conflicts.
Example Output:
The command produces a list of conflicts, detailing which components are incompatible and possibly making suggestions on how to resolve these issues.
Conclusion:
The cs resolve
command is a multifaceted tool that provides detailed insights into dependencies and their management within a project. By leveraging its capabilities to resolve transitive dependencies, analyze dependency trees, and detect conflicts or dependencies on particular libraries or versions, developers can maintain robust and error-free software architectures.