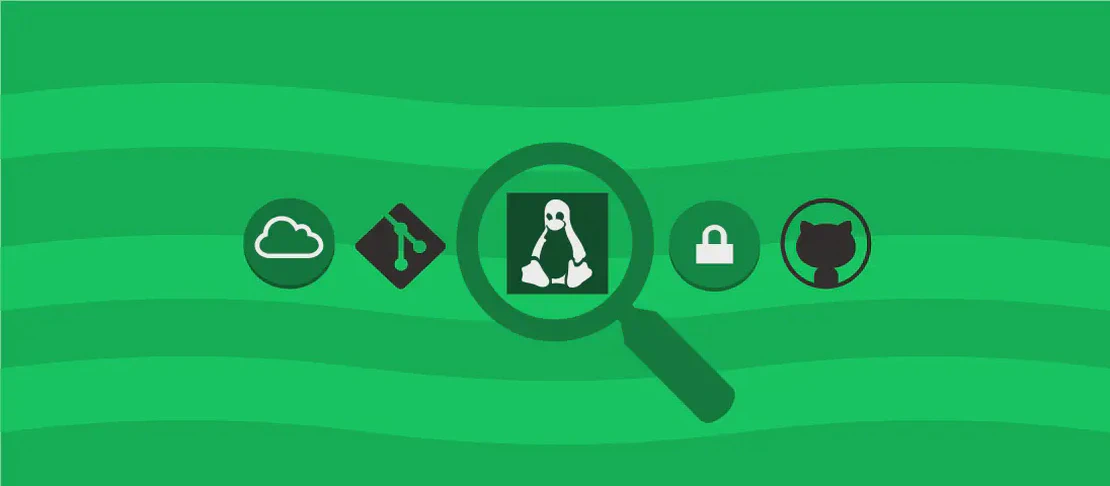
How to Use the Command 'csc' (with Examples)
The ‘csc’ command stands for the Microsoft C# Compiler, which is a tool used to compile C# source files into Common Intermediate Language (CIL) executables or libraries. This powerful command-line utility allows developers to build applications and libraries directly from one or more C# files, offering a plethora of options to customize the compilation process, such as specifying output names, embedding resources, or produced executable types. The flexibility of ‘csc’ makes it an essential tool for C# developers who wish to maintain control over their build processes.
Use case 1: Compile One or More C# Files to a CIL Executable
Code:
csc path/to/input_file_a.cs path/to/input_file_b.cs
Motivation:
When creating a C# application, a developer often writes code across multiple files. Compiling several C# files into a single executable is a common task that developers perform to bring together all the functionality their application encompasses. This approach is not only efficient but also necessary for ensuring seamless interactions between different parts of the application.
Explanation:
csc
: This invokes the C# compiler.path/to/input_file_a.cs
andpath/to/input_file_b.cs
: These paths point to the C# source files that need to be compiled. Each file contains classes and methods that are part of the application.
Example Output:
Producing a .exe file named after the first C# file provided (e.g., input_file_a.exe
). This executable can be run directly to see the application in action.
Use case 2: Specify the Output Filename
Code:
csc /out:path/to/filename path/to/input_file.cs
Motivation:
Specifying the output filename provides the developer with control over how their compiled application is named, especially useful in larger projects where multiple builds are performed or where naming conventions need to be adhered to for versioning or distribution purposes.
Explanation:
csc
: The command to start the C# compiler./out:path/to/filename
: This flag allows the user to specify a custom name and location for the compiled executable or library. It overrides the default naming process.path/to/input_file.cs
: The path to the C# source file being compiled.
Example Output:
Creates an output executable or library with the specified filename, making it easier to manage and identify among numerous builds.
Use case 3: Compile into a .dll
Library Instead of an Executable
Code:
csc /target:library path/to/input_file.cs
Motivation:
Compiling code into a .dll
(Dynamic Link Library) is crucial when building reusable components or libraries, which can be consumed by multiple applications. This is particularly beneficial in a modular architecture or when creating plugins.
Explanation:
csc
: Initiates the C# compilation process./target:library
: Instructs the compiler to output a library (DLL) file rather than an executable.path/to/input_file.cs
: The path indicating which source file to compile into a library.
Example Output:
Generates a .dll
file instead of an .exe
, allowing other applications to reference this library and use its contained functionality.
Use case 4: Reference Another Assembly
Code:
csc /reference:path/to/library.dll path/to/input_file.cs
Motivation:
Referencing other assemblies is essential when working with dependencies or when your application needs to leverage external libraries and frameworks. It ensures that the compiled application has all necessary resources, enabling it to function as intended.
Explanation:
csc
: The compiler for C# files./reference:path/to/library.dll
: This argument tells the compiler to include the specified assembly, making its types and methods available to the compiling application.path/to/input_file.cs
: The path to the primary source file for the compilation.
Example Output:
Produces an executable or library file that has access to the referenced assembly’s resources, enabling the application to utilize third-party or additional functionality.
Use case 5: Embed a Resource
Code:
csc /resource:path/to/resource_file path/to/input_file.cs
Motivation:
Embedding resources such as images, files, or data directly into an assembly is a prompt way to ensure your application carries all required dependencies internally. It’s particularly helpful to avoid external file handling issues and enhance portability.
Explanation:
csc
: The C# compiler command./resource:path/to/resource_file
: This includes the specified file as an embedded resource within the compiled output.path/to/input_file.cs
: The path to the C# source file being compiled.
Example Output:
Produces an application that includes and can access the embedded resource internally, without needing to rely on external files.
Use case 6: Automatically Generate XML Documentation
Code:
csc /doc:path/to/output.xml path/to/input_file.cs
Motivation:
Automating XML documentation generation is significant for maintaining clear, up-to-date documentation that can be used for code reference, sharing with teams, or generating API documentation. It ensures that documentation is consistently aligned with the codebase.
Explanation:
csc
: Calls the C# compiler./doc:path/to/output.xml
: Directs the compiler to produce XML documentation based on the comments in the code, outputting to the specified file.path/to/input_file.cs
: This is the path where the source file exists.
Example Output:
Creates an XML file containing documentation comments extracted from the source code, aiding in the creation of developer guides or API docs.
Use case 7: Specify an Icon
Code:
csc /win32icon:path/to/icon.ico path/to/input_file.cs
Motivation:
Specifying an icon for an application is essential for branding and user recognition, particularly in GUI applications where visual presentation matters to provide a polished and professional appearance.
Explanation:
csc
: The command to execute the C# compiler./win32icon:path/to/icon.ico
: This option assigns an ICO file as the application’s icon.path/to/input_file.cs
: The path to the source code file being compiled.
Example Output:
Produces an executable with the specified icon, which appears in the operating system’s file explorer and when the application is running, enhancing brand coherence.
Use case 8: Strongly-Name the Resulting Assembly with a Keyfile
Code:
csc /keyfile:path/to/keyfile path/to/input_file.cs
Motivation:
Using a strong name to sign assemblies is critical for security and version management, particularly in enterprise environments. It guarantees the assembly’s authenticity and integrity, preventing unauthorized modifications.
Explanation:
csc
: This is the C# compiler invocation./keyfile:path/to/keyfile
: Directs the compiler to use a specific key file to sign the assembly, providing a strong name.path/to/input_file.cs
: The path to the C# file being compiled.
Example Output:
Generates a strongly-named assembly, designated for secure deployment and version control, ensuring that it can coexist with other assemblies without conflicts.
Conclusion:
The csc
command is a versatile tool within the .NET ecosystem, offering various features that support the development and deployment of robust C# applications. From specifying output types and embedding resources to ensuring secure assembly versioning, the csc
command empowers developers with fine-grained control over the compilation process. Embracing these features not only enhances the productivity and agility of software development but also ensures that applications can be created with precision and reliability.