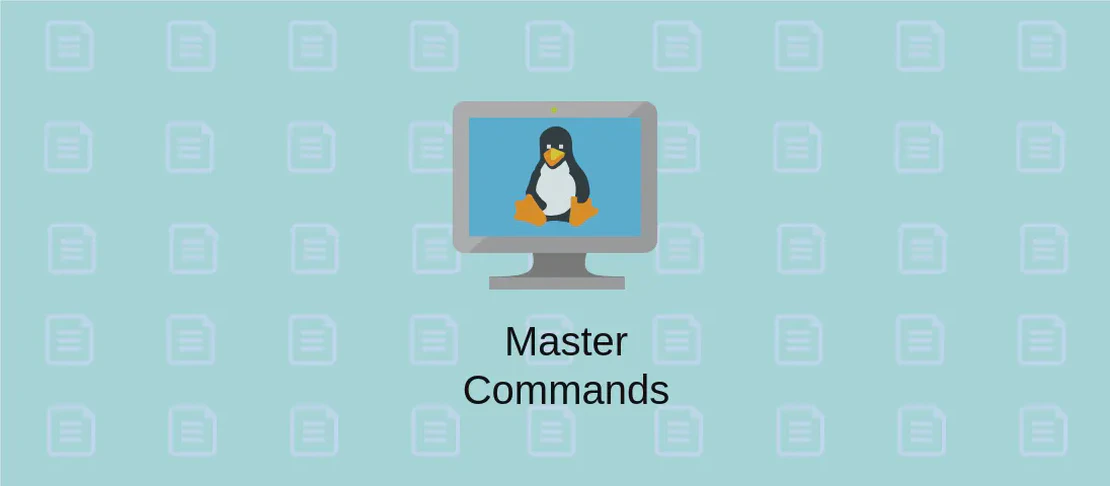
Mastering the Dart Command Line Tool (with examples)
Dart is a programming language designed for building web, server, and mobile applications. The Dart command line tool offers powerful options for managing Dart projects, compiling code, managing dependencies, and more. This tool is part of the Dart SDK and provides various commands to facilitate the development process.
Use case 1: Initialize a new Dart project in a directory of the same name
Code:
dart create project_name
Motivation:
Starting a new project can often be an intimidating task, especially when dealing with directory setup and project configuration. The dart create
command automates this process by setting up a new Dart project in a directory named after your project. This command saves time and ensures that your project is configured correctly with standard files and structure.
Explanation:
dart
: This is the Dart command line tool invoked from your terminal or command prompt.create
: This subcommand is used to initiate a new Dart project.project_name
: This argument specifies the name you desire for your new project. It also becomes the directory name where the project files will be stored.
Example output:
Creating project_name using template console-simple...
project_name/.gitignore
project_name/CHANGELOG.md
project_name/pubspec.yaml
project_name/bin/project_name.dart
Running pub get to resolve dependencies...
Success!
Use case 2: Run a Dart file
Code:
dart run path/to/file.dart
Motivation:
During development, frequently running your code to test out new changes or debug specific sections is essential. The dart run
command simplifies this process by executing a Dart file directly, without the need for additional compilation steps. This quick feedback loop is critical in identifying issues and validating functionality as you write code.
Explanation:
dart
: The Dart command line tool.run
: The subcommand used to execute Dart script files.path/to/file.dart
: The path specifies the Dart file you wish to run. Ensure the file path is correct relative to your current directory.
Example output:
Hello, World!
Use case 3: Download dependencies for the current project
Code:
dart pub get
Motivation:
Modern software projects often rely on numerous external libraries and packages to accelerate development and add functionalities. The dart pub get
command is essential when setting up a project or after adding new dependencies to the pubspec.yaml
file. This command fetches the listed packages, ensuring they are downloaded and made available for your project.
Explanation:
dart
: Invokes the Dart tool.pub
: Calls the Dart package manager, similar tonpm
for Node orpip
for Python.get
: Instructs the package manager to fetch the dependencies specified in your project’spubspec.yaml
file.
Example output:
Resolving dependencies...
+ analyzer 2.1.0
+ async 2.8.1
+ collection 1.16.0
+ source_span 1.8.1
Downloading dependencies...
Downloading analyzer 2.1.0...
Downloading async 2.8.1...
Use case 4: Run unit tests for the current project
Code:
dart test
Motivation:
Testing is a vital aspect of software development as it ensures your application functions correctly and remains resilient over time. The dart test
command provides a straightforward way to execute all unit tests in your Dart project. This command helps developers maintain code quality and identify breaking changes throughout the development cycle.
Explanation:
dart
: The Dart command line tool.test
: A subcommand that runs all the test files, usually located in thetest
directory of your Dart project.
Example output:
00:01 +5: All tests passed!
Use case 5: Update an outdated project’s dependencies to support null-safety
Code:
dart pub upgrade --null-safety
Motivation:
Null-safety is a major feature in Dart that helps avoid null reference errors at runtime. Upgrading your project’s dependencies to adhere to null-safety is crucial for optimizing code quality and ensuring safer, more reliable applications. The dart pub upgrade --null-safety
command updates dependencies and enables null safety in one go, allowing developers to modernize their codebases.
Explanation:
dart
: The Dart command line tool.pub
: Refers to the Dart package manager.upgrade
: Tells the package manager to update dependencies to the latest versions.--null-safety
: This flag specifies that the command should attempt to migrate dependencies to versions compatible with Dart’s null-safety feature.
Example output:
Resolving dependencies...
Upgraded to null-safety compatible dependencies.
Use case 6: Compile a Dart file to a native binary
Code:
dart compile exe path/to/file.dart
Motivation:
Compiling Dart scripts to native binary executables is advantageous when you want to improve execution speed, distribute your application without requiring the Dart runtime, or deploy scripts as standalone tools. The dart compile exe
command facilitates this by producing a self-sufficient binary from your Dart code.
Explanation:
dart
: The Dart command line tool.compile
: The command to begin the compilation process.exe
: Indicates the output format should be a native executable.path/to/file.dart
: The path to the Dart file you wish to compile.
Example output:
Compiling path/to/file.dart to path/to/file.exe...
Generated: path/to/file.exe
Use case 7: Apply automated fixes to the current project
Code:
dart fix --apply
Motivation:
Keeping your code clean and up to date with the latest syntactic and stylistic best practices can be daunting. Automatic fixes can save developers time by programmatically making recommended changes. The dart fix --apply
command leverages built-in and community-provided rules to apply these fixes, improving code quality and consistency.
Explanation:
dart
: The Dart command line tool.fix
: Invokes the tool to identify potential fixes in your codebase.--apply
: Directs the tool to automatically apply all of the recommended changes.
Example output:
Analyzing project...
Applying fixes in lib/...
Fixes applied successfully!
Conclusion:
The Dart command line tool is an essential asset for Dart developers, simplifying numerous tasks throughout the development lifecycle. Whether you’re initializing a project, testing, or managing dependencies, this tool offers robust commands to streamline and enhance your workflow. Understanding these commands, as well as their specific arguments and outputs, paves the way for efficient and effective software development in the Dart ecosystem.