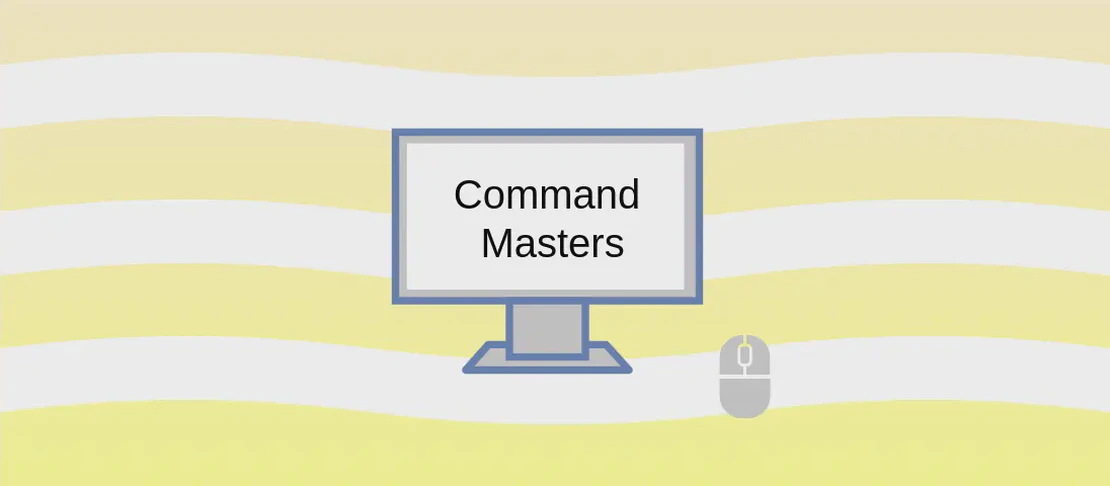
How to use the command 'declare' (with examples)
The ‘declare’ command in Bash is used to declare variables and assign them attributes. It enables you to specify the type of variable, such as string, integer, array, or associative array. This provides flexibility and control over how the variables are treated and used within your Bash scripts.
Use case 1: Declare a string variable with the specified value
Code:
declare variable="value"
Motivation: Declaring a string variable with the specified value allows you to store and manipulate text-based data. This can be useful for tasks such as storing user input, displaying messages, or working with file paths.
Explanation:
- ‘declare’ is the command keyword used to declare a variable.
- ‘variable’ is the name of the variable you want to declare.
- ‘=’ is the assignment operator used to assign a value to the variable.
- ‘“value”’ is the specified value you want to assign to the variable. It can be any string of characters.
Example output:
echo $variable
Output: value
Use case 2: Declare an integer variable with the specified value
Code:
declare -i variable="value"
Motivation: Declaring an integer variable with the specified value allows you to perform mathematical operations and comparisons on numerical data. This is useful for tasks such as counting, tracking progress, or implementing conditional logic.
Explanation:
- ‘declare’ is the command keyword used to declare a variable.
- ‘-i’ is an attribute option that specifies the variable as an integer.
- ‘variable’ is the name of the variable you want to declare.
- ‘=’ is the assignment operator used to assign a value to the variable.
- ‘“value”’ is the specified value you want to assign to the variable. It should be a valid integer.
Example output:
echo $variable
Output: value (as an integer, not as a string)
Use case 3: Declare an array variable with the specified value
Code:
declare -a variable=(item_a item_b item_c)
Motivation: Declaring an array variable allows you to store multiple values in a single variable. This is useful when you want to work with a collection of related data, such as a list of filenames, command-line arguments, or options.
Explanation:
- ‘declare’ is the command keyword used to declare a variable.
- ‘-a’ is an attribute option that specifies the variable as an array.
- ‘variable’ is the name of the variable you want to declare.
- ‘=’ is the assignment operator used to assign a value to the variable.
- ‘(item_a item_b item_c)’ is the specified value you want to assign to the array variable. It contains a list of items enclosed in parentheses.
Example output:
echo ${variable[0]}
Output: item_a
Use case 4: Declare an associative array variable with the specified value
Code:
declare -A variable=([key_a]=item_a [key_b]=item_b [key_c]=item_c)
Motivation: Declaring an associative array variable allows you to store and retrieve values using key-value pairs. This is useful when you want to associate related data, such as a mapping between names and ages, or between identifiers and corresponding values.
Explanation:
- ‘declare’ is the command keyword used to declare a variable.
- ‘-A’ is an attribute option that specifies the variable as an associative array.
- ‘variable’ is the name of the variable you want to declare.
- ‘=’ is the assignment operator used to assign a value to the variable.
- ‘[key_a]=item_a [key_b]=item_b [key_c]=item_c’ is the specified value you want to assign to the associative array variable. It consists of key-value pairs enclosed in square brackets.
Example output:
echo ${variable[key_b]}
Output: item_b
Use case 5: Declare a readonly string variable with the specified value
Code:
declare -r variable="value"
Motivation: Declaring a readonly string variable ensures that its value cannot be changed throughout the execution of your script. This is useful when you have a constant value that should not be modified or accidentally reassigned.
Explanation:
- ‘declare’ is the command keyword used to declare a variable.
- ‘-r’ is an attribute option that specifies the variable as readonly.
- ‘variable’ is the name of the variable you want to declare.
- ‘=’ is the assignment operator used to assign a value to the variable.
- ‘“value”’ is the specified value you want to assign to the variable. It can be any string of characters.
Example output:
variable="new_value"
Output: Cannot assign new value to readonly variable
Use case 6: Declare a global variable within a function with the specified value
Code:
declare -g variable="value"
Motivation: Declaring a global variable within a function allows you to share and access the variable across multiple functions or scripts. Normally, variables declared within a function are limited to that function’s scope, but with the ‘-g’ option, you can make it accessible from outside the function.
Explanation:
- ‘declare’ is the command keyword used to declare a variable.
- ‘-g’ is an attribute option that specifies the variable as global.
- ‘variable’ is the name of the variable you want to declare.
- ‘=’ is the assignment operator used to assign a value to the variable.
- ‘“value”’ is the specified value you want to assign to the variable. It can be any string of characters.
Example output:
echo $variable
Output: value
Conclusion:
The ‘declare’ command in Bash provides a powerful way to declare and assign attributes to variables. Whether you need to store text, numbers, arrays, or key-value pairs, the ‘declare’ command allows you to define variables with the desired properties. Understanding the different use cases and attributes associated with the ‘declare’ command can enhance your Bash scripting capabilities and improve code clarity and efficiency.