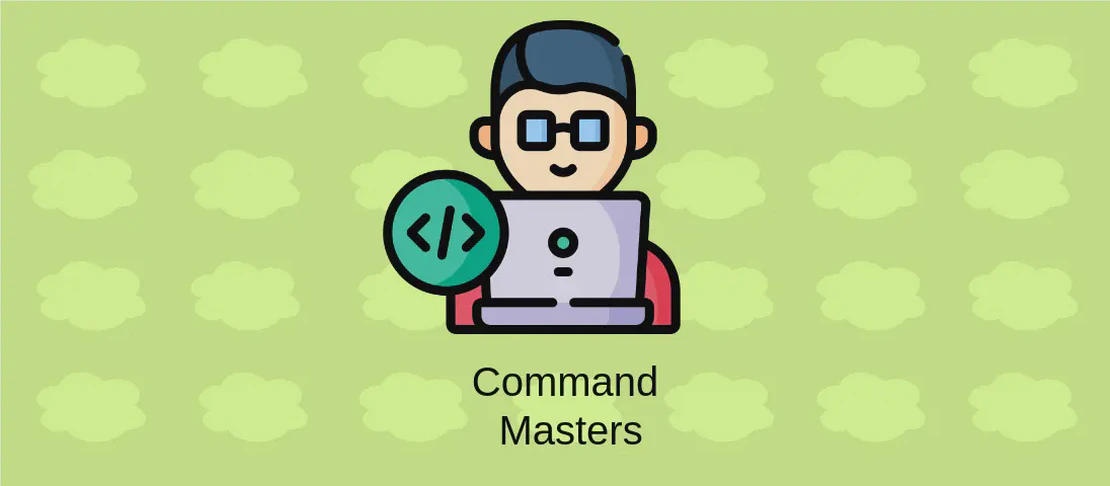
deno (with examples)
1: Running a JavaScript or TypeScript file
Code:
deno run path/to/file.ts
Motivation: This command is used to execute a JavaScript or TypeScript file in the Deno runtime environment. It allows developers to run their code without the need for a separate runtime or browser.
Explanation:
deno run
: This is the command that tells Deno to execute a script file.path/to/file.ts
: This argument specifies the path to the JavaScript or TypeScript file you want to run. Make sure to provide the correct file path relative to the current working directory.
Example output: If the file path/to/file.ts
contains the code console.log("Hello, Deno!");
, running the above command will output Hello, Deno!
.
2: Starting a REPL (interactive shell)
Code:
deno
Motivation: The Deno REPL, or the interactive shell, is useful for experimenting with JavaScript or TypeScript code in an interactive and immediate manner. It allows developers to quickly test small code snippets without the need to create or run a separate file.
Explanation: Running the deno
command without any arguments starts the Deno REPL. It provides a prompt where you can directly enter and execute JavaScript or TypeScript code.
Example output: When the Deno REPL starts, it presents a prompt >
. Here, you can enter code directly and see the output immediately. For example:
> console.log("Hello, Deno!");
Hello, Deno!
3: Running a file with network access enabled
Code:
deno run --allow-net path/to/file.ts
Motivation: Deno provides a security sandbox that restricts network access by default. However, in some cases, you may need to make network requests from your code. This command allows you to explicitly enable network access for the script being executed.
Explanation:
deno run
: This is the command that tells Deno to execute a script file.--allow-net
: This argument explicitly enables network access for the script.path/to/file.ts
: This argument specifies the path to the JavaScript or TypeScript file you want to run. Make sure to provide the correct file path relative to the current working directory.
Example output: If the file path/to/file.ts
contains code that makes a network request, running the above command will successfully execute the script and retrieve data from the network.
4: Running a file from a URL
Code:
deno run https://deno.land/std/examples/welcome.ts
Motivation: Deno allows you to run script files directly from a URL. This feature is helpful when you want to quickly test or execute scripts without having to download and save them locally.
Explanation: The deno run
command accepts a URL as an argument, indicating that the script should be fetched and executed from the provided URL.
Example output: Running the above command will fetch the script file welcome.ts
from the URL https://deno.land/std/examples/welcome.ts
and execute it. The script may generate specific output depending on its implementation.
5: Installing an executable script from a URL
Code:
deno install https://deno.land/std/examples/colors.ts
Motivation: This command allows you to install scripts as executables, making them accessible from the command line. Installing scripts can be useful when you regularly use certain Deno scripts and want to run them as standalone commands.
Explanation: The deno install
command followed by a URL installs the script as an executable. Once installed, you can run the script by typing its name directly in the command line.
Example output: After running the above command, the script colors.ts
will be installed as an executable. You can then execute it by simply typing colors
in the command line. The specific output will depend on the implementation of the colors.ts
script.