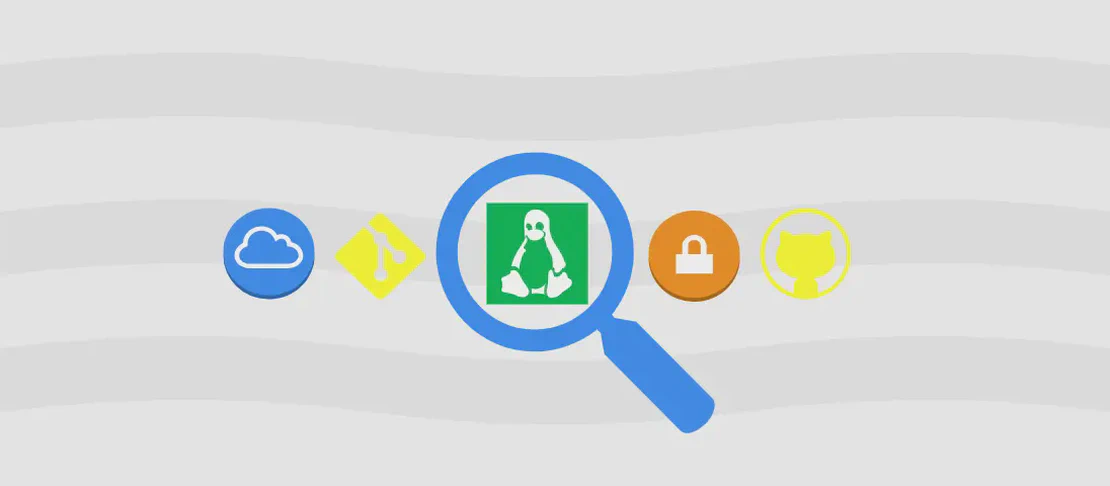
Devcontainer Command in Docker: A Comprehensive Guide (with examples)
The devcontainer
command is a versatile tool designed to use Docker containers as development environments. It simplifies setting up and maintaining consistent and reproducible development setups, leveraging containerization to ensure that the environment remains uniform across different machines and setups. This command is particularly popular among developers who work in collaborative environments or who need to manage multiple projects that require separate dependencies and configurations.
Use case 1: Create and run a Dev Container
Code:
devcontainer up
Motivation:
In many software development scenarios, the challenge is ensuring that a development environment matches a production environment to catch potential issues early. By running devcontainer up
, developers can seamlessly initiate and configure their development environment, mirroring production exactly and eliminating “it works on my machine” issues. This command helps automate the setup process, reducing manual steps, boosting productivity, and ensuring environment consistency.
Explanation:
The command devcontainer up
is concise and focused on one primary action - setting up and running a containerized development environment based on existing configurations. It leverages a predefined devcontainer.json
file to pull together the necessary Docker images and run them. This abstracts away many of the typically manual steps like setting environment variables, installing dependencies, and starting up servers.
Example Output:
- Initializing configuration…
- Pulling Docker images…
- Starting the container environment…
- Dev Container is up and running at localhost:8000
Use case 2: Apply a Dev Container Template to a workspace
Code:
devcontainer templates apply --template-id template_id --template-args template_args --workspace-folder path/to/workspace
Motivation:
Developers often work on projects with similar setups, like a Python development environment configurations. By using the apply
command, one can rapidly scaffold a new workspace with all requisite configurations without starting from scratch each time. This promotes standardization in the team and reduces the initialization time for new projects.
Explanation:
--template-id template_id
: Specifies the unique identifier of the template to be applied. This ensures the correct environment is instantiated.--template-args template_args
: Allows additional parameters to be passed for customizing the template application, giving developers flexibility to meet specific project needs.--workspace-folder path/to/workspace
: Designates the target directory where the template should be applied, ensuring that the correct project folder is set up.
Example Output:
- Applying template ‘Python3-WebApp’ to workspace ‘/user/project’…
- Template applied successfully. Workspace is now ready with specified configurations.
Use case 3: Execute a command on a running Dev Container in the current workspace
Code:
devcontainer exec command
Motivation:
Executing arbitrary commands inside a running container can be a critical part of development and debugging workflows. This feature allows developers to run scripts, install packages, or test their applications without leaving the container environment, thus maintaining consistency and reducing context-switching.
Explanation:
exec
is a sub-command that specifies an operation requiring execution within the confines of the running Dev Container, thereby ensuring operations respect the containerized environment configurations.command
refers to any shell command or script that should be run within the container, providing developers the flexibility to perform various operations without exiting their current development setup.
Example Output:
- Running command:
npm start
- Application is now running on port 3000 within the Dev Container.
Use case 4: Build a Dev Container image from devcontainer.json
Code:
devcontainer build path/to/workspace
Motivation:
Building a Docker image ensures that your development environment, with all its dependencies and configurations, can be reliably recreated. Useful in scenarios where developers need to validate changes to dependencies or configurations and prepare versions of the environment for sharing or deployment.
Explanation:
build
indicates the action of creating a Docker image from the configuration.path/to/workspace
specifies the directory containing thedevcontainer.json
file. This path represents where the configuration and any app-specific context are located.
Example Output:
- Reading ‘devcontainer.json’ configuration…
- Docker image ‘my-devcontainer’ built successfully.
Use case 5: Open a Dev Container in VS Code (the path is optional)
Code:
devcontainer open path/to/workspace
Motivation:
Integrating containerized development environments directly into an IDE like VS Code provides an enhanced development experience, combining the benefits of modern code editors with the consistency of container environments. This feature is pivotal for developers who want to leverage code editor features, such as autocomplete or code debuggers, within a standardized environment.
Explanation:
open
triggers VS Code to launch the specified or current workspace within a Dev Container, providing an integrated development setting.path/to/workspace
sets the path for the workspace to be loaded. If omitted, the default or current workspace directory will be used.
Example Output:
- Opening VS Code with workspace at ‘/user/project’…
- Dev Container ready. You are now in a containerized IDE environment.
Use case 6: Read and print the configuration of a Dev Container from devcontainer.json
Code:
devcontainer read-configuration
Motivation:
Understanding the setup of your development environment is crucial, especially when troubleshooting or modifying configurations. This command lets developers view the current settings and configurations without manually navigating and reading cumbersome configuration files, streamlining the process of auditing or revising environment settings.
Explanation:
read-configuration
instructs thedevcontainer
tool to parse and publicly list the settings currently defined indevcontainer.json
, allowing for quick insight and inspection.
Example Output:
- Retrieving configuration…
- Configuration: {“name”: “Node.js”, “extensions”: [“dbaeumer.vscode-eslint”], “forwardPorts”: [3000]}
Conclusion:
The devcontainer
command’s variety of use cases cater to the diverse needs of modern development practices, from setting up environments and executing commands within containers to integrating these setups seamlessly into development workflows using VS Code. Its adoption simplifies the management of development environments, focuses on replicating production conditions, and saves ample time, allowing developers to concentrate on building robust applications.