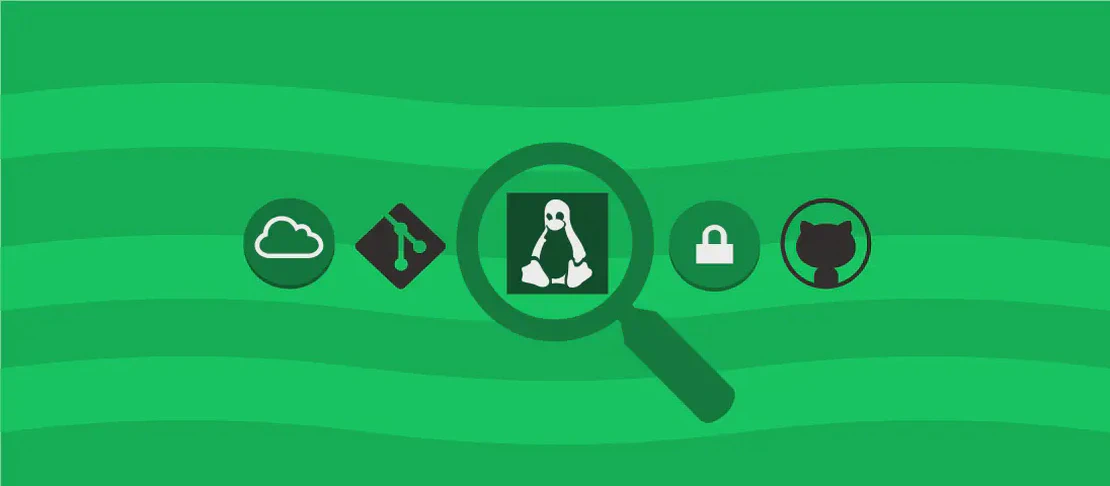
How to use the command 'django-admin' (with examples)
Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. At the heart of Django’s utility, there is the django-admin
command, a powerful tool to automate various administrative tasks. This command-line utility allows developers to quickly scaffold new projects, create apps, check versions, and access command-specific help. Here, we explore how to employ the django-admin
command effectively, along with detailed examples.
Use case 1: Create a new Django project
Code:
django-admin startproject project_name
Motivation:
Creating a new project is usually the first step in any Django development process. When you initiate a new project, Django sets up a directory structure, including several useful default configurations for your web application. This ensures a structured and organized beginning, minimizing errors and making your code base more manageable from the start.
Explanation:
startproject
: This is the command that tellsdjango-admin
to create a new project. It scaffolds a basic structure that includes files and directories essential for a Django project.project_name
: This placeholder should be replaced with your desired project name. It will be used to create a directory with that name which contains the project’s settings and application-specific files.
Example Output:
Upon executing the command, you will typically see a new directory named project_name
with subdirectories and files like manage.py
, settings.py
, urls.py
, and other necessary components.
Use case 2: Create a new app for the current project
Code:
django-admin startapp app_name
Motivation:
A Django project can have multiple apps, each serving a distinct purpose. For example, an ecommerce project might have separate apps for user profiles, product listings, and checkout functionality. This modular approach allows for greater reusability and maintainability of code. Creating a new app is crucial whenever a new piece of functionality is required in the project.
Explanation:
startapp
: This part of the command instructsdjango-admin
to create a new app within an existing Django project. Apps encapsulate specific functionality, making them easy to manage.app_name
: This is a placeholder for the app’s name. It creates a directory with this name that contains default directories and files required for the app.
Example Output:
Upon execution, a new directory named app_name
will be created with files like models.py
, views.py
, tests.py
, among others, ready to be customized according to the app’s functionality.
Use case 3: Check the current version of Django
Code:
django-admin --version
Motivation:
Knowing the Django version you are working with can be vital, especially when referencing documentation or ensuring compatibility with libraries and Python versions. This is often one of the first checks when setting up an existing project in a new environment.
Explanation:
--version
: This is an argument that tellsdjango-admin
to print out the installed Django version. It’s useful for confirming the exact version you are working with.
Example Output:
The command outputs the version number, such as 4.2.3
, directly into the terminal, confirming the Django version currently installed.
Use case 4: Display help for a specific command
Code:
django-admin help command
Motivation:
New developers or those working with unfamiliar commands might require detailed usage instructions. The help
argument provides specific guidance for executing any django-admin
command properly, ensuring developers can leverage the full power of Django’s capabilities.
Explanation:
help
: This subcommand provides extensive help details for anotherdjango-admin
command.command
: Replace this with any specific command name for which you need further information. It details options, arguments, and usage examples.
Example Output:
When run with a valid command, such as startproject
, it will list helpful information regarding that command, aiding developers in correctly utilizing the option.
Conclusion:
The django-admin
command is an indispensable tool for Django development, streamlining administrative tasks and making the developer’s life easier through automation. Whether it’s setting up new projects, creating apps, verifying Django versions, or getting help for specific commands, mastering the use of django-admin
is a step toward efficient and effective Django development. Each example highlighted here not only showcases individual use cases but underscores the broader importance of organized and intelligent work processes in the world of web development with Django.