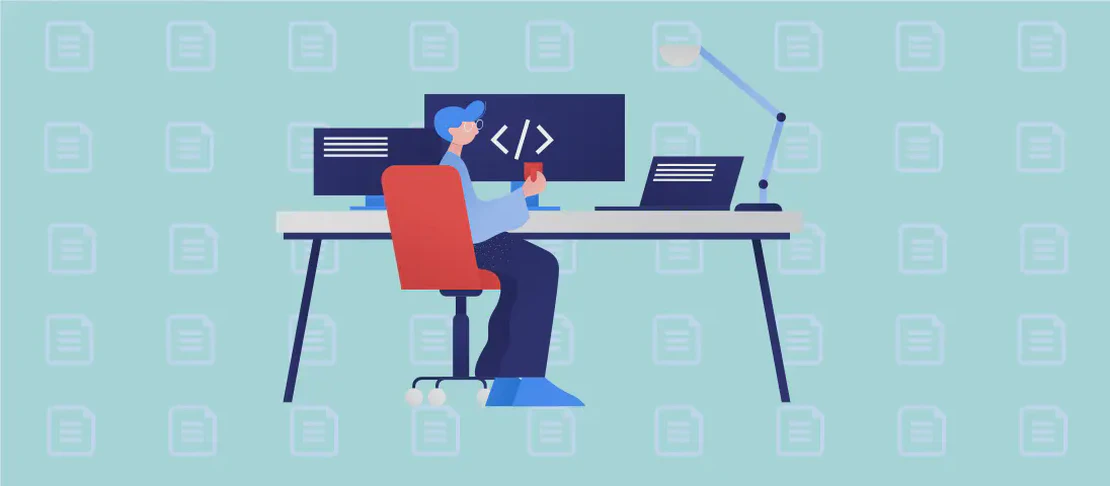
How to Use the Command 'dlv' (with examples)
Delve (dlv
) is a powerful debugger tailored for the Go programming language, allowing developers to debug Go programs effectively. It offers a variety of features that enable step-by-step debugging, which is essential to understand the flow and state of a program at runtime. Delve is designed to work seamlessly with Go tools, making it an indispensable utility for developers involved in Go programming. Below, we explore different use cases of the dlv
command to aid in various debugging scenarios.
Use case 1: Compile and Begin Debugging the Main Package in the Current Directory
Code:
dlv debug
Motivation:
When working on a Go project, especially during the development of a new feature or while fixing bugs, you may need to start the debugger quickly with minimal setup. Using dlv debug
allows you to compile and immediately start debugging the main package located in your current directory. This can significantly speed up the debugging process by removing the need for additional parameters and focusing solely on the local code.
Explanation:
dlv
: The command to invoke the Delve debugger.debug
: This subcommand tells Delve to compile and start debugging the package in the current directory. It defaults to themain
package if no arguments are provided.
Example Output:
Upon executing the command, Delve will compile the main package and start the interactive debugger session, presenting the user with a CLI for stepping through the code.
Type 'help' for list of commands.
(dlv)
Use case 2: Compile and Begin Debugging a Specific Package
Code:
dlv debug ./my/package
Motivation:
Sometimes, you might want to focus on a specific package rather than the entire project. This is particularly useful when the main application is too cumbersome to debug in one go, or if you’re working on a library or module independently. Debugging a specific package allows you to isolate issues and test functionality specific to that component without the noise from other parts of the codebase.
Explanation:
dlv
: Invokes the Delve debugger.debug
: Directs Delve to compile and debug the designated package../my/package
: Specifies the path to the particular package you wish to debug. Adjust this path to match the location of your target package.
Example Output:
After running the command, Delve compiles and prepares the specified package for debugging, starting a new session.
Type 'help' for list of commands.
(dlv)
Use case 3: Compile a Test Binary and Begin Debugging the Compiled Program
Code:
dlv test
Motivation:
Debugging test cases is just as crucial as debugging application code. Tests ensure your code reacts correctly to various inputs and scenarios. The dlv test
command compiles and prepares your test suite for debugging, allowing you to step through each test case methodically to identify flaws in logic or unexpected behavior.
Explanation:
dlv
: Calls the Delve debugger.test
: Command that compiles the test binary for the current package and initiates a debug session. It operates over test files with a_test.go
suffix, executing them within the debugger.
Example Output:
Starting a Delve session over test files provides an environment to test breakpoints within your test logic.
Type 'help' for list of commands.
(dlv)
Use case 4: Connect to a Headless Debug Server
Code:
dlv connect 192.168.1.100:2345
Motivation:
There are scenarios where the application you need to debug isn’t running on your local machine, possibly due to constraints like environment dependencies or hardware-specific behavior. In such cases, starting a headless Delve debug server on a remote machine and connecting to it allows you to handle remote debugging efficiently. It is incredibly helpful for debugging server applications, reducing the distance between the developer and the deployed environment.
Explanation:
dlv
: Initiates the debugger.connect
: Starts a session to connect to a remote headless Delve instance.192.168.1.100:2345
: Specifies the IP address and port number of the remote machine running the Delve headless server to which you want to connect.
Example Output:
After successfully connecting, you can control the debugging session remotely from your terminal.
Type 'help' for list of commands.
(dlv)
Use case 5: Attach to a Running Process and Begin Debugging
Code:
dlv attach 12345
Motivation:
Occasionally, you need to debug a program that is already running rather than restarting it, which might disrupt its current state. Whether it’s reproducing a rare bug or diagnosing performance issues, attaching Delve to an active process allows you to inspect the current execution context without interrupting business continuity, especially useful in production environments.
Explanation:
dlv
: Calls the Delve debugger.attach
: Instructs Delve to connect to an existing in-progress process.12345
: Represents the Process ID (PID) of the running Go program you want to debug. Replace it with the appropriate PID of your target process.
Example Output:
The command attaches to the specified process, permitting real-time analysis.
Type 'help' for list of commands.
(dlv)
Use case 6: Compile and Begin Tracing a Program
Code:
dlv trace ./my/package --regexp 'Handle.*'
Motivation:
Tracing is crucial when needing to understand how specific functions execute within your codebase. Perhaps you’re interested in seeing every function call starting with “Handle” to trace how requests are being processed through various handlers. This tracing mechanism provides insight into execution flow patterns and interactions between components.
Explanation:
dlv
: Begins the debugging with Delve.trace
: Directs Delve to compile for tracing opposed to step-through debugging../my/package
: Specifies the package to trace.--regexp 'Handle.*'
: Uses a regular expression to define which functions should be traced, capturing any functions that match the given pattern.
Example Output:
Output will document all the matches and path through which the execution occurred, making it easier to follow execution flow.
Tracepoint 1: ./my/package/somefile.go:42 HandleRequest()
Tracepoint 2: ./my/package/otherfile.go:133 HandleResponse()
...
Conclusion:
Delve (dlv
) is an essential tool for Go developers, offering a spectrum of debugging and tracing capabilities tailored to assist in both development and production environments. From compiling main packages, debugging specific libraries, or connecting to remote servers, Delve empowers its users to precisely diagnose and fix issues with targeted, sophisticated debugging techniques. Through these examples, developers can refine their debugging workflows to match their specific needs and development style.