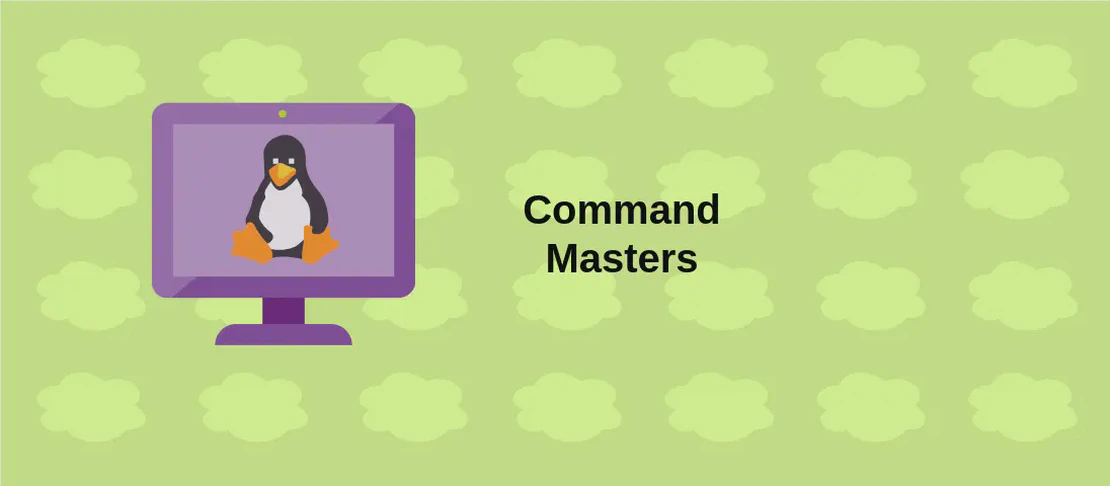
How to Use the Command 'dmd' (with examples)
The ‘dmd’ command is the official compiler for the D programming language, a language known for its powerful features supporting high-performance applications. This compiler allows developers to build and compile D source files, optimizing them for different instantiations and settings. It is widely used by D developers to translate their written code into executable programs, ensuring they run safely and efficiently on their target platforms. Here, we explore different use cases and examples of the ‘dmd’ command to better understand its functionality and versatile application.
Use case 1: Build a D Source File
Code:
dmd path/to/source.d
Motivation:
When developing software in the D programming language, compiling your source files into executable binaries is a crucial step. The command dmd path/to/source.d
simplifies this process by allowing you to compile your D source file with a single command, making it easier for developers to test and deploy their applications efficiently.
Explanation:
dmd
: This is the command to use the D compilers.path/to/source.d
: This argument specifies the path to the D source file you want to compile. Thed
extension indicates a D file.
Example Output:
If the source file compiles successfully, you will get an output indicating that an executable has been created. If there are errors in the code, they will be highlighted in the command line output, helping developers pinpoint issues to correct.
Use case 2: Generate Code for All Template Instantiations
Code:
dmd -allinst
Motivation:
In D programming, templates are a powerful feature used to write generic and reusable code. However, managing the instantiations of these templates can be challenging. The -allinst
flag ensures every template instantiation is accounted for, which is particularly useful when cross-referencing or debugging complex projects where template behaviors must be explicitly confirmed.
Explanation:
dmd
: Uses the D compiler.-allinst
: This flag tells the compiler to generate code for all instantiated templates within your project. It ensures no instantiation is left behind, aiding in thorough debugging and testing.
Example Output:
The compilation process will list all the template instantiations used in your project, giving you confidence that all necessary code paths are covered.
Use case 3: Control Bounds Checking
Code:
dmd -boundscheck=on
Motivation:
Bounds checking is crucial for preventing errors like buffer overflows, which can lead to security vulnerabilities. The -boundscheck
option allows a developer to specify the level of bounds checking required, supporting safer application execution and helping catch potential out-of-bounds errors during development.
Explanation:
dmd
: Indicates the use of the D compiler.-boundscheck=on
: This argument enables bounds checking for all array and pointer indexes, ensuring they are within their valid range during the program execution, thus enforcing safer code.
Example Output:
When this option is enabled, if any operation attempts to access an out-of-bounds index in your code, an exception will be thrown, allowing you to handle it gracefully or fix the code logic.
Use case 4: List Information on All Available Checks
Code:
dmd -check=h
Motivation:
Understanding the various checks available to you as a developer is intrinsic to writing robust code. The -check
option provides a quick way to list all available checks that can be enforced during compilation, aiding in enhancing the reliability and security of your programs.
Explanation:
dmd
: Calls the D compiler.-check=h
: This argument lists all available checking options you can specify, described in the help text (h
is shorthand for help).
Example Output:
The command outputs a comprehensive list of all checks along with their descriptions, guiding you on how to use them to improve the correctness of your code.
Use case 5: Turn on Colored Console Output
Code:
dmd -color
Motivation:
Visual cues can significantly enhance the readability of console outputs. With the -color
option, you can activate colored output in your terminal, making error messages, warnings, and other diagnostic information easier to spot and interpret. This is particularly useful when dealing with extensive code or complex error messages.
Explanation:
dmd
: Uses the D compiler.-color
: Activates colored output in the console, adding visual differentiation for various types of messages returned during the compilation process.
Example Output:
When compiling with this option, different messages will be color-coded based on their type, such as errors displayed in red and warnings in yellow, assisting developers in quickly identifying problem areas in their code.
Conclusion:
By utilizing the various command-line options available with dmd
, you can effectively manage your D programming projects with precision and efficiency. From compiling source files and debugging template instantiations to employing safety checks and enhancing console output, these examples demonstrate the command’s versatility and importance in supporting developers to produce optimized and robust software.