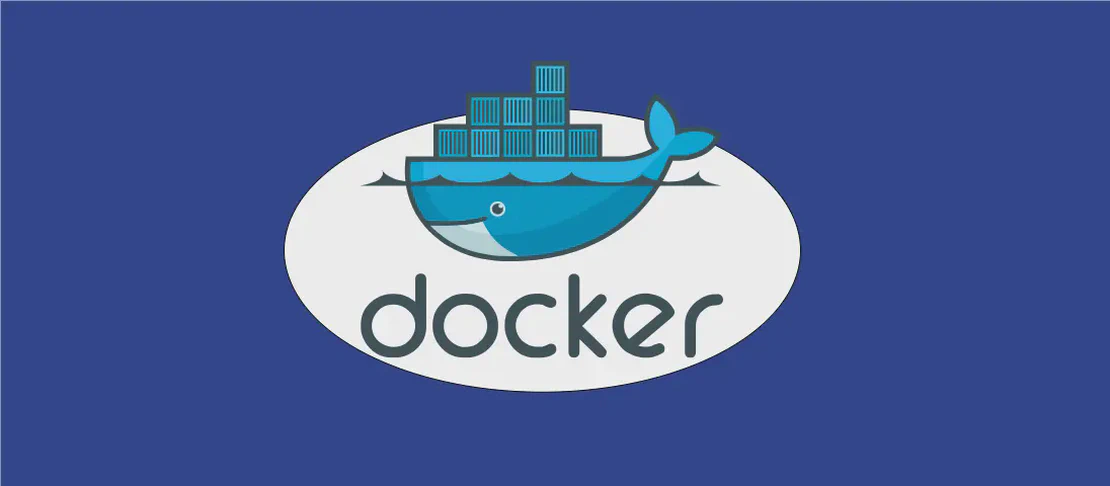
How to use the command 'docker build' (with examples)
The docker build
command is an integral part of Docker, a platform used to develop, ship, and run applications inside containers. This command allows users to create a Docker image from a Dockerfile, capturing a complete snapshot of an application and its dependencies, which can be used to deploy applications in any environment.
Build a Docker image using the Dockerfile in the current directory
Code:
docker build .
Motivation:
In software development, establishing a consistent and reproducible environment is crucial. This use case represents the most straightforward and common scenario where a Docker image is built using the Dockerfile located in the current directory. By executing this command, developers can easily encapsulate their application in a container for deployment or sharing.
Explanation:
docker build
: Invokes the Docker build command..
: The dot (.) specifies the current directory as the build context, which includes all files in the directory. During the build, Docker reads the Dockerfile from this directory to construct the image.
Example Output:
Sending build context to Docker daemon 3.072kB
Step 1/3 : FROM python:3.8-slim
---> 4b7dc3a3f135
Step 2/3 : COPY . /app
---> Using cache
---> 1234abcd5678
Step 3/3 : CMD ["python", "/app/script.py"]
---> Using cache
---> abcd56781234
Successfully built abcd56781234
Build a Docker image from a Dockerfile at a specified URL
Code:
docker build github.com/creack/docker-firefox
Motivation:
Remote code repositories are often used for continuous integration and deployment pipelines. This command allows users to create a Docker image directly from a repository URL containing a Dockerfile. It’s an efficient way to automate builds without needing local copies of files.
Explanation:
docker build
: Initiates the Docker image creation process.github.com/creack/docker-firefox
: This URL points to a remote repository containing the application’s Dockerfile. Docker fetches the Dockerfile from this location to build the image.
Example Output:
Cloning into 'docker-firefox'...
remote: Enumerating objects: 10, done.
remote: Total 10 (delta 0), reused 0 (delta 0)
Receiving objects: 100% (10/10), 13.00 KiB | 1.00 MiB/s, done.
Sending build context to Docker daemon 3.584kB
Step 1/4 : FROM debian:buster
---> f5ab0e391d02
Step 2/4 : COPY . /app
---> Using cache
---> e67f6d4e2834
Step 3/4 : RUN apt-get update && apt-get install -y firefox
---> Running in 5d2emoic8hf4
Removing intermediate container 5d2emoic8hf4
---> 776b41g64fae
Step 4/4 : CMD ["firefox"]
---> Using cache
---> 18ea7be0130n
Successfully built 18ea7be0130n
Build a Docker image and tag it
Code:
docker build --tag myapp:1.0 .
Motivation:
Tagging images is a critical practice for version control and management within Docker registries. By tagging an image, developers can easily track and identify specific versions or releases, facilitating deployment and rollback operations.
Explanation:
docker build
: Initiates the build process.--tag myapp:1.0
: Assigns the tagmyapp:1.0
to the image, wheremyapp
is the repository name and1.0
is the version. Tags are crucial for managing different versions in a Docker registry..
: Specifies the current directory as the build context.
Example Output:
Sending build context to Docker daemon 2.048kB
Step 1/5 : FROM nginx:alpine
---> 1e43e5d73f61
Step 2/5 : COPY . /usr/share/nginx/html
---> 876543210fed
Step 3/5 : EXPOSE 80
---> Using cache
---> f1edcba98765
Step 4/5 : CMD ["nginx", "-g", "daemon off;"]
---> Running in b3c9bv7i0k3e
Removing intermediate container b3c9bv7i0k3e
---> 47cb6abcd543
Successfully built 47cb6abcd543
Successfully tagged myapp:1.0
Build a Docker image with no build context
Code:
docker build --tag myapp:2.0 - < Dockerfile
Motivation:
In scenarios where the Docker build process does not require access to additional files, bypassing the build context can lead to efficiency gains, especially when the build context would otherwise be large or unnecessary. This approach is useful for simple Dockerfiles or when security constraints limit file access.
Explanation:
docker build
: Commences the Docker image creation.--tag myapp:2.0
: Tags the image withmyapp:2.0
.-
: The dash (-
) signifies no context will be sent, requiring the Dockerfile to be piped directly from the standard input.< Dockerfile
: Redirects the contents of theDockerfile
into the build process, enabling Docker to build the image without an explicit context.
Example Output:
Step 1/4 : FROM node:14
---> 39ff6a75c259
Step 2/4 : WORKDIR /app
---> Running in 83de7gf94bh2
Removing intermediate container 83de7gf94bh2
---> be5cb1b2d159
Step 3/4 : COPY package*.json ./
---> 29fb78dc0f10
Step 4/4 : CMD ["node", "app.js"]
---> Running in c4sd8hd7w6e
Removing intermediate container c4sd8hd7w6e
---> ac9d6eb01234
Successfully built ac9d6eb01234
Successfully tagged myapp:2.0
Do not use the cache when building the image
Code:
docker build --no-cache --tag myapp:1.1 .
Motivation:
Caching is used to speed up builds, but there are times when the changes in the Dockerfile or source files should not rely on cache, such as during debugging or when a base image has been updated. This use case ensures a fresh build by bypassing the cache.
Explanation:
docker build
: Triggers the build process.--no-cache
: Disables the usage of cached layers during the build, prompting Docker to rebuild every layer from scratch.--tag myapp:1.1
: Applies themyapp:1.1
tag to the image..
: Designates the current directory as the build context.
Example Output:
Step 1/5 : FROM ubuntu:20.04
---> 1d7f45f744f7
Step 2/5 : RUN apt-get update
---> Running in 97eabc123lmn
Removing intermediate container 97eabc123lmn
---> def1a0cdaf92
Step 3/5 : RUN apt-get install -y wget
---> Running in 46n89b54hjx7
Removing intermediate container 46n89b54hjx7
---> cd9ba8b7v654
Step 4/5 : COPY . /app
---> 18e654a98765
Step 5/5 : CMD ["./app/start.sh"]
---> Running in 39ei9db7gj67
Removing intermediate container 39ei9db7gj67
---> 456ae9v34612
Successfully built 456ae9v34612
Successfully tagged myapp:1.1
Build a Docker image using a specific Dockerfile
Code:
docker build --file CustomDockerfile .
Motivation:
In projects with multiple Dockerfiles, for instance, different environments (development, testing, production) might require separate configurations. This use case specifies which Dockerfile to use for the build, providing developers flexibility and control.
Explanation:
docker build
: Commences the build operation.--file CustomDockerfile
: SpecifiesCustomDockerfile
to be used instead of the defaultDockerfile
..
: Uses the current directory as the build context.
Example Output:
Step 1/3 : FROM golang:1.16
---> 6cdb1a2929c9
Step 2/3 : ADD . /src
---> 7ebe6dc123af
Step 3/3 : RUN go build -o /app /src
---> Running in 9fb1c90jh4c8
Removing intermediate container 9fb1c90jh4c8
---> cde8dfe5a76a
Successfully built cde8dfe5a76a
Successfully tagged example/go-build:latest
Build with custom build-time variables
Code:
docker build --build-arg HTTP_PROXY=http://10.20.30.2:1234 --build-arg FTP_PROXY=http://40.50.60.5:4567 .
Motivation:
Occasionally, builds need environment-specific variables like proxies or API keys without hardcoding them into the Dockerfile, making builds more adaptable and secure. This example allows setting these variables at build time to alter the behavior or configuration of software inside the Docker image.
Explanation:
docker build
: Initiates the process to build a Docker image.--build-arg HTTP_PROXY=http://10.20.30.2:1234
: Sets a build-time argumentHTTP_PROXY
with the specified proxy URL.--build-arg FTP_PROXY=http://40.50.60.5:4567
: Assigns a proxy URL to theFTP_PROXY
build argument. Multiple--build-arg
flags are supported for numerous variables..
: Specifies that the current directory is to be used as the build context.
Example Output:
Sending build context to Docker daemon 4.096kB
Step 1/4 : FROM alpine:latest
---> 54b8a2f4881b
Step 2/4 : ARG HTTP_PROXY
---> Running in 64de9bc123mn
Removing intermediate container 64de9bc123mn
---> 1234d5f9fa22
Step 3/4 : ENV HTTP_PROXY ${HTTP_PROXY}
---> Running in a5d1e0v543j2
Removing intermediate container a5d1e0v543j2
---> e56v2b2c86ab
Step 4/4 : RUN apk update && apk add curl
---> Running in d7h9vo9m5i4k
Removing intermediate container d7h9vo9m5i4k
---> ef678dabcd12
Successfully built ef678dabcd12
Conclusion:
The docker build
command is a versatile and powerful tool essential for creating Docker images tailored to different environments and use cases. Each use case provides unique options to fine-tune the build process, optimize runtime performance, and maintain consistency across development environments. Whether tagging images, building without a cache, or specifying build arguments, these examples demonstrate how docker build
can meet diverse application needs.