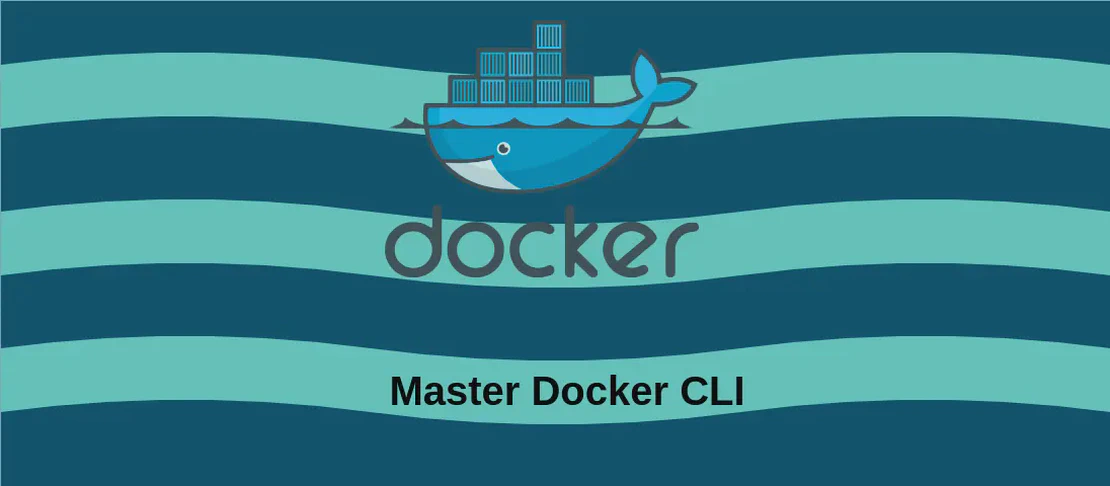
How to Use the Command 'docker commit' (with examples)
The docker commit
command is a powerful utility in Docker that allows users to create a new image from changes made to an existing container. This means that any modifications applied to a running container can be saved permanently in the form of a new Docker image. This process facilitates versioning and the ability to replicate a configured environment.
Create an Image from a Specific Container
Code:
docker commit container image:tag
Motivation:
Creating an image from a specific container is crucial when you have made modifications or deployed specific software configurations within a running Docker container that you’d like to reproduce. By saving these changes into an image, it becomes easy to create additional containers with the same environment setup, ensuring consistency across deployments.
Explanation:
container
: This is the name or ID of the running container from which you’re creating the new image. It serves as the source of the snapshot.image:tag
: Defines the name and optional tag of the image created. The tag is a useful way to version the image.
Example output:
sha256:a9409f8a7ffbbd6c2b5ede2f5d1205d9a5e3fd3796e07d238d7bdf9b4feae4af
Apply a CMD
Dockerfile Instruction to the Created Image
Code:
docker commit --change "CMD command" container image:tag
Motivation:
The CMD
instruction in a Dockerfile specifies the default command to be run when the container starts. Using the --change
flag with a CMD
instruction while committing allows you to set or change the default startup command of the image you are creating. This is particularly useful when you need the container to start with a specific service running by default.
Explanation:
--change "CMD command"
: The--change
flag applies Dockerfile instructions to the image during the commit.CMD command
sets the command that should run when the container starts.container image:tag
: As in the previous use case, this specifies the source container and the new image name.
Example output:
sha256:cd9b61b6e340b8a229ac0fbf8d575f4d5214ff3fed26633367db6aaea8f8e270
Apply an ENV
Dockerfile Instruction to the Created Image
Code:
docker commit --change "ENV name=value" container image:tag
Motivation:
Applying an ENV
variable during a commit allows you to inject environment variables directly into your Docker image. These variables can be crucial for situations such as setting up a development or production environment, configurable settings, or secret management.
Explanation:
--change "ENV name=value"
: This flag sets an environment variable directly into the new image. It is a way to pass configuration details without modifying your application’s source code.container image:tag
: This syntax remains consistent for defining the source container and the new image.
Example output:
sha256:eb7e3c1cb6f223e8f003f53587219acf176a561bb3a61adbdf4530e80c10f098
Create an Image with a Specific Author in the Metadata
Code:
docker commit --author "author" container image:tag
Motivation:
Adding an author to the image metadata can be beneficial for maintaining records of who made specific changes or built the image. This is especially useful in team environments where multiple developers or operators interact with the container infrastructure.
Explanation:
--author "author"
: This option adds an informational label to the image metadata indicating who committed the changes. It helps in tracking the image’s provenance.container image:tag
: Identifies the container and provides a name to the image as usual.
Example output:
sha256:b87c981c8838ef8a87da1e7d65c9d6d3a17263a986eb8428b78463ae0099e772
Create an Image with a Specific Comment in the Metadata
Code:
docker commit --message "comment" container image:tag
Motivation:
Comments in metadata help document the purpose or changes involved in the image. This can assist users in understanding what alterations have been incorporated, thereby facilitating easier troubleshooting and image management.
Explanation:
--message "comment"
: This flag allows users to add a string comment to the image metadata to serve as a changelog or descriptor.container image:tag
: Represents the container and the new image naming convention once again.
Example output:
sha256:019fbaf2c12bf6ceb2a2ec6abd7dd159a222a379494026a8a8b07a5d7c0e49a4
Create an Image Without Pausing the Container During Commit
Code:
docker commit --pause false container image:tag
Motivation:
Pausing a container can be disruptive to services especially in production environments where downtime should be minimized. Preventing pause during the commit ensures continuity of services while still capturing the current state of the container.
Explanation:
--pause=false
: This option ensures that the container continues running during the commit operation. The default behavior is to pause and freeze all processes in the container while the commit is taking place, which can be problematic for stateful applications.container image:tag
: As always, indicates the container and desired new image identity.
Example output:
sha256:7b91823cd42150f23c9e9cd04c9937a9a3e1fea5ff7515b903b92f422df11b14
Display Help
Code:
docker commit --help
Motivation:
Displaying help is crucial for quickly recalling command options and syntax, especially for users who are in the process of learning or who do not frequently use the complete set of options.
Explanation:
--help
: This flag prints the help message and usage information for thedocker commit
command, detailing all available options and flags.
Example output:
Usage: docker commit [OPTIONS] CONTAINER [REPOSITORY[:TAG]]
Create a new image from a container's changes
Options:
-a, --author string Author (e.g., "name <email@example.com>")
-c, --change list Apply Dockerfile instruction to the created image
-m, --message string Commit message
--pause Pause container during commit (default true)
Conclusion
The docker commit
command offers a flexible way to secure changes made to running containers into a reproducible Docker image. Whether it’s preserving environment variables, specifying startup commands, or ensuring continuity without service interruption, docker commit
provides the means to ensure your container configurations can be deployed consistently and reliably across different environments.