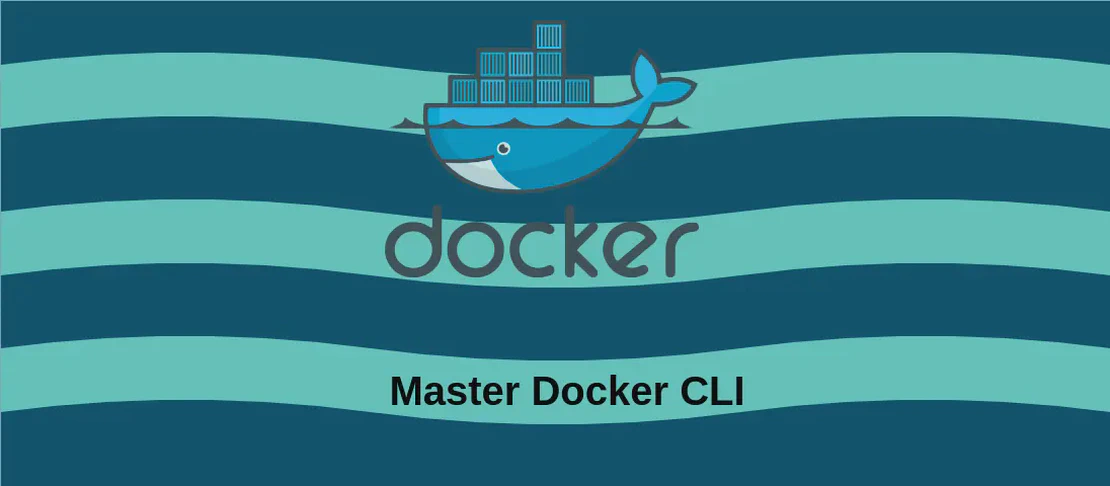
How to Use the Command 'docker' (with examples)
Docker is a platform designed to enable the easy creation, deployment, and running of applications by using containers. These containers package up an application with all the parts it needs, such as libraries and other dependencies, and ship it as one package. Docker helps ensure that software will always run the same way, regardless of where it’s deployed. It’s widely used in development, testing, and deployment processes due to its efficiency and ease of use.
Use Case 1: Listing All Docker Containers (Running and Stopped)
Code:
docker ps --all
Motivation: When working with Docker, it’s important to have visibility into all containers that exist on your system, whether they are currently running or stopped. This insight is crucial for managing resources, troubleshooting, and ensuring the proper handling of containerized applications.
Explanation:
docker ps
: This command by itself lists all the currently running containers.--all
or-a
: This flag extends the functionality ofdocker ps
to include all containers, not just the ones that are currently running. This is helpful for managing stopped containers, which may need to be restarted, modified, or removed.
Example Output:
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
ae3995b9ac2e ubuntu "/bin/bash" 3 days ago Exited (0) 42 hours ago silly_hugle
5eb281deeaef httpd "httpd-foreground" 5 days ago Up 5 days 80/tcp web_server_01
Use Case 2: Start a Container from an Image, with a Custom Name
Code:
docker run --name container_name image
Motivation: Assigning a custom name to a Docker container simplifies managing and referencing the container. Custom names are beneficial in environments with multiple containers, making it easier to recognize the role or purpose of each one.
Explanation:
docker run
: This command creates and starts a new container from a specified image.--name
: This option assigns a custom name to the container, making it easier to reference and manage later.container_name
: Replace this with your desired container name.image
: Specify the Docker image you want to create the container from.
Example Output:
Unable to find image 'nginx:latest' locally
latest: Pulling from library/nginx
55cbf04beb70: Pull complete
...
Digest: sha256:c619295fb5aad34581ad11011c3b4c8e...
Status: Downloaded newer image for nginx:latest
Use Case 3: Start or Stop an Existing Container
Code:
docker start|stop container_name
Motivation: Managing the lifecycle of a Docker container is crucial for efficient utilization of resources. Starting and stopping containers as needed helps to control environmental factors, cost, and resource allocation decisively.
Explanation:
docker start
: This command activates a stopped container.docker stop
: This command shuts down a running container gracefully.container_name
: Replace this with the name or ID of the container you intend to start or stop.
Example Output:
Container web_server_01 started
Or:
Container web_server_01 stopped
Use Case 4: Pull an Image from a Docker Registry
Code:
docker pull image
Motivation: Pulling an image from a Docker registry allows you access to pre-packaged software environments. This is fundamentally important when you need an image that is ready to be run or built upon without starting from scratch.
Explanation:
docker pull
: Downloads a Docker image from a registry.image
: This is the identifier of the image you want to download, including optional tags to specify its version.
Example Output:
Using default tag: latest
latest: Pulling from library/mysql
Digest: sha256:b3c5c60a62e...
Status: Image is up to date for mysql:latest
Use Case 5: Display the List of Already Downloaded Images
Code:
docker images
Motivation: Having a list of downloaded images readily available is essential for developers to track the images they have on their systems. This ensures they can efficiently manage disk space, update old versions, and use the correct image for creating containers.
Explanation:
docker images
: This command shows all Docker images currently stored on the local machine, including details like repository name, tags, image ID, and more.
Example Output:
REPOSITORY TAG IMAGE ID CREATED SIZE
nginx latest 6d56a8dc4490 2 weeks ago 109MB
ubuntu latest 78b84c3c8486 3 weeks ago 72.9MB
Use Case 6: Open an Interactive TTY with Bourne Shell (sh
) inside a Running Container
Code:
docker exec -it container_name sh
Motivation: Accessing the shell of a running container is invaluable for troubleshooting, configuration, and testing purposes. It allows developers to inspect the container’s filesystem, check logs, or run commands directly within the container’s environment.
Explanation:
docker exec
: This command runs a command within an existing container.-it
: Combines two flags:-i
keeps STDIN open even if not attached, and-t
allocates a pseudo-TTY, essentially emulating terminal behavior within the container.container_name
: The name or ID of the container you want to access.sh
: Specifies that you want to open a shell session inside the container.
Example Output:
root@ae3995b9ac2e:/#
Use Case 7: Remove a Stopped Container
Code:
docker rm container_name
Motivation: Freeing up system resources by deleting unused containers is essential for maintaining a clean and efficient working environment. Removing stopped containers also helps prevent confusion about which containers are actively serving application purposes.
Explanation:
docker rm
: This command removes one or more stopped containers from the system.container_name
: The name or ID of the container you wish to remove.
Example Output:
container_name
Use Case 8: Fetch and Follow the Logs of a Container
Code:
docker logs -f container_name
Motivation: Monitoring logs in real time is crucial for debugging and ensuring that applications running inside containers are operating as intended. The ability to view logs dynamically helps quickly identify issues and improve application performance.
Explanation:
docker logs
: Retrieves logs from a running or stopped container.-f
: Follows the log output, continuing to display new log entries as they are generated.container_name
: The container’s name or ID from which you wish to fetch logs.
Example Output:
[date] [INFO] Starting process..
[date] [ERROR] Failed to connect to database
...
Conclusion
Docker commands offer significant capabilities in managing containers and images, each serving distinct purposes like creation, monitoring, and deletion. Understanding these commands, their flags, and options is vital for efficiently leveraging Docker technology to enhance application development, deployment, and operational workflows. From listing containers to pulling images and exploring logs, these commands form the backbone of effective Docker management.