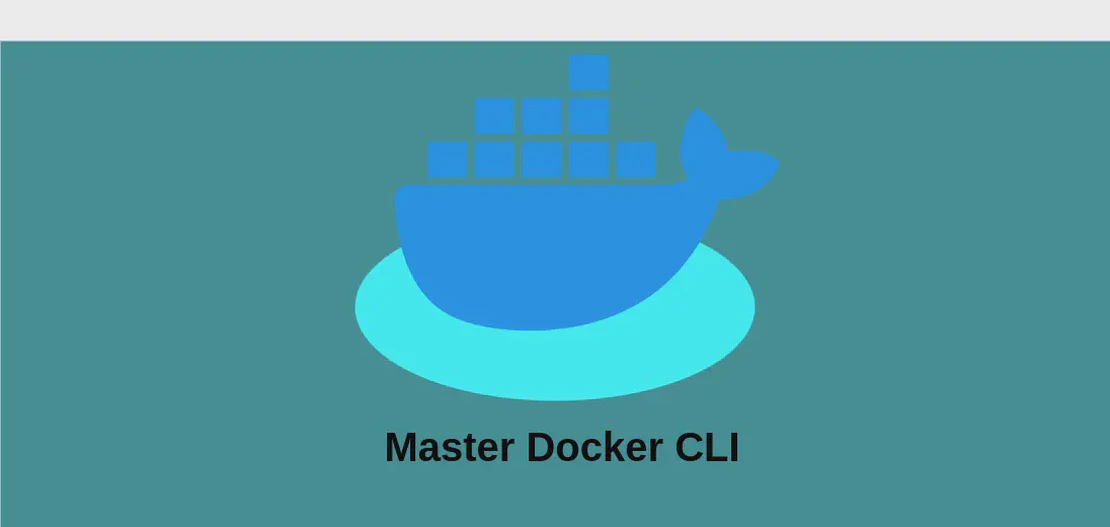
How to Use the Command 'docker compose' (with examples)
Docker Compose is a tool that simplifies the process of running multi-container Docker applications. By reading service definitions from a YAML file, it allows you to create, start, stop, and manage entire environments consistently and with minimal effort. It abstracts the complexity involved in bringing together individual container configurations, functioning as a convenient method for deploying distributed applications. Here, we’ll delve into various common use cases of the docker compose
command.
Use case 1: List All Running Containers
Code:
docker compose ps
Motivation:
Monitoring running containers is crucial for maintaining smooth operations and quickly diagnosing potential issues. With Docker Compose, you often run multiple containers across different services, and knowing their current status helps in effective resource and service management.
Explanation:
docker compose
: This is the command that interfaces with Docker Compose.ps
: This subcommand lists the currently running containers within the context of the Docker Compose project defined by your YAML file.
Example Output:
NAME COMMAND STATE PORTS
web "nginx -g 'daemon of…" Up 80/tcp
db "docker-entrypoint.s…" Up 5432/tcp
Use case 2: Create and Start All Containers in the Background
Code:
docker compose up --detach
Motivation:
Starting all containers in the background allows you to continue using the terminal for other tasks. It’s particularly useful when dealing with long-lived services like web servers or databases, where constant interaction through the terminal’s interface isn’t necessary once they’re up and running.
Explanation:
docker compose
: Executes the Docker Compose command.up
: This subcommand starts the containers defined in the YAML file.--detach
: This flag starts the containers in the background (detached mode), allowing the terminal to be freed up for other tasks.
Example Output:
Creating network "example_default" with the default driver
Creating volume "example_data" with default driver
Starting example_db_1 ... done
Starting example_web_1 ... done
Use case 3: Start All Containers, Rebuild if Necessary
Code:
docker compose up --build
Motivation:
Over time, changes might be made to the Docker images or configurations used by your services. When updates occur, it’s essential to rebuild the images to ensure that you’re running the latest versions of your applications. This use case is particularly relevant during development or after changes in the source code or Dockerfile.
Explanation:
docker compose
: Runs the command with Compose.up
: Initiates running of containers.--build
: This flag forces the rebuilding of images before starting the containers to incorporate any changes.
Example Output:
Building web
Step 1/5 : FROM nginx:alpine
...
Step 5/5 : CMD ["nginx", "-g", "daemon off;"]
...
Recreating example_web_1 ... done
Use case 4: Start All Containers with a Specific Project Name
Code:
docker compose -p project_name --file path/to/file up
Motivation:
Using a specific project name or an alternate compose file is beneficial when managing multiple environments or variations of your deployment. It allows isolation between projects, which is handy in testing or deploying different configurations simultaneously.
Explanation:
docker compose
: The base command for Compose operations.-p project_name
: Designates a project name, overriding the default, which is typically the name of the directory.--file path/to/file
: Specifies an alternate Docker Compose file, enabling customized configurations.up
: Starts the specified containers.
Example Output:
Creating network "project_name_default" with the default driver
Creating volume "project_name_data" with default driver
Starting project_name_db_1 ... done
Starting project_name_web_1 ... done
Use case 5: Stop All Running Containers
Code:
docker compose stop
Motivation:
Stopping all running containers is a necessary operational task when you want to temporarily halt services without destroying them. This is useful during maintenance, troubleshooting, or simply powering down for resource conservation without losing data or configuration.
Explanation:
docker compose
: Interacts with Docker Compose.stop
: This subcommand halts all running containers without removing them or their configurations.
Example Output:
Stopping example_web_1 ... done
Stopping example_db_1 ... done
Use case 6: Stop and Remove All Containers, Networks, Images, and Volumes
Code:
docker compose down --rmi all --volumes
Motivation:
Fully stopping and cleaning up a Docker Compose environment is often required when you need to free up resources or start from a clean slate. This is beneficial for continuous integration environments, where each build might want a fresh start.
Explanation:
docker compose
: The command interface for Compose operations.down
: Stops and removes all components defined in the compose file.--rmi all
: Removes all images used by the services in the compose file.--volumes
: Removes all associated volumes, freeing up disk space.
Example Output:
Removing example_web_1 ... done
Removing example_db_1 ... done
Removing network example_default
Removing volume example_data
Use case 7: Follow Logs for All Containers
Code:
docker compose logs --follow
Motivation:
Following logs in real-time is a crucial diagnostic tool that allows administrators and developers to monitor application behavior, capture errors, and gain insights into running services. It’s especially valuable when assessing application performance or diagnosing issues.
Explanation:
docker compose
: The Compose command.logs
: Accesses the output logs of services.--follow
: Continues to stream the logs as they are produced, similar to tailing a log file.
Example Output:
Attaching to example_web_1, example_db_1
web_1 | 172.19.0.1 - - [05/Oct/2023:12:34:56 +0000] "GET / HTTP/1.1" 200 612
db_1 | LOG: database system was shut down at 2023-10-05 12:34:00 UTC
Use case 8: Follow Logs for a Specific Container
Code:
docker compose logs --follow container_name
Motivation:
In a multi-container setup, isolating logs for a specific container can help focus on a service of interest, making monitoring or debugging more efficient. This use case is essential when you’re troubleshooting a single component of your application stack.
Explanation:
docker compose
: Command for Docker Compose.logs
: Retrieves logs.--follow
: Enables real-time log streaming.container_name
: Specifies the name of the container whose logs you want to follow, allowing targeted monitoring.
Example Output:
Attaching to example_web_1
web_1 | 172.19.0.1 - - [05/Oct/2023:12:36:24 +0000] "POST /login HTTP/1.1" 302 -
Conclusion:
The docker compose
command offers a comprehensive suite of capabilities that simplify container orchestration. Whether you’re deploying a single service or managing a complex application stack, these examples demonstrate the versatility and power of Docker Compose, allowing for efficient setup, operation, and maintenance of container environments. By harnessing these commands, developers and operators can achieve greater productivity and more robust application deployment pipelines.