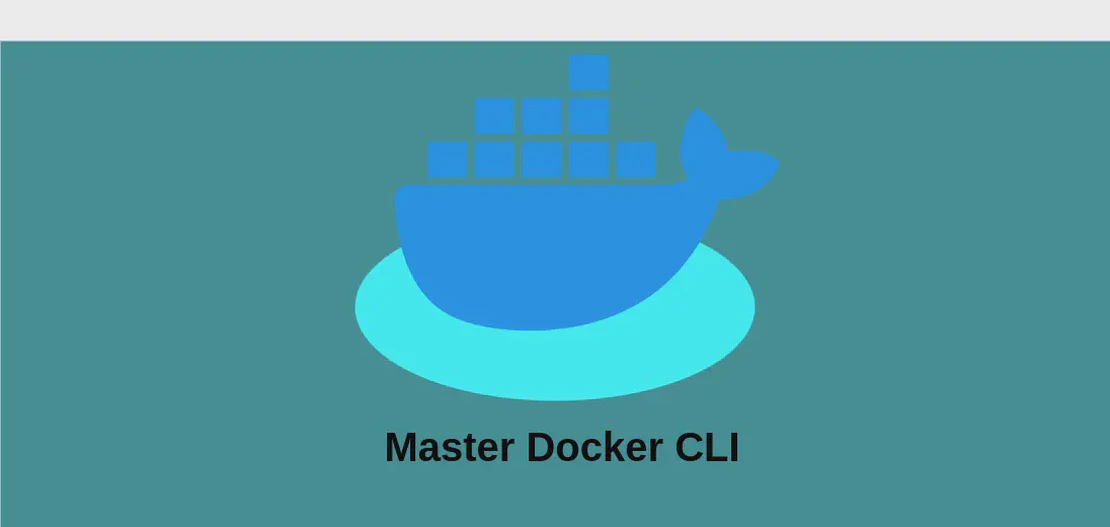
How to use the 'docker container' command (with examples)
Docker is a platform used for developing, shipping, and running applications in a more streamlined and efficient manner. The docker container
command is fundamental in managing the lifecycle of containers, which are isolated environments running your applications. This command allows users to list, start, stop, pause, inspect, export, and commit containers, providing comprehensive control over containerized applications.
Use case 1: List currently running Docker containers
Code:
docker container ls
Motivation:
Listing the currently running containers is essential for monitoring the applications that are actively executing. It provides an overview of the containers’ states, image names, uptime, and resource consumption. This information is crucial for system administrators and developers who need to manage and troubleshoot applications in production or development environments.
Explanation:
docker
: The main Docker command.container
: Specifies that you’re executing a container-related operation.ls
: Abbreviation for “list”, which displays information about running containers.
Example Output:
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
abcd1234efgh nginx:latest "nginx -g 'daemon of…" 5 hours ago Up 5 hours 0.0.0.0:80->80/tcp webserver
ijkl5678mnop mysql:5.7 "docker-entrypoint.s…" 2 days ago Up 2 days 3306/tcp, 33060/tcp db
Use case 2: Start one or more stopped containers
Code:
docker container start container1_name container2_name
Motivation:
Occasionally, containers may need to be stopped for various reasons, such as maintenance or resource preservation. The ability to start stopped containers ensures that services can be quickly resumed, maintaining the continuity of applications without losing previous states or data.
Explanation:
docker
: Invokes Docker capabilities.container
: Indicates container-related actions.start
: Command to initiate the operation of one or more containers.container1_name
,container2_name
: Represents the names or IDs of the containers you wish to start.
Example Output:
container1_name
container2_name
Use case 3: Kill one or more running containers
Code:
docker container kill container_name
Motivation:
Killing a container is sometimes necessary when a container becomes unresponsive or is using excessive resources. This forceful termination ensures problematic applications do not affect the host system or other running containers. This command should be used with caution since it does not allow the container to gracefully shut down.
Explanation:
docker
: Initiates the Docker command interface.container
: Specifies a container-related operation.kill
: Immediately terminates the specified containers.container_name
: The name or ID of the container to be terminated.
Example Output:
container_name
Use case 4: Stop one or more running containers
Code:
docker container stop container_name
Motivation:
Stopping containers is a common task when needing to halt operations temporarily. Unlike killing a container, stopping it allows for a graceful shutdown, which is more appropriate for preserving data integrity and maintaining the container’s current state for future resumption.
Explanation:
docker
: Accesses Docker’s command palette.container
: Indicates operations related to containers.stop
: Gracefully stops the execution of specified containers.container_name
: Denotes the container’s identification needed to perform the operation.
Example Output:
container_name
Use case 5: Pause all processes within one or more containers
Code:
docker container pause container_name
Motivation:
Pausing a container suspends all its processes without stopping or killing the application. This can be especially useful during maintenance tasks on the host system to free up CPU resources temporarily without disrupting the entire service.
Explanation:
docker
: Calls on Docker utilities.container
: Relates to actions targeted at containers.pause
: Freezes all operations within the specified containers.container_name
: The identifier of the container to be paused.
Example Output:
container_name
Use case 6: Display detailed information on one or more containers
Code:
docker container inspect container_name
Motivation:
Inspecting containers gives insights into their configuration, runtime settings, networking details, and resource usage. Developers and system administrators often need such detailed information to troubleshoot issues, optimize performance, and ensure proper configurations.
Explanation:
docker
: Engages with the Docker interface.container
: Operations centered around container management.inspect
: Retrieves detailed configuration and status information for the specified container(s).container_name
: The specific container for which the information is requested.
Example Output:
[
{
"Id": "abcd1234efgh",
"Name": "/webserver",
"Path": "nginx",
...
}
]
Use case 7: Export a container’s filesystem as a tar archive
Code:
docker container export container_name
Motivation:
Exporting a container’s filesystem is beneficial for capturing its entire file structure. This is valuable for backup purposes, distribution, and deploying the same environment across different systems, ensuring consistency in applications without needing to rebuild the entire setup.
Explanation:
docker
: References Docker capabilities.container
: Indicates the operation is related to container execution.export
: Creates a tar archive of the container’s filesystem.container_name
: Specifies the container to be exported.
Example Output:
A tar archive is created and saved to the specified location, not visible directly in the terminal output.
Use case 8: Create a new image from a container’s changes
Code:
docker container commit container_name
Motivation:
Committing a container allows you to save the current state of a container into a new image. This is particularly useful for development environments, where specific changes need to be preserved and replicated in other containers consistently.
Explanation:
docker
: Engages Docker command-line functionality.container
: Pertains to container management operations.commit
: Saves changes in the current container state as a new image.container_name
: The specific container whose current state will be saved as an image.
Example Output:
sha256:1234567890abcdef...
Conclusion:
The docker container
command is an essential tool for managing Docker containers, enabling tasks such as listing, starting, stopping, pausing, inspecting, exporting, and committing containers. Each use case described above highlights a specific scenario where these commands can be effectively applied, underscoring Docker’s flexibility and control in containerized application development and management.