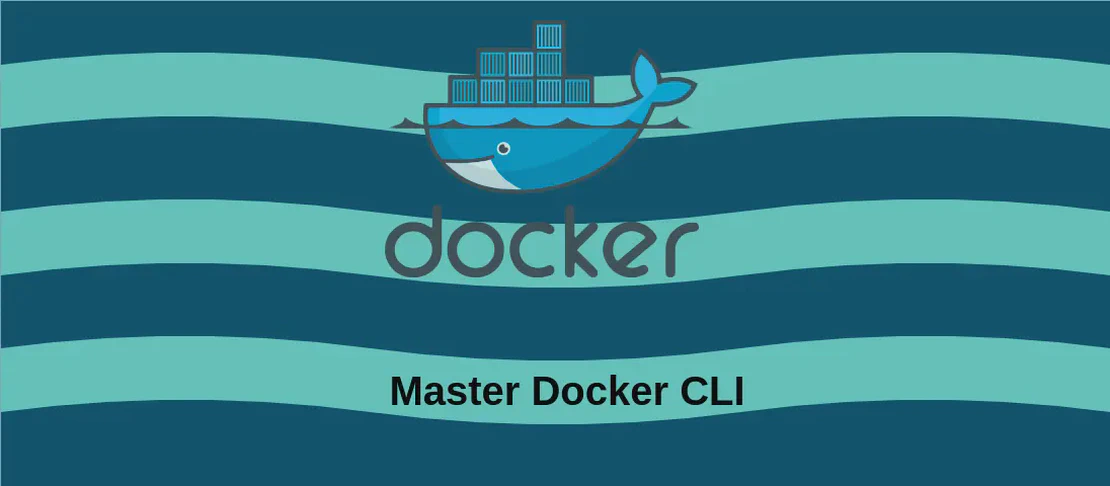
How to Use the Command 'docker inspect' (with Examples)
Docker containers and images have become the cornerstone of modern application deployment. Understanding these, however, demands a tool that can provide granular information. The command docker inspect
serves this purpose by returning low-level details on Docker objects such as containers, images, networks, and volumes. Utilizing docker inspect
efficiently can simplify debugging, enhance security monitoring, and provide insights into containerized environments, making it indispensable for developers and system administrators alike.
Use Case 1: Display Help
Code:
docker inspect
Motivation:
This command offers insights or quick references, especially useful for beginners or those unfamiliar with docker inspect
. In most terminal commands, invoking help shows how to use the command and what options are available. It’s a great starting point for those looking to understand what options can be explored further with docker inspect
.
Explanation:
Running docker inspect
without any additional arguments or flags prompts Docker to display a help message. This is a built-in functionality in most command-line utilities to assist users in understanding the syntax and available options.
Example Output:
Usage: docker inspect [OPTIONS] NAME|ID [NAME|ID...]
Return low-level information on Docker objects
Use Case 2: Display Information About a Container, Image, or Volume Using a Name or ID
Code:
docker inspect container|image|ID
Motivation:
This functionality acts as a catch-all to reveal a comprehensive set of details for any Docker object specified by name or ID. It’s particularly valuable for getting a full-scope view when troubleshooting or auditing resource configurations within a Docker environment.
Explanation:
container|image|ID
: Placeholder for the targeted object. Depending on what you wish to inspect, you replace this with the actual container name, image name, or ID. Docker will then return detailed metadata such as environment variables, mounts, network settings, and more.
Example Output:
[
{
"Id": "e90e34656806",
"Created": "2023-10-12T11:32:18.284156789Z",
"Path": "/bin/sh",
...
}
]
Use Case 3: Display a Container’s IP Address
Code:
docker inspect --format '{{range.NetworkSettings.Networks}}{{.IPAddress}}{{end}}' container
Motivation:
In a networked environment, knowing the IP address of a container is crucial for any form of inter-container communication or external access. This command helps you quickly ascertain the assigned IP address without manually combing through verbose output. It’s especially useful for debugging network-related issues.
Explanation:
--format
: Specifies the template format for output, allowing users to extract specific pieces of information.'{{range.NetworkSettings.Networks}}{{.IPAddress}}{{end}}'
: This Go template accesses the IPAddress property nested within the network settings. Therange
function iterates over networks, displaying their IPs.
Example Output:
172.17.0.2
Use Case 4: Display the Path to the Container’s Log File
Code:
docker inspect --format='{{.LogPath}}' container
Motivation:
Logs are essential for tracing the execution flow, understanding errors, and monitoring containerized applications. Knowing the log file path helps in quickly accessing log data, essential for incident response, monitoring, and audits.
Explanation:
--format='{{.LogPath}}'
: Uses the Go template to directly extract theLogPath
, which is the path to the location where Docker stores logs for the specific container.
Example Output:
/var/lib/docker/containers/e90e34656806/e90e34656806-json.log
Use Case 5: Display the Image Name of the Container
Code:
docker inspect --format='{{.Config.Image}}' container
Motivation:
In environments where numerous containers may run simultaneously, tracking which image each container uses is vital for version control, ensuring consistency, and debugging. This information links runtime containers back to their originating images.
Explanation:
--format='{{.Config.Image}}'
: This formats the output to specifically fetch theImage
property’s value, indicated in the container’s configuration (Config
).
Example Output:
nginx:latest
Use Case 6: Display the Configuration Information as JSON
Code:
docker inspect --format='{{json .Config}}' container
Motivation:
Sometimes you need to review the configuration details structured in a programmatically accessible format. JSON is a widely-used structured data format that supports easy parsing by various programming languages or tools, facilitating automation or data sharing.
Explanation:
--format='{{json .Config}}'
: The command leverages JSON templating to output the configuration (Config
) section of the container’s metadata as a formatted JSON object.
Example Output:
{
"Hostname": "e90e34656806",
"Domainname": "",
...
}
Use Case 7: Display All Port Bindings
Code:
docker inspect --format='{{range $p, $conf := .NetworkSettings.Ports}} {{$p}} -> {{(index $conf 0).HostPort}} {{end}}' container
Motivation:
Understanding port mappings is crucial when diagnosing connectivity issues or setting up firewall rules. Knowing which host ports are forwarded to a container aids in configuring other network components and ensuring secure deployments.
Explanation:
--format='{{range $p, $conf := .NetworkSettings.Ports}} {{$p}} -> {{(index $conf 0).HostPort}} {{end}}'
: This iterates over thePorts
object withinNetworkSettings
, associating container ports with the relevant host ports they’re mapped to.
Example Output:
80/tcp -> 8080
443/tcp -> 8443
Conclusion:
The versatility of docker inspect
empowers developers and system administrators by providing comprehensive visibility into Docker objects, which enhances troubleshooting, performance optimization, and security efforts. By mastering the different ways to use docker inspect
, one can not only gain deep insights but also automate and streamline Docker container management effectively.