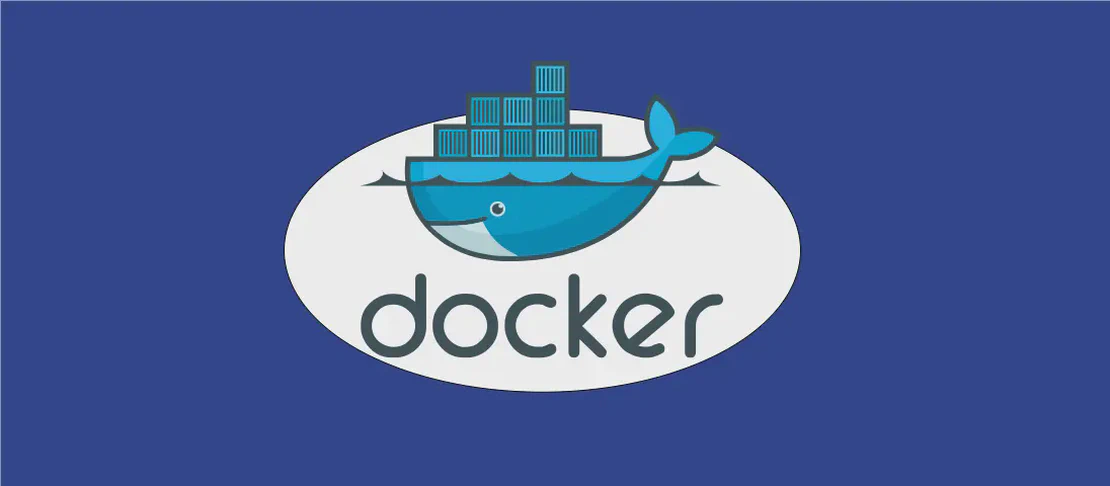
How to use the command `docker login` (with examples)
The docker login
command enables users to securely log into a Docker registry. This allows them to authenticate their access to the registry and interact with its contents. By using this command, users can pull and push images to and from the registry, as well as perform other Docker registry-related actions.
Use case 1: Interactively log into a registry
Code:
docker login
Motivation:
This use case is suitable when a user wants to log into a Docker registry interactively. The command prompts the user for their username and password. This method is both simple and convenient for users who are logging into the registry from the command line.
Explanation:
By executing docker login
without any additional arguments, the user is prompted to enter their username and password interactively. Once the correct credentials are entered, the user gains access to the targeted Docker registry.
Example output:
$ docker login
Username: myusername
Password:
Login Succeeded
Use case 2: Log into a registry with a specific username
Code:
docker login --username username
Motivation:
This use case is suitable when a user wants to specify their username for logging into a Docker registry. By providing the username argument during login, the user can avoid being prompted for their username and instead directly enter their password when requested.
Explanation:
Using the --username
flag, followed by the desired username, allows the user to specify their username for logging into the Docker registry. Upon execution, the command prompts the user to enter their password.
Example output:
$ docker login --username myusername
Password:
Login Succeeded
Use case 3: Log into a registry with a username and password
Code:
docker login --username username --password password server
Motivation:
This use case is suitable when a user wants to log into a Docker registry by providing both their username and password. The user may opt to specify a specific registry server to log into, which is useful when multiple registries are available.
Explanation:
By using the --username
flag, followed by the desired username, and the --password
flag, followed by the corresponding password, the user can log into the Docker registry directly, without being prompted for their credentials. Additionally, the server
argument can be used to specify a registry server (URL) if interacting with a particular registry.
Example output:
$ docker login --username myusername --password mypassword myregistry.com
Login Succeeded
Use case 4: Log into a registry with a password from stdin
Code:
echo "password" | docker login --username username --password-stdin
Motivation:
This use case is suitable when a user wants to provide the password for logging into a Docker registry from standard input (stdin
). This approach is useful when needing to automate the login process without exposing the password in the command history.
Explanation:
By using the echo
command, followed by the password enclosed in quotation marks, and piping it to the docker login
command with the --password-stdin
flag, the user can provide the password for logging into the Docker registry. The --username
flag is used to specify the desired username.
Example output:
$ echo "mypassword" | docker login --username myusername --password-stdin
Login Succeeded
Conclusion:
The docker login
command is a versatile tool for securely logging into Docker registries. By following the provided use cases, users can interactively or programmatically log into registries, specifying their credentials manually or from standard input.