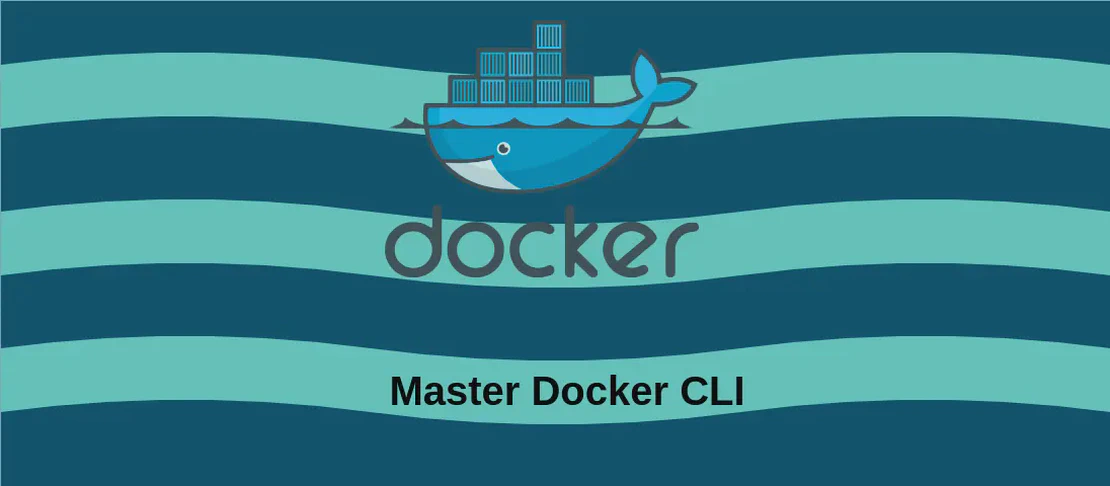
How to manage Docker machines using 'docker-machine' (with examples)
docker-machine
is a command-line tool designed to streamline the process of managing virtual hosts that run Docker. It simplifies the creation, configuration, and administration of Docker environments across different systems or cloud providers, making it easier to manage containerized applications on multiple machines.
Use case 1: List currently running Docker machines
Code:
docker-machine ls
Motivation:
Listing Docker machines is often the first step for developers or system administrators who are managing multiple Docker environments. It provides a snapshot of all the Docker machines configured on your system, allowing you to quickly identify which machines are running, their IP addresses, and their current state. This is particularly useful for managing resources and ensuring that all necessary environments are operational.
Explanation:
The command docker-machine ls
simply lists all the Docker machines currently set up on your host. There are no additional arguments required as this use case is designed to provide an overview of all machines at once.
Example output:
NAME ACTIVE DRIVER STATE URL SWARM DOCKER ERRORS
dev - virtualbox Running tcp://192.168.99.100:2376 v19.03.12
prod * vmware Stopped Unknown
Use case 2: Create a new Docker machine with specific name
Code:
docker-machine create my-machine
Motivation:
Creating a new Docker machine is essential when you want to set up an isolated Docker environment. This can be particularly useful when testing new features, running experiments without disturbing the production environment, or setting up a fresh instance on a new host system. By giving it a specific name, such as my-machine
, it becomes easier to reference and manage the instance later.
Explanation:
The command docker-machine create my-machine
involves using the create
keyword to initialize a new Docker machine. The argument my-machine
specifies the desired name of the new machine being created, making it distinct and identifiable among other machines.
Example output:
Running pre-create checks...
Creating machine...
(my-machine) Creating SSH key...
(my-machine) Creating VirtualBox VM...
(my-machine) Starting the VM...
(my-machine) Waiting for an IP...
Machine "my-machine" was created successfully.
Use case 3: Get the status of a machine
Code:
docker-machine status my-machine
Motivation:
Knowing the status of a Docker machine is fundamental for any administrative tasks, such as when you need to ascertain whether a machine is actively running, stopped, or has encountered any issues. This information helps in decision-making, particularly when prioritizing troubleshooting or when scaling your Docker applications.
Explanation:
The docker-machine status my-machine
command queries the current state of the specified Docker machine my-machine
. By providing the machine’s name, the system directly retrieves and displays its current operational status.
Example output:
Running
Use case 4: Start a machine
Code:
docker-machine start my-machine
Motivation:
Starting a Docker machine is a necessary action when you wish to launch or resume operations on a machine that was previously stopped. This is particularly relevant after maintenance downtime, intentional shutdowns, or before deploying updates. Ensuring a machine is running is a prerequisite for deploying containers or services on it.
Explanation:
The use of the command docker-machine start my-machine
involves the start
keyword, which signals the system to boot up the Docker machine named my-machine
. This action transitions its state from stopped to running.
Example output:
Starting "my-machine"...
(my-machine) Check network to re-create if needed...
(my-machine) Waiting for an IP...
Machine "my-machine" was started successfully.
Use case 5: Stop a machine
Code:
docker-machine stop my-machine
Motivation:
Stopping a Docker machine is crucial when you need to conserve system resources or when the machine is not in use, such as during non-working hours or while performing maintenance. This can also help in reducing costs, particularly in a cloud provider environment where charges might accrue based on active machine time.
Explanation:
The command docker-machine stop my-machine
uses the stop
keyword to cease all operations on the specified Docker machine my-machine
. This switches its state from running to stopped, thereby halting any active containers or services on it.
Example output:
Stopping "my-machine"...
Machine "my-machine" was stopped successfully.
Use case 6: Inspect information about a machine
Code:
docker-machine inspect my-machine
Motivation:
Inspecting a Docker machine provides detailed information about its configuration and current status, such as driver details, resource allocations, and operating conditions. This is useful when troubleshooting issues, planning upgrades, or understanding the environment you’re working with for compliance and security assessments.
Explanation:
The command docker-machine inspect my-machine
leverages the inspect
keyword to retrieve comprehensive details about the specified Docker machine my-machine
. The output is often presented in a JSON format, containing all relevant data pertaining to the machine.
Example output:
{
"DriverName": "virtualbox",
"Driver": {
"CPU": 2,
"Memory": 1024,
...
},
"HostOptions": {
...
}
}
Conclusion:
The docker-machine
command set offers a robust toolkit for managing Docker environments on different hosts efficiently. Whether you’re listing machines, starting or stopping them, or digging deeper into their configurations, understanding each command’s function and purpose ensures effective Docker management and enhances your workflow in containerized application deployment and maintenance.