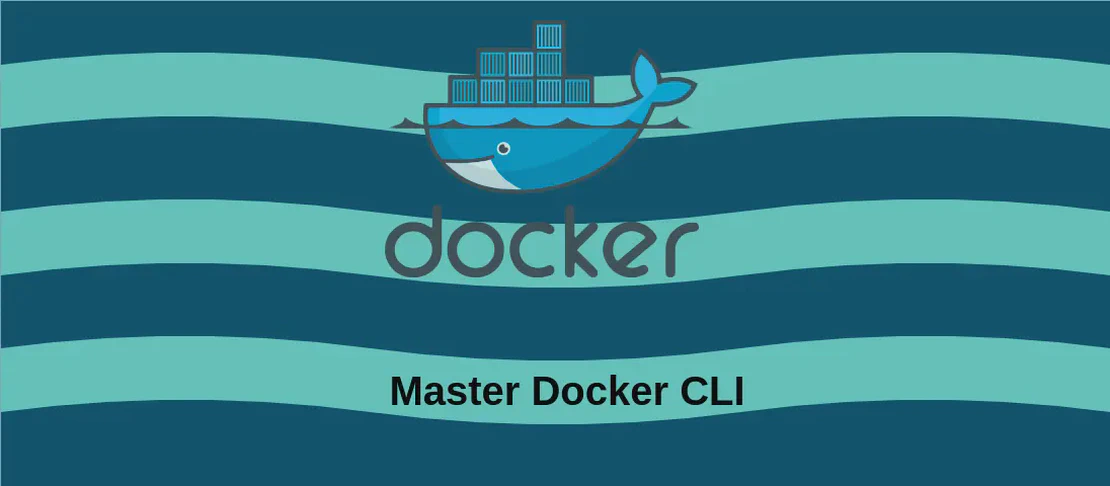
How to use the command 'docker network' (with examples)
Docker networks are a fundamental part of Docker that allow you to define how containers communicate with each other within a Docker host or across multiple Docker hosts. The Docker network command provides a variety of tools to help manage, configure, and control these networks, making containerized applications more modular, scalable, and secure. This comprehensive guide will delve into different use cases of the docker network
command with illustrative examples to demonstrate its functionality and versatility.
Use case 1: Listing all available and configured networks on Docker daemon
Code:
docker network ls
Motivation: Understanding which networks are currently available and active on your Docker daemon is crucial for managing container communication. By listing all networks, you gain insight into the default networks created by Docker, as well as any custom networks you’ve defined, allowing you to troubleshoot, configure, or optimize network settings.
Explanation:
docker
: This is the base command for interacting with Docker components.network
: Specifies that the command related operations are targeted at Docker networks.ls
: Stands for ’list’, which outputs all existing networks on the Docker daemon.
Example Output:
NETWORK ID NAME DRIVER SCOPE
f9f1e2ae3c49 bridge bridge local
450a4c94f27d host host local
4698dfe109f9 none null local
ef3f3ae5b2bb my_custom_network bridge local
This output includes default networks like bridge
, host
, and none
, as well as any user-defined networks such as my_custom_network
.
Use case 2: Creating a user-defined network
Code:
docker network create --driver bridge my_custom_network
Motivation: Creating a user-defined network allows for better isolation and security between containers. It also facilities customized network settings and topology, enabling specific application needs. For instance, user-defined networks can facilitate multi-host networking for distributed applications.
Explanation:
docker network create
: Initiates the creation of a new network.--driver bridge
: Specifies the network driver to be used. Thebridge
driver is commonly used for connecting containers on a single host. Alternatively, you might chooseoverlay
,macvlan
, etc., depending on your requirements.my_custom_network
: The name of the new network being created. This can be any unique name you choose.
Example Output:
82fd5b0c0a3f
This is the unique network ID assigned to your newly created my_custom_network
.
Use case 3: Displaying detailed information about one or more networks
Code:
docker network inspect my_custom_network
Motivation: Inspecting a network provides detailed configuration information such as network details, connected containers, options, and more. This is particularly useful for debugging network issues or verifying that a network is set up correctly as per the specified configuration.
Explanation:
docker network inspect
: Command to retrieve details of specific networks.my_custom_network
: The specific network you are inspecting. You can list multiple network names or IDs separated by space for detailed inspection.
Example Output:
[
{
"Name": "my_custom_network",
"Id": "82fd5b0c0a3f",
"Created": "2023-10-15T12:34:56.789Z",
"Scope": "local",
"Driver": "bridge",
...
"Containers": {},
...
}
]
This JSON output provides all the specific details of my_custom_network
, including network drivers, scope, options, and connected containers.
Use case 4: Connecting a container to a network using a name or ID
Code:
docker network connect my_custom_network my_container
Motivation: By manually connecting a container to a particular network, you ensure that it can communicate with other containers on that same network. This control over container connections is critical for managing inter-container communication, securing data flows, and organizing segment-based deployment strategies.
Explanation:
docker network connect
: Command to connect a container to a specified network.my_custom_network
: The name of the network you want to connect to.my_container
: The name or ID of the container you want to connect.
Example Output: There is no output from this command if it executes successfully. However, a failure might result in an error message indicating the failure reason, such as container or network not found.
Use case 5: Disconnecting a container from a network
Code:
docker network disconnect my_custom_network my_container
Motivation: Disconnecting a container from a network effectively isolates it from communicating with other containers within that network. This can be necessary for security reasons, or when the container no longer needs to participate in the application’s network segment.
Explanation:
docker network disconnect
: Command to disconnect a specific container from a network.my_custom_network
: The network from which the container should be disconnected.my_container
: The container to be disconnected, specified by its name or ID.
Example Output: A successful disconnection will not produce any output, implying seamless execution. If the command fails, an error message is returned, stating why it could not be executed.
Use case 6: Removing all unused (not referenced by any container) networks
Code:
docker network prune
Motivation: As applications evolve, old or unnecessary networks can accumulate, consuming system resources. Periodically pruning unused networks can reduce clutter, optimize performance, and free up network IDs for future use.
Explanation:
docker network prune
: Command to remove all networks that are not being used by any running container. This cleanup command does not require any additional arguments unless utilizing options like-f
for force prune without confirmation.
Example Output:
Deleted Networks:
ef3c6e8b96b5
e93abdb7953b
f9b1a25d7efa
This output lists the network IDs of all networks that were successfully deleted.
Use case 7: Removing one or more unused networks
Code:
docker network rm my_custom_network
Motivation:
Should you need to remove specific networks, perhaps those you have retired or no longer need, you can use this command to do so. It provides a targeted approach compared to prune
, offering more controlled network cleanup.
Explanation:
docker network rm
: This command removes specified networks.my_custom_network
: The name of the network to remove. Multiple network names can be listed to delete several networks at once.
Example Output:
my_custom_network
This confirms that the specified network, my_custom_network
, was successfully removed from the Docker daemon.
Conclusion
Understanding and utilizing Docker networks effectively is instrumental for orchestrating container communications, ensuring security, and maintaining efficient operations. By employing the docker network
command, users can harness powerful tools to manage, inspect, and modify network configurations crucial for any containerized infrastructure.