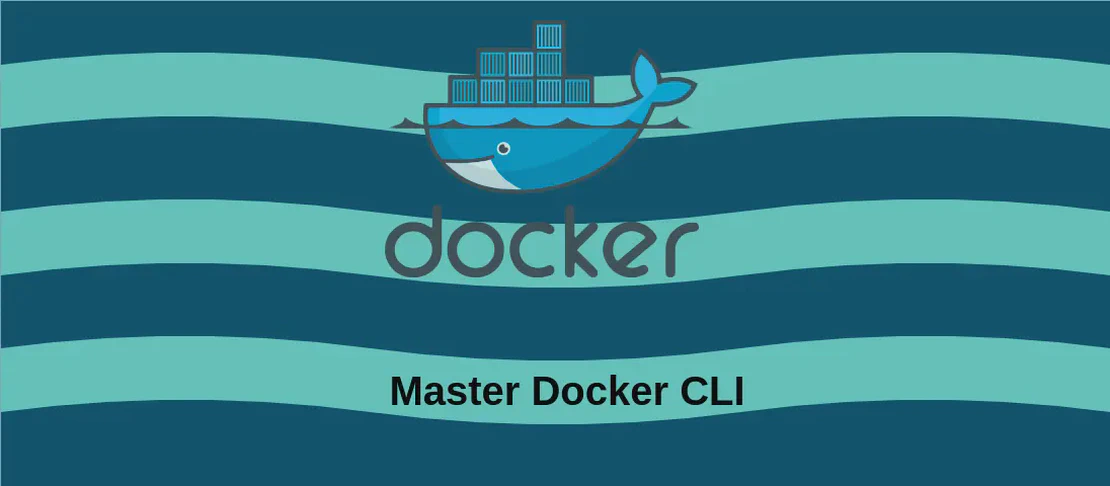
How to Use the Command 'docker ps' (with examples)
The docker ps
command is an essential tool for Docker users, providing insights into the state of Docker containers. It is used to list all the running containers by default. Additionally, with various options, the command can offer detailed information about all containers, filter outputs, and much more. This guide will delve into different use cases of the docker ps
command, demonstrating its versatility and utility in managing Docker environments.
Use Case 1: List currently running Docker containers
Code:
docker ps
Motivation:
This fundamental use case serves to quickly view all containers that are currently running. As a Docker user, knowing which containers are currently active is crucial for managing applications, monitoring workloads, and ensuring that the desired services are operational without interruption.
Explanation:
docker ps
: The command without any additional arguments defaults to showing a list of all running containers. This simplicity makes it an excellent tool for rapid status checks.
Example Output:
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
fa23d2f7b81c nginx:latest "nginx -g 'daemon of…" 2 minutes ago Up 2 minutes 0.0.0.0:80->80/tcp web_server
e678f9ad9c8c redis:alpine "docker-entrypoint.s…" 5 minutes ago Up 5 minutes 6379/tcp redis_cache
Use Case 2: List all Docker containers (running and stopped)
Code:
docker ps --all
Motivation:
In real-world applications, understanding not just what is running but what has also stopped is crucial for debugging, resource management, and tracking container history. This command helps in identifying containers that may have exited due to errors or have been purposely stopped.
Explanation:
--all
or-a
: This flag modifies thedocker ps
command to list all containers, regardless of their current state, whether they’re running, stopped, or exited.
Example Output:
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
fa23d2f7b81c nginx:latest "nginx -g 'daemon of…" 10 minutes ago Exited (0) 2 seconds ago nginx_server
e678f9ad9c8c redis:alpine "redis-server" 15 minutes ago Exited (0) 5 seconds ago redis_server
0d4a25305745 mysql:5.7.32 "docker-entrypoint.s…" 20 minutes ago Up 20 minutes 3306/tcp mysql_db
Use Case 3: Show the latest created container (includes all states)
Code:
docker ps --latest
Motivation:
Quickly identifying the newest container can be essential for workflows where containers are created rapidly, such as in continuous integration and deployment pipelines. Knowing the last created container can speed up troubleshooting and debugging processes.
Explanation:
--latest
or-l
: This flag limits the output to only display the most recently created container, irrespective of its state. It is useful for focusing on the newest work being done within the Docker host.
Example Output:
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
787b79109a7c alpine "echo Hello World" 1 second ago Exited (0) 1 second ago amazing_thompson
Use Case 4: Filter containers that contain a substring in their name
Code:
docker ps --filter "name=name"
Motivation:
In complex environments with numerous containers, finding a specific container by name or identifying a group of containers with similar naming conventions becomes a necessity. This is particularly useful in staged environments (such as dev, test, prod) where container names reflect their role.
Explanation:
--filter
: This option allows users to specify a condition that the listed containers must meet."name=name"
: This specific filter returns all containers with names including the substring “name”.
Example Output:
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
4321bca8bbcd app:latest "start-app.sh" 30 minutes ago Up 30 minutes 0.0.0.0:8080->8080/tcp app_name_server
Use Case 5: Filter containers that share a given image as an ancestor
Code:
docker ps --filter "ancestor=image:tag"
Motivation:
Often, multiple containers are launched from the same image for scaling or testing purposes. This command helps in selectively viewing all such containers, providing a clear perspective of which instances of a particular service or application are currently deployed.
Explanation:
--filter
: Again, this option is used for condition-based filtering."ancestor=image:tag"
: Filters to show containers that have used a specified image as an ancestor, identifying all running instances built from a specific image version.
Example Output:
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
5678f9abcdef ubuntu:18.04 "/bin/bash" 40 seconds ago Up 40 seconds ubuntu_instance
2345abc123f4 ubuntu:18.04 "/bin/sh -c 'sleep 1'" 1 minute ago Exited (0) 30 seconds ago ubuntu_test
Use Case 6: Filter containers by exit status code
Code:
docker ps --all --filter "exited=code"
Motivation:
Knowing which containers have exited with specific error codes is invaluable for debugging and ensuring application reliability. This feature enables quick identification of issues by highlighting containers that have encountered errors during their lifecycle.
Explanation:
--all
: Lists all containers irrespective of their current state.--filter "exited=code"
: Filters containers based on the specified exit status code, such as 0 for success or a non-zero value for failure.
Example Output:
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
5678f9ab127c perl "perl -e 'exit 1;'" 15 minutes ago Exited (1) 14 minutes ago perl_error_case
Use Case 7: Filter containers by status
Code:
docker ps --filter "status=status"
Motivation:
Filtering containers by their status is key in disruption management and operational diagnostics. It allows administrators to quickly separate running and paused containers from those that are in undesired states like exited or dead, facilitating timely interventions.
Explanation:
--filter
: This argument enables condition-based output filtering.status=status
: Shows only those containers that match the specified status such as running, paused, exited, etc.
Example Output:
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
2345bca1d234 node:14 "node server.js" 25 minutes ago Up 25 minutes 0.0.0.0:3000->3000/tcp node_app
Use Case 8: Filter containers that mount a specific volume or have a volume mounted in a specific path
Code:
docker ps --filter "volume=path/to/directory" --format "table .ID\t.Image\t.Names\t.Mounts"
Motivation:
Volume management is critical in Docker environments, especially for persistent storage concerns. By identifying containers using specific volumes, you facilitate resource tracking, setup audits, and help ensure proper data management strategies are being implemented.
Explanation:
--filter "volume=path/to/directory"
: Filters containers that use a particular directory path as a volume.--format "table .ID\t.Image\t.Names\t.Mounts"
: Formats the output in a table displaying container ID, image, name, and the mounted volumes, providing a structured and visually comprehensible summary of relevant containers.
Example Output:
CONTAINER ID IMAGE NAMES MOUNTS
8765acde432b mongo:4.2.6 mongodb /data/db
Conclusion:
This article helped illustrate the versatility and power of the docker ps
command through various practical use cases. Understanding these examples enables Docker users to effectively navigate container management tasks, from simple listings to complex filtering based on names, images, statuses, and volumes. By mastering these commands, users can streamline their Docker operations, troubleshoot efficiently, and manage resources with greater precision.