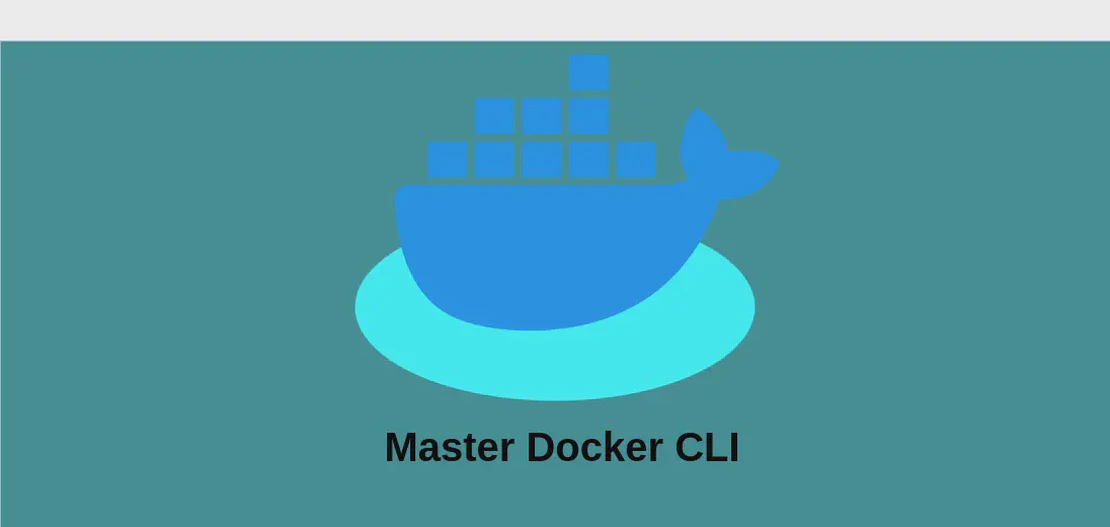
Mastering the Command 'docker run' (with examples)
The docker run
command is a fundamental command within the Docker ecosystem, used to create and start a new container from a specified image. This command is versatile and can be customized with various options to cater to different needs, including running commands interactively, detaching processes, setting environments, and much more. Understanding how to use docker run
effectively allows developers and system administrators to manage Docker containers with greater efficiency and flexibility.
Use case 1: Run command in a new container from a tagged image
Code:
docker run image:tag command
Motivation:
This basic use case is essential for running a specific command within a new Docker container using an image that might have several versions. By specifying the tag, you can ensure you’re deploying the correct version that your application or environment relies on.
Explanation:
image:tag
: Specifies the Docker image to use, whereimage
is the name of the Docker image, andtag
indicates the version of that image.command
: The command you wish to execute inside the newly created container.
Example output:
The application runs within the container, displaying its output in your terminal. If it’s a web application, you might see logs indicating the server has started.
Use case 2: Run command in a new container in background and display its ID
Code:
docker run --detach image command
Motivation:
Running a container in the background is useful when you need processes to operate without interrupting your terminal session. This is common for long-running services like web servers or databases.
Explanation:
--detach
: This option runs the container in the background, allowing the user to regain control of their shell.image
: The name of the Docker image to run.command
: The command to be executed in the background.
Example output:
The terminal displays a unique container ID, confirming it is running in the background. You can use this ID to manage the container with other Docker commands.
Use case 3: Run command in a one-off container in interactive mode and pseudo-TTY
Code:
docker run --rm --interactive --tty image command
Motivation:
Interactive mode is ideal for when you need to troubleshoot or make real-time changes within a container. Combining it with --rm
ensures that the container is automatically removed once you exit.
Explanation:
--rm
: Automatically removes the container when it exits, which helps in managing resources efficiently.--interactive
: Keeps STDIN open, allowing user input within the container.--tty
: Allocates a pseudo-TTY, providing a more user-friendly terminal interface.image
: The specific Docker image you are running.command
: The command to execute, which can involve interactive apps like a shell.
Example output:
You enter an interactive session within the container, seeing a shell prompt or the interactive application’s interface, depending on the specified command.
Use case 4: Run command in a new container with passed environment variables
Code:
docker run --env 'variable=value' --env variable image command
Motivation:
Environment variables are critical for configuring applications during runtime without hardcoding values. This approach allows for easy testing and deployment across different environments by simply changing variables.
Explanation:
--env 'variable=value'
: Sets an environment variable inside the container with a specific value.--env variable
: Enables specifying environment variables, possibly inherited from the host environment.image
: The Docker image to deploy.command
: The command that will run, utilizing the set environment variables.
Example output:
The container uses environment variables during execution, which can affect how the command behaves or configures the application.
Use case 5: Run command in a new container with bind mounted volumes
Code:
docker run --volume /path/to/host_path:/path/to/container_path image command
Motivation:
Using bind mounts is crucial for persisting data or sharing configuration files and other assets between the host and the container. It is ideal for environments where data needs to be retained after container stoppages.
Explanation:
--volume /path/to/host_path:/path/to/container_path
: Maps a directory from the host into the container, allowing bidirectional file system access.image
: Indicates the Docker image to execute.command
: The command that will interact with the provided mounted volumes.
Example output:
Changes made to files within /path/to/container_path
are reflected in the host’s /path/to/host_path
, ensuring persistence beyond the container lifecycle.
Use case 6: Run command in a new container with published ports
Code:
docker run --publish host_port:container_port image command
Motivation:
Publishing ports is essential for network communications between containers and external clients. It’s often used with web servers and database services to allow outside access.
Explanation:
--publish host_port:container_port
: Maps a port on the Docker host to a port within the container, facilitating external access.image
: The Docker image to be run.command
: Executes with the container accessible through the published ports.
Example output:
Network requests to host_port
are forwarded to container_port
, allowing external applications to communicate with the containerized service.
Use case 7: Run command in a new container overwriting the entrypoint of the image
Code:
docker run --entrypoint command image
Motivation:
Overwriting the entrypoint can be necessary when debugging images or executing specific maintenance scripts, as it allows bypassing the default entrypoint set by the Dockerfile.
Explanation:
--entrypoint command
: Overrides the default entrypoint with the specified command.image
: The Docker image to deploy.command
: Represents the new entrypoint, allowing execution of alternative commands on startup.
Example output:
The container starts executing the provided entrypoint command, rather than the default entrypoint set in the image.
Use case 8: Run command in a new container connecting it to a network
Code:
docker run --network network image
Motivation:
Connecting a container to a specific network allows controlled communication between containers. It’s pivotal for managing complex application architectures relying on multiple interconnected services.
Explanation:
--network network
: Specifies the Docker network to which the container will connect, facilitating controlled traffic flow and isolation.image
: The image for the Docker container.command
: Executes with network capabilities defined by the specified Docker network.
Example output:
The container gains access to the specified network, allowing for interactions with other containers or services within the same network segment.
Conclusion:
Understanding the versatile options provided by the docker run
command is crucial for efficient container management and deployment. Each use case presented reflects the wide range of tasks and configurations Docker can handle, making it a powerful tool in the realm of modern software development and IT operations. Whether you’re setting up small-scale applications or designing intricate microservice architectures, mastering docker run
commands paves the way for smoother, more reliable deployments.