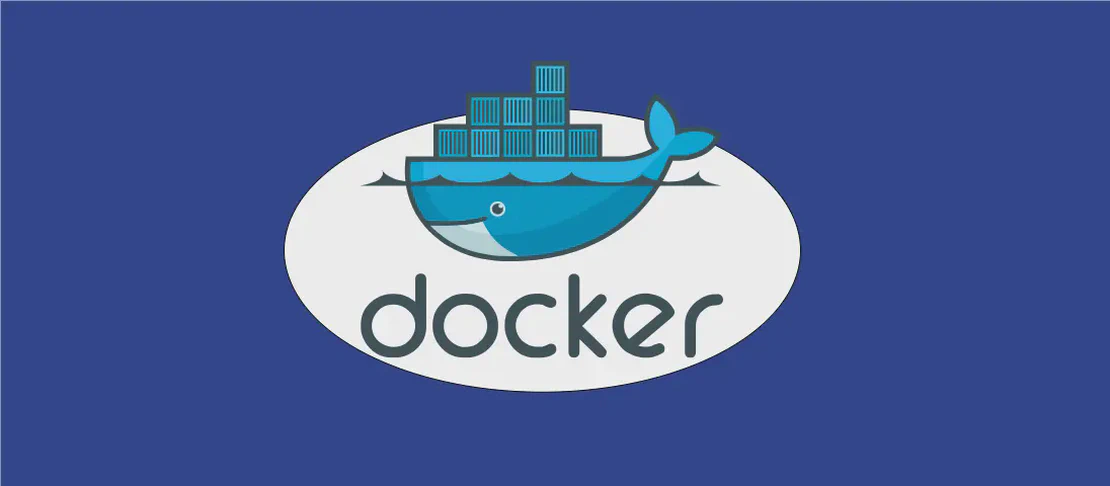
How to use the command 'docker save' (with examples)
The docker save
command is a powerful tool for Docker users who want to export Docker images into tar archive files. This command is invaluable for backing up Docker images, transferring them between different environments, or storing them in a centralized repository without using a registry. By saving Docker images to tar files, you gain the flexibility to easily share and manage your Docker environment.
Save an image by redirecting stdout
to a tar archive
Code:
docker save image:tag > path/to/file.tar
Motivation:
This example demonstrates how to save a Docker image by redirecting the standard output (stdout
) directly to a tar file. It’s useful in scenarios where you want to quickly back up an image or send it over a network stream, and using >
for redirection offers a straightforward approach.
Explanation:
docker save
: This initiates the process of saving a Docker image.image:tag
: Here,image
is the name of the Docker image you wish to save, andtag
specifies the version of the image. Tags help distinguish between different builds or versions of the same image.>
: This is a shell operator that redirects the standard output from thedocker save
command to a file.path/to/file.tar
: This specifies the path and name of the tar archive that will store the image..tar
is a common extension for tar files, which are uncompressed archive files often used in Linux environments.
Example Output:
The tar file is saved to the specified location. You might not receive immediate console feedback, indicating successful execution. You can verify the presence of the tar file using file system commands like ls
.
Save an image to a tar archive
Code:
docker save --output path/to/file.tar image:tag
Motivation:
This use case shows the most direct way to save a Docker image snapshot to a file using the --output
option. This method gives you the flexibility to specify the output file directly within the command, which can, for some users, be more intuitive and explicit than using shell redirection.
Explanation:
docker save
: Initializes saving a Docker image.--output path/to/file.tar
: The--output
flag specifies the destination file where the tar archive will be saved.image:tag
: Specifies which image with its respective tag you want to save.
Example Output:
After execution, the specified file file.tar
will contain the Docker image. It allows for easy verification of the image’s backup by checking the archiving file’s presence.
Save all tags of the image
Code:
docker save --output path/to/file.tar image_name
Motivation:
This example is handy when you need to save all tagged versions of a Docker image repository. It’s especially beneficial in environments where images are frequently updated, and you want a comprehensive backup without losing any tagged versions.
Explanation:
docker save
: Starts exporting the Docker image(s).--output path/to/file.tar
: Indicates where to store the archived images.image_name
: Represents the root name of the image you wish to save. By not specifying a tag, Docker saves all available tags for this image repository to the tar archive.
Example Output:
The tar file could grow larger, as it contains multiple tags of the same image. The result is a multi-version archive that facilitates rollback or sharing of different stages of the Docker image lifecycle.
Cherry-pick particular tags of an image to save
Code:
docker save --output path/to/file.tar image_name:tag1 image_name:tag2 ...
Motivation:
Cherry-picking specific tags is necessary when you need precise backups or to minimize file size, storing only relevant versions of an image. This method provides selective control over which tags are exported, making it highly adaptable for targeted exportations.
Explanation:
docker save
: Commences the task of preserving specified Docker images.--output path/to/file.tar
: Directs where to save the archive.image_name:tag1 image_name:tag2 ...
: These are sequential image and tag pairs. Each pair corresponds to a unique Docker image version you want to preserve in the archive.
Example Output:
The resulting tar archive will be smaller than saving all tags, as it only contains the specified versions. This efficient storage approach provides precise data management tailored to specific needs or space constraints.
Conclusion:
The docker save
command is pivotal for Docker environment management, enabling users to create backups, share images, and maintain version controls effectively. Through various configurations such as output specification, bash redirection, and selective or full-range tagging, Docker proves flexible in accommodating diverse operational needs in managing containerized applications.