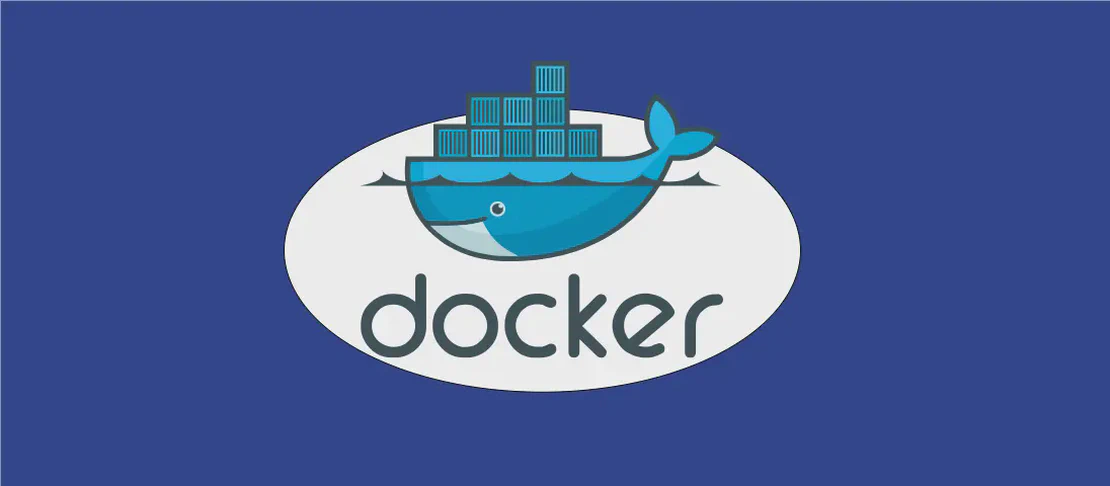
How to manage Docker services using 'docker service' (with examples)
The Docker service
command is an essential tool for managing services on a Docker daemon. It allows users to create, list, inspect, scale, and remove services, enabling efficient orchestration of containerized applications in a swarm environment. These functionalities are crucial for deploying and maintaining applications at scale. The command is particularly useful for developers and system administrators who need to manage distributed applications efficiently.
Use case 1: List the services on a Docker daemon
Code:
docker service ls
Motivation:
Listing services is a fundamental task in Docker’s management toolset. It allows administrators and developers to get an overview of the currently running services in their Docker Swarm. This is particularly useful for troubleshooting, ensuring all required services are running, and gaining insight into the state of your deployments.
Explanation:
docker
: This is the Docker command-line interface.service
: It specifies that the operation pertains to Docker services.ls
: This argument lists all the services currently running on the Docker daemon.
Example Output:
ID NAME MODE REPLICAS IMAGE PORTS
x2z9z4z5t1fw web replicated 3/3 nginx:latest *:80->80/tcp
y7p5z9z5t5yz api replicated 2/2 api-service:latest *:8080->80/tcp
Use case 2: Create a new service
Code:
docker service create --name web_service nginx:latest
Motivation:
Creating a new service is a critical action in deploying containerized applications. This command allows you to set up a new instance of a containerized application, specifying its name, the image to use, and additional parameters if desired. It’s the starting point for running an application within a Docker Swarm.
Explanation:
docker
: Invokes the Docker command-line tool.service
: Indicates that a service-related task is being performed.create
: Initiates the creation of a new service.--name web_service
: Assigns the human-readable nameweb_service
to the new service.nginx:latest
: Specifies the Docker image and tag to use, in this case, the latest version of the Nginx image.
Example Output:
udxyvynvfete1ra7eqwf65tzu
Use case 3: Display detailed information about one or more services
Code:
docker service inspect web_service
Motivation:
Understanding the details of a service is imperative for debugging and effective management. The inspect
command provides comprehensive details about a service, including settings, assigned tasks, and current status. It’s helpful for gaining in-depth insights and verifying configurations.
Explanation:
docker
: Calls the Docker command utility.service
: Specifies that the service commands are being invoked.inspect
: Indicates you want to view detailed information.web_service
: The name or ID of the service you want to inspect.
Example Output:
[
{
"ID": "udxyvynvfete1ra7eqwf65tzu",
"Version": {
"Index": 51
},
"CreatedAt": "2023-08-01T23:36:28.270896182Z",
"UpdatedAt": "2023-08-01T23:36:30.326888945Z",
"Spec": {
"Name": "web_service",
...
}
}
]
Use case 4: List the tasks of one or more services
Code:
docker service ps web_service
Motivation:
Monitoring tasks provides insights into how work progresses across the swarm. It helps in assessing task distribution, rebalancing, or determining if scaling actions were successful. This information aids in performance tuning and resource allocation.
Explanation:
docker
: The command to access Docker’s command-line interface.service
: These commands specifically target services.ps
: Lists the tasks of the specified service.web_service
: The service name for which you want to list tasks.
Example Output:
ID NAME IMAGE NODE DESIRED STATE CURRENT STATE ERROR PORTS
tx1cabcwi9c9 web_service.1 nginx:latest node-1 Running Running 9 minutes ago
tanfovansnw7 web_service.2 nginx:latest node-2 Running Running 9 minutes ago
Use case 5: Scale to a specific number of replicas for a service
Code:
docker service scale web_service=5
Motivation:
Scaling services according to demand is essential for meeting user requirements while optimizing resource utilization. Adjusting replicas helps handle varying workloads and ensures high availability, making sure services remain reliable even under heavy traffic.
Explanation:
docker
: Initiates the Docker interface.service
: Directs the commands to service-level actions.scale
: Specifies the desire to adjust the number of replicas.web_service=5
: Targets the specified service (web_service
) and sets the number of replicas to 5.
Example Output:
web_service scaled to 5
overall progress: 5 out of 5 tasks
Use case 6: Remove one or more services
Code:
docker service rm web_service
Motivation:
Effectively removing services when they are no longer needed or when cleaning up unused resources is critical for maintaining an efficient Docker environment. This helps free system resources and keeps the swarm environment uncluttered.
Explanation:
docker
: Accesses the Docker command-line interface.service
: Pertains to services within Docker.rm
: Removes the specified service(s).web_service
: The service name you intend to remove.
Example Output:
web_service
Conclusion
The docker service
command embodies a set of powerful tools that simplify and enhance the management of Docker services within a swarm. From creating new services to scaling existing ones and removing services that are no longer needed, each use case demonstrates essential tasks for orchestrating distributed applications. By mastering these commands, developers and system administrators can effectively manage their containerized applications and maintain robust, scalable infrastructures.