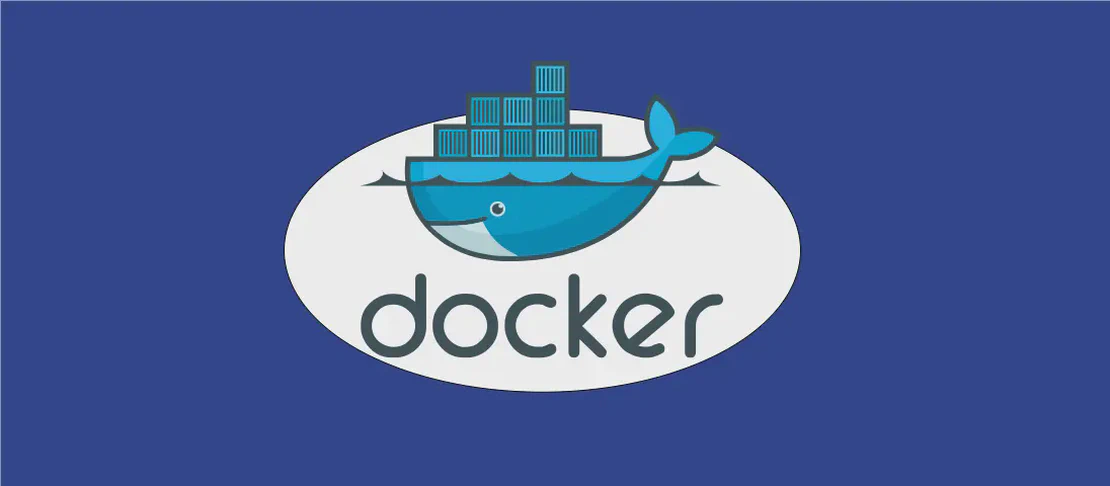
How to use the command 'docker update' (with examples)
The ‘docker update’ command is used to update the configuration of Docker containers. It allows you to modify various container-specific configurations such as the restart policy, CPU count, memory limit, process ID limit, and memory swap limit.
Use case 1: Update restart policy to apply when a specific container exits
Code:
docker update --restart=always container_name
Motivation: The ‘docker update’ command with the ‘–restart’ flag is useful when you want a container to automatically restart whenever it exits. In this example, the ‘always’ restart policy is applied to the ‘container_name’, which means Docker will automatically restart the container regardless of its exit status.
Explanation: The ‘–restart’ flag specifies the restart policy for the container. The available restart policies are ‘always’, ’no’, ‘on-failure’, and ‘unless-stopped’. In this case, the ‘always’ restart policy is used. The ‘container_name’ argument is the name or ID of the specific container you want to update.
Example output: The container ‘container_name’ will now automatically restart whenever it exits.
Use case 2: Update the policy to restart up to three times a specific container when it exits with non-zero exit status
Code:
docker update --restart=on-failure:3 container_name
Motivation: In case a specific container frequently fails, you may want to limit the number of restart attempts Docker makes. This command allows you to control the container’s restart behavior and set a threshold for the number of restart attempts.
Explanation: The ‘–restart’ flag is used again to specify the restart policy, but this time it is set to ‘on-failure:3’, which means Docker will restart the container up to three times if it exits with a non-zero exit status. The ‘container_name’ argument identifies the container to update.
Example output: If the container ‘container_name’ exits with a non-zero exit status, Docker will attempt to restart it up to three times.
Use case 3: Update the number of CPUs available to a specific container
Code:
docker update --cpus 2 container_name
Motivation: Sometimes, a container may require more CPU resources to run efficiently. With this command, you can allocate a specific number of CPUs to a container, allowing it to utilize more computational power.
Explanation: The ‘–cpus’ flag is used to specify the number of CPUs to allocate to the container. In this example, the value ‘2’ indicates that two CPUs will be assigned to the ‘container_name’.
Example output: The container ‘container_name’ will be able to utilize two CPUs for improved performance.
Use case 4: Update the memory limit in [M]egabytes for a specific container
Code:
docker update --memory 512M container_name
Motivation: By limiting the memory usage of a specific container, you can prevent it from consuming excessive resources and affecting the performance of other containers or the host system.
Explanation: The ‘–memory’ flag is used to set the memory limit for the container. In this example, ‘512M’ is the specified memory limit in megabytes for the ‘container_name’.
Example output: The container ‘container_name’ will be constrained to using a maximum of 512 megabytes of memory.
Use case 5: Update the maximum number of process IDs allowed inside a specific container
Code:
docker update --pids-limit 100 container_name
Motivation: Limiting the number of process IDs within a container can help prevent malicious or runaway processes from consuming excessive resources or affecting the stability of the host system.
Explanation: The ‘–pids-limit’ flag sets the maximum number of process IDs allowed within the container. In this example, ‘100’ is the specified limit for the ‘container_name’. If you want to allow an unlimited number of process IDs, you can use ‘-1’ instead.
Example output: The container ‘container_name’ will be restricted to a maximum of 100 process IDs.
Use case 6: Update the amount of memory in [M]egabytes a specific container can swap to disk
Code:
docker update --memory-swap 1024M container_name
Motivation: Swapping allows a container to use disk space as an extension of physical memory. By updating the memory swap limit, you can control the amount of memory a container can swap to disk.
Explanation: The ‘–memory-swap’ flag is used to set the memory swap limit for the container. In this example, ‘1024M’ specifies the limit in megabytes for the ‘container_name’. To allow unlimited memory swapping, you can use ‘-1’ as the limit.
Example output: The container ‘container_name’ will have a memory swap limit of 1024 megabytes.
Conclusion:
The ‘docker update’ command provides convenient ways to modify the configuration of Docker containers. Whether you need to change the restart policy, allocate more CPUs or memory, or set resource limits, this command allows you to manage container-specific configurations effectively. By understanding how to use each of the provided use cases, you can optimize the behavior and resource utilization of your Docker containers.