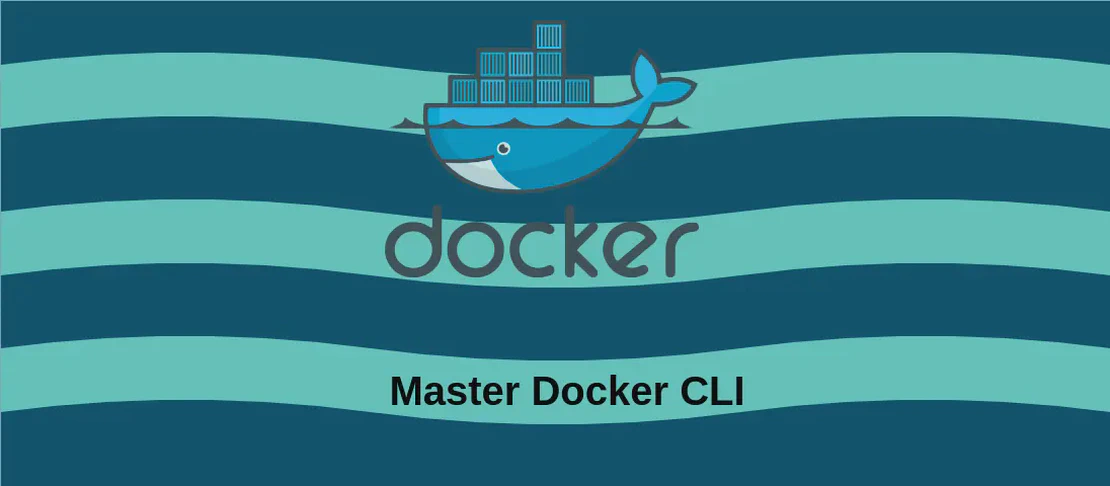
Using Docker Volume Command (with examples)
The Docker volume command is an essential part of Docker’s suite of tools that allows users to manage volumes within their Docker environment. Volumes are a critical component of Docker as they enable persistent data storage, making it possible to retain data even after containers are terminated or recreated. This command provides functionalities for creating, listing, inspecting, and removing volumes, among other operations. Below are various use cases of this command with detailed explanations.
Use case 1: Create a volume
Code:
docker volume create volume_name
Motivation: Creating a volume is the foundational step in managing data persistence in Docker. By establishing a volume, you secure a storage space that can be attached to one or more containers. This is particularly useful in scenarios where data needs to be shared or preserved across container restarts or between different containers.
Explanation:
docker volume
: This initiates the Docker volume command utility.create
: This subcommand instructs Docker to create a new volume.volume_name
: This is the name you assign to the volume for identification.
Example output:
volume_name
This output confirms that a new volume with the specified name has been successfully created.
Use case 2: Create a volume with a specific label
Code:
docker volume create --label label volume_name
Motivation: Assigning a label to a volume aids in organizing, identifying, and managing volumes, especially when dealing with complex environments that include numerous volumes. Labels are key-value pairs that add metadata to Docker entities, and they are instrumental in automating workflows and maintaining comprehensive documentation of resources.
Explanation:
docker volume create
: Begins the creation of a new volume.--label label
: This option allows you to assign a specific label to the volume.label
here is a placeholder for the actual key-value pair you intend to use, such as--label environment=production
.volume_name
: The designated name for the new volume.
Example output:
volume_name
This indicates that the volume with the specified label and name has been successfully created.
Use case 3: Create a tmpfs
volume with a size of 100 MiB and a uid of 1000
Code:
docker volume create --opt type=tmpfs --opt device=tmpfs --opt o=size=100m,uid=1000 volume_name
Motivation: Creating a tmpfs volume is valuable for scenarios that require high-speed storage with data that doesn’t need to persist beyond container lifecycle, as tmpfs stores data in memory. Specifying options like size and UID ensures that the volume meets specific resource and security requirements.
Explanation:
docker volume create
: Command to create a new volume.--opt type=tmpfs
: Specifies that the volume type is tmpfs, indicating in-memory storage.--opt device=tmpfs
: Denotes the device used for tmpfs storage.--opt o=size=100m,uid=1000
: Sets additional options, specifying a size of 100 MiB and a user ID of 1000, which dictates who owns the volume.volume_name
: The name assigned to this configuration of the volume.
Example output:
volume_name
This confirms that a tmpfs volume with the specified options has been successfully created.
Use case 4: List all volumes
Code:
docker volume ls
Motivation: Listing volumes is crucial for gaining insights into the storage resources available in your Docker environment. It provides visibility into what volumes exist, their usage, and helps in maintenance and debugging tasks.
Explanation:
docker volume ls
: This command lists all existing volumes, showing information such as names and driver types.
Example output:
DRIVER VOLUME NAME
local volume_name1
local volume_name2
The output lists each volume’s driver type and name, allowing you to verify and manage stored data effectively.
Use case 5: Remove a volume
Code:
docker volume rm volume_name
Motivation: Removing a volume is essential when you need to free unused resources or when a volume is no longer necessary. It helps in efficient resource management and in preventing clutter in your Docker environment.
Explanation:
docker volume rm
: This deletes a specified volume.volume_name
: The name of the volume you wish to remove.
Example output:
volume_name
This output indicates that the specified volume has been successfully deleted.
Use case 6: Display information about a volume
Code:
docker volume inspect volume_name
Motivation: Inspecting a volume is vital for understanding its configuration, such as details about the mount point, driver, and usage. This information assists in debugging, auditing, and compliance tasks.
Explanation:
docker volume inspect
: Initiates the inspection of a specified volume, detailing its configuration and properties.volume_name
: The name of the volume you want to inspect.
Example output:
[
{
"CreatedAt": "2023-10-01T12:34:56Z",
"Driver": "local",
"Labels": null,
"Mountpoint": "/var/lib/docker/volumes/volume_name/_data",
"Name": "volume_name",
"Options": {},
"Scope": "local"
}
]
The detailed JSON output provides comprehensive information about the volume.
Use case 7: Remove all unused local volumes
Code:
docker volume prune
Motivation: Pruning unused volumes is a key maintenance task that helps reclaim storage space. It is especially important in environments where storage is a limited resource or where there is a need to frequently clean up unnecessary data.
Explanation:
docker volume prune
: Triggers the removal of all unused local volumes.
Example output:
Deleted Volumes:
volume_name1
volume_name2
Total reclaimed space: 123.4MB
This confirms that the unused volumes have been successfully removed and reclaimed space is reported.
Use case 8: Display help for a subcommand
Code:
docker volume subcommand --help
Motivation: Accessing help for a subcommand is crucial for understanding how to use various volume management operations. It provides descriptions, options, and examples that can simplify command formulation and usage.
Explanation:
docker volume
: Refers to the primary command.subcommand --help
: Replacesubcommand
with any specific subcommand (e.g., create, rm) to view its help information.
Example output:
Usage: docker volume <subcommand> [OPTIONS]
Manage Docker volumes
Options:
--help Print usage
The help output provides guidance on how to use the specific subcommand and its options.
Conclusion:
The Docker volume command provides a versatile toolkit for managing persistent data storage within a containerized environment. Understanding and effectively utilizing each of its use cases can significantly enhance data management, improve resource utilization, and streamline workflows in Docker.