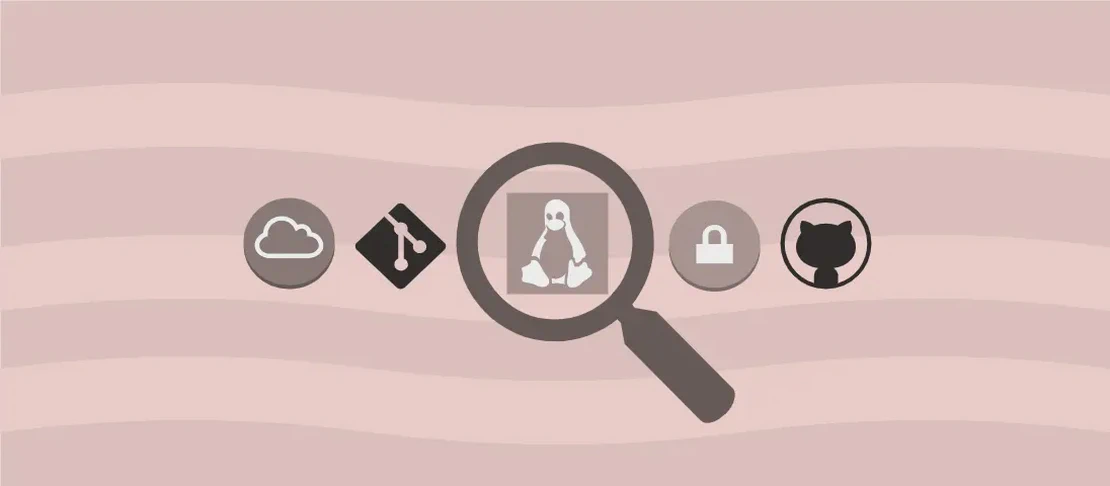
How to Use the Command 'dotnet add package' (with examples)
The dotnet add package
command is part of the .NET CLI (Command-Line Interface) tools that facilitate the management of .NET project dependencies. Specifically, this command allows developers to add or update package references in a .NET project file, enhancing the project’s functionality by utilizing various libraries available in NuGet, which is the package manager for .NET. By using this command, developers can efficiently include a variety of libraries, tools, and frameworks in their projects, thereby harnessing the power of open-source or proprietary code packages maintained by the community or specialized vendors.
Use case 1: Add a package to the project in the current directory
Code:
dotnet add package package
Motivation:
When working on a .NET project, developers often need to incorporate additional functionalities provided by external packages. This command allows for the seamless integration of such a package into the project located in the current directory. By adding a package, you can extend the capabilities of your application, save development time, and avoid reinventing the wheel.
Explanation:
dotnet add package
: The core command used to add a package.package
: Represents the name of the package you wish to add.
Example Output:
After executing the command, the console confirms the addition of the package with output similar to:
info : Adding PackageReference for package 'PACKAGE_NAME' into project 'PROJECT_NAME'.
Use case 2: Add a package to a specific project
Code:
dotnet add path/to/file.csproj package package
Motivation:
In scenarios where a solution contains multiple projects, you might want to add a package to a specific project rather than the one in the current directory. This command gives precise control over which project gets the new package, ensuring that only the necessary projects have the required dependencies.
Explanation:
dotnet add
: Initiates the addition process.path/to/file.csproj
: Specifies the file path to the project file (.csproj) where you want the package to be added.package
: The name of the package to include in the project.
Example Output:
info : Adding PackageReference for package 'PACKAGE_NAME' into project 'path/to/file.csproj'.
Use case 3: Add a specific version of a package to the project
Code:
dotnet add package package --version 1.0.0
Motivation:
Software projects often require specific versions of a package to ensure compatibility or to leverage particular features introduced in a stable release. Specifying the version ensures that the project builds and behaves as expected across different environments and developers.
Explanation:
dotnet add package
: Begins the process of adding a package.package
: Indicates the package name.--version 1.0.0
: A flag that specifies the exact version of the package to be added.
Example Output:
info : Adding PackageReference for package 'PACKAGE_NAME' version '1.0.0' into project 'PROJECT_NAME'.
Use case 4: Add a package using a specific NuGet source
Code:
dotnet add package package --source https://api.nuget.org/v3/index.json
Motivation:
At times, packages might be hosted on different NuGet feeds or custom sources. Using a specific source ensures that you are downloading and installing the package from the correct repository, providing a layer of security and authenticity to the dependencies being added.
Explanation:
dotnet add package
: Executes the addition of the package.package
: The desired package name.--source https://api.nuget.org/v3/index.json
: Specifies the NuGet source URL where the package can be found.
Example Output:
info : Adding PackageReference for package 'PACKAGE_NAME' from source 'https://api.nuget.org/v3/index.json' into project 'PROJECT_NAME'.
Use case 5: Add a package only when targeting a specific framework
Code:
dotnet add package package --framework net7.0
Motivation:
Different frameworks might require different packages for optimal performance or compatibility. Adding a package conditionally, based on the framework, ensures that the correct dependencies are in place according to the target framework’s specifications.
Explanation:
dotnet add package
: Begins adding the package.package
: The package name you wish to add.--framework net7.0
: A flag specifying that the package should only be added when targeting .NET framework version 7.0.
Example Output:
info : Adding PackageReference for package 'PACKAGE_NAME' for framework 'net7.0' into project 'PROJECT_NAME'.
Use case 6: Add and specify the directory where to restore packages
Code:
dotnet add package package --package-directory path/to/directory
Motivation:
Customizing the directory where packages are restored can be crucial for specific project needs, such as disk space management, project organization, or adhering to a particular directory structure required by the development environment.
Explanation:
dotnet add package
: Initializes the process to add a package.package
: Refers to the name of the package.--package-directory path/to/directory
: Specifies the path to store retrieved package files, overriding the default path.
Example Output:
info : Adding PackageReference for package 'PACKAGE_NAME' with package directory 'path/to/directory' into project 'PROJECT_NAME'.
Conclusion:
The dotnet add package
command streamlines the process of managing dependencies within .NET projects. Each use case demonstrates the versatility of this command, enabling developers to handle packages in a manner that best suits their project’s specific needs and constraints. Whether managing package versions, targeting specific frameworks, or organizing custom directory structures, this command plays a pivotal role in modern .NET development workflows.