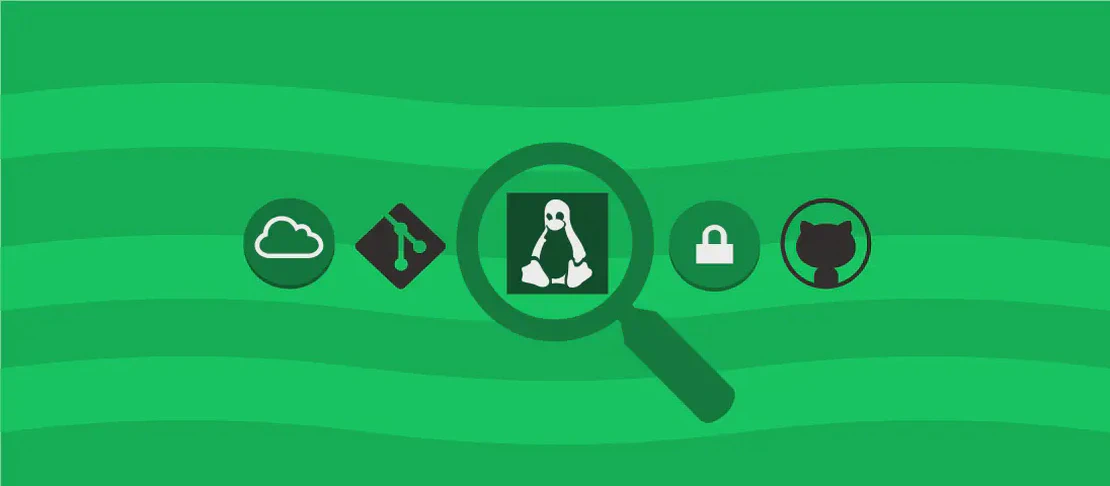
How to use the command 'dotnet add reference' (with examples)
The dotnet add reference
command is a valuable tool in the .NET developer’s toolkit, allowing developers to establish project-to-project dependencies effectively. By adding references between projects, you can manage and share code across different parts of an application or even between multiple applications. This capability ensures code reuse, cleaner project management, and streamlined collaboration across development teams. The command is versatile and can handle both single and multiple project references with ease.
Use case 1: Add a reference to the project in the current directory
Code:
dotnet add reference path/to/reference.csproj
Motivation:
When developing a modular application, you often need to connect your main project with specific library projects that contain shared functionality. By adding a project-to-project reference, you ensure that changes in the library are correctly reflected in the main project’s build process without duplicating code across projects.
Explanation:
dotnet add reference
: Initiates the command to add a reference to the current project.path/to/reference.csproj
: This argument specifies the file path to the .csproj file of the project you wish to reference. It tells the command which library project or module the current project should depend on.
Example Output:
Reference `path/to/reference.csproj` added to the project.
Use case 2: Add multiple references to the project in the current directory
Code:
dotnet add reference path/to/reference1.csproj path/to/reference2.csproj ...
Motivation:
In more complex applications, your main project might rely on multiple discrete modules or libraries. Adding multiple references at once streamlines the setup process, saving time and reducing the risk of errors that might occur if adding them individually.
Explanation:
dotnet add reference
: Utilizes the command to add references to the current project.path/to/reference1.csproj
,path/to/reference2.csproj
,...
: Each of these paths points to a different project file that the current project will reference. By providing multiple paths, all these dependencies can be linked in a single command execution.
Example Output:
Reference `path/to/reference1.csproj`, `path/to/reference2.csproj` added to the project.
Use case 3: Add a reference to the specific project
Code:
dotnet add path/to/project.csproj reference path/to/reference.csproj
Motivation:
This is particularly useful in scenarios where you may be managing multiple projects within the same solution but want to specify which particular project should get the new reference. This fine-grained control is essential when working on large solutions with numerous projects.
Explanation:
dotnet add
: Begins the addition process of a reference.path/to/project.csproj
: Specifies the project file to which you wish to add another project as a reference. It serves as the target project.reference
: This keyword tells the tool that a reference is to be added.path/to/reference.csproj
: Indicates the source project file that will become a dependency for the target project.
Example Output:
Reference `path/to/reference.csproj` added to project `path/to/project.csproj`.
Use case 4: Add multiple references to the specific project
Code:
dotnet add path/to/project.csproj reference path/to/reference1.csproj path/to/reference2.csproj ...
Motivation:
This command is helpful when you need to bulk add multiple project references to a particular target project, which might happen often in large-scale applications where shared libraries are extensively used.
Explanation:
dotnet add
: Command for starting the addition of project references.path/to/project.csproj
: Determines the target project for new references being added.reference
: Marks the beginning of the reference addition segment within the command.path/to/reference1.csproj
,path/to/reference2.csproj
,...
: Lists the projects to be added as dependencies to the target project. This allows for multiple dependencies to be managed simultaneously.
Example Output:
Reference `path/to/reference1.csproj`, `path/to/reference2.csproj` added to project `path/to/project.csproj`.
Conclusion:
The dotnet add reference
command is integral in managing project dependencies across .NET applications. Whether you are working with a simple project or an extensive solution comprising several projects, mastering this command ensures efficient management and development workflows. By understanding the use cases and applying them as needed, you can leverage shared code more effectively, save time, and consistently maintain your projects’ architecture.