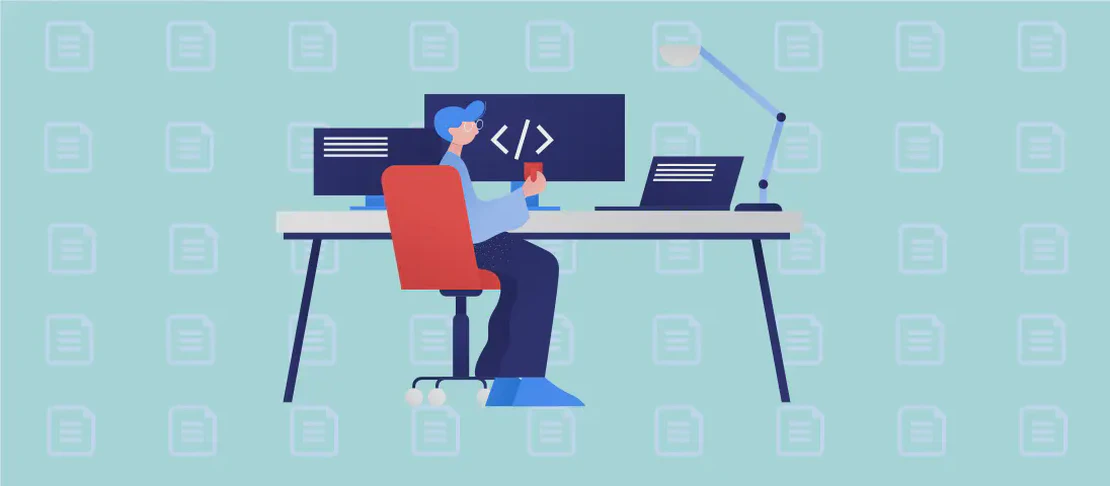
Understanding the 'dotnet build' Command (with examples)
The dotnet build
command is an essential tool in the .NET ecosystem, facilitating the compilation of .NET applications and their dependencies. It plays a critical role by converting source code into runnable applications, bundling required dependencies and resources. Utilizing this command, developers can compile projects in various configurations and environments, streamlining the software development process.
Use case 1: Compile the project or solution in the current directory
Code:
dotnet build
Motivation:
This is the most straightforward use case of the dotnet build
command, where you simply wish to compile the default project or solution in the current directory. By doing so, developers can quickly compile their code without specifying a path, facilitating rapid iteration and testing.
Explanation:
dotnet build
: This command initiates the build process for any .NET project or solution file located in the current directory from which the command is executed. It automatically identifies the project file and compiles it, handling dependencies as indicated in the project configuration.
Example Output:
Microsoft (R) Build Engine version 16.7.0+7fb82e5b2 for .NET
Copyright (C) Microsoft Corporation. All rights reserved.
Determining projects to restore...
Restored C:\Projects\MyApp\MyApp.csproj (in 150 ms).
MyApp -> C:\Projects\MyApp\bin\Debug\net5.0\MyApp.dll
Build succeeded.
0 Warning(s)
0 Error(s)
Time Elapsed 00:00:03.45
Use case 2: Compile a .NET project or solution in a specific path
Code:
dotnet build path/to/project_or_solution
Motivation:
In larger repositories with multiple projects or when working outside the project’s root directory, specifying a path is necessary. It allows developers to target and build specific projects or solutions, aiding in scenarios when not all parts of the solution need to be compiled.
Explanation:
path/to/project_or_solution
: This argument directs thedotnet build
command to the exact location of the project or solution file you wish to compile. This flexibility is crucial for developers working in environments with multiple projects or shared codebases.
Example Output:
Microsoft (R) Build Engine version 16.7.0+7fb82e5b2 for .NET
Copyright (C) Microsoft Corporation. All rights reserved.
Determining projects to restore...
Restored C:\OtherProjects\AnotherApp\AnotherApp.csproj (in 180 ms).
AnotherApp -> C:\OtherProjects\AnotherApp\bin\Debug\net5.0\AnotherApp.dll
Build succeeded.
0 Warning(s)
0 Error(s)
Time Elapsed 00:00:02.90
Use case 3: Compile in release mode
Code:
dotnet build --configuration Release
Motivation:
When preparing an application for production, a ‘Release’ build configuration is typically used. This configuration often includes optimizations for performance, enabling you to deliver a more efficient application ready for deployment.
Explanation:
--configuration Release
: This option tells thedotnet build
command to compile the project using the ‘Release’ configuration. This setup disables debugging information, optimizes output, and is intended for use in production environments, contrasting with the default ‘Debug’ mode used during development.
Example Output:
Microsoft (R) Build Engine version 16.7.0+7fb82e5b2 for .NET
Copyright (C) Microsoft Corporation. All rights reserved.
MyApp -> C:\Projects\MyApp\bin\Release\net5.0\MyApp.dll
Build succeeded.
0 Warning(s)
0 Error(s)
Time Elapsed 00:00:02.03
Use case 4: Compile without restoring dependencies
Code:
dotnet build --no-restore
Motivation:
In situations where dependencies have already been restored, or to save time and bypass redundant operations, the --no-restore
argument can be utilized. This expedites the build process by avoiding a potentially lengthy dependency restoration step, especially useful in CI/CD pipelines.
Explanation:
--no-restore
: This argument skips the automatic dependency restoration step usually conducted before a build. This is practical when you are sure that dependencies are up-to-date, saving time and computational resources.
Example Output:
Microsoft (R) Build Engine version 16.7.0+7fb82e5b2 for .NET
Copyright (C) Microsoft Corporation. All rights reserved.
MyApp -> C:\Projects\MyApp\bin\Debug\net5.0\MyApp.dll
Build succeeded.
0 Warning(s)
0 Error(s)
Time Elapsed 00:00:01.65
Use case 5: Compile with a specific verbosity level
Code:
dotnet build --verbosity minimal
Motivation:
Adjusting verbosity is essential for acquiring the right balance of information during the build. It allows developers to either minimize cluttered outputs or gain comprehensive diagnostic details, aligning with their current focus on debugging or monitoring.
Explanation:
--verbosity minimal
: Theverbosity
option modifies the amount of detail provided in the build output. ‘Minimal’ reduces output to just the essentials, but other levels (‘quiet’, ’normal’, ‘detailed’, ‘diagnostic’) are available to suit different needs, from basic success confirmation to in-depth analysis.
Example Output:
MyApp -> C:\Projects\MyApp\bin\Debug\net5.0\MyApp.dll
Use case 6: Compile for a specific runtime
Code:
dotnet build --runtime win-x64
Motivation:
In cross-platform development, you might need to build for different runtimes to ensure compatibility. This command allows you to specify the runtime environment for which your application should be compiled, thus catering to varied deployment targets.
Explanation:
--runtime win-x64
: This argument specifies the target runtime environment, such as ‘win-x64’ for 64-bit Windows. It designs the build to be runnable on specific systems, proving indispensable when creating platform-specific applications.
Example Output:
Microsoft (R) Build Engine version 16.7.0+7fb82e5b2 for .NET
Copyright (C) Microsoft Corporation. All rights reserved.
MyApp -> C:\Projects\MyApp\bin\Debug\net5.0\win-x64\MyApp.dll
Build succeeded.
0 Warning(s)
0 Error(s)
Time Elapsed 00:00:03.84
Use case 7: Specify the output directory
Code:
dotnet build --output path/to/directory
Motivation:
For organizational purposes and clarity in file management, designating a custom output directory for build artifacts can be invaluable. This option allows developers to direct outputs to specific locations, supporting cleaner project structures and easier artifact management.
Explanation:
--output path/to/directory
: Theoutput
argument lets you define the exact location for compiling output files, such as binaries or DLLs, facilitating integration with deployment scripts or easier access for testing.
Example Output:
Microsoft (R) Build Engine version 16.7.0+7fb82e5b2 for .NET
Copyright (C) Microsoft Corporation. All rights reserved.
MyApp -> C:\CustomOutput\MyApp.dll
Build succeeded.
0 Warning(s)
0 Error(s)
Time Elapsed 00:00:02.67
Conclusion:
The dotnet build
command proves to be an indispensable utility for .NET developers, supporting a broad range of build configurations and optimizations. Whether it’s for development, testing, or deploying applications across different platforms, understanding these use cases gives developers the flexibility to streamline and optimize their workflows proficiently.