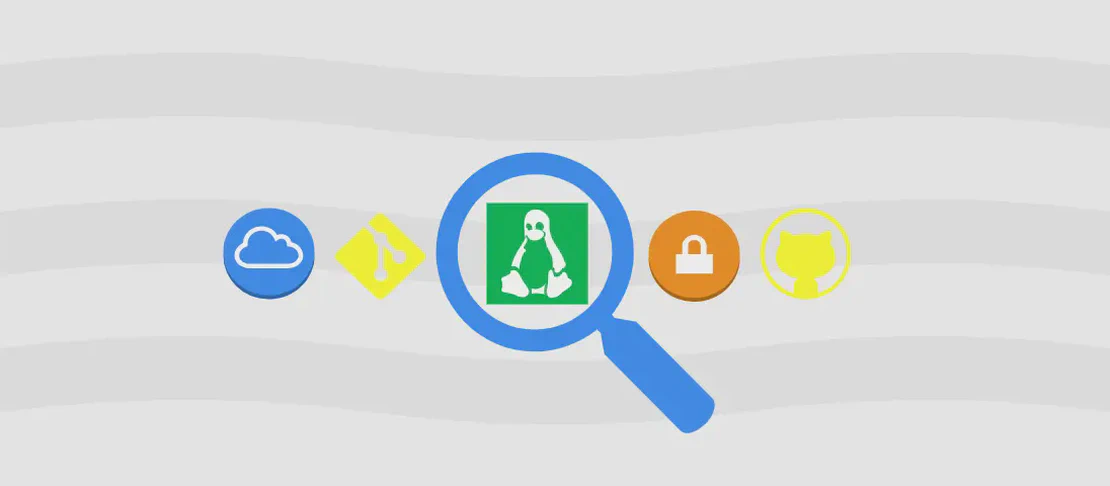
How to Use the Command 'dotnet' (with examples)
The dotnet
command is an essential tool for developers working with .NET Core, a cross-platform framework for building applications. It provides a command-line interface to manage .NET projects, execute code, and handle project dependencies, among other capabilities. This article explores various use cases of the dotnet
command with practical examples, helping developers understand its versatility and functionality in software development.
Use Case 1: Initialize a New .NET Project
Code:
dotnet new template_short_name
Motivation:
The initialization of a new .NET project is often the first step in application development. By using the dotnet new
command, developers can quickly set up the environment needed for their project. This command provides a way to bootstrap an application with a predefined structure, which includes essential configuration files and code templates. For instance, a developer who wants to start a web application can use a relevant template to speed up the development process, ensuring that all necessary components and settings are in place.
Explanation:
dotnet
: The command-line tool used to interact with .NET Core functionalities.new
: A subcommand that creates a new project or file from a template.template_short_name
: This represents the identifier of the template to be used for creating the project, such as ‘console’ for a console application or ‘mvc’ for a Model-View-Controller web application.
Example Output:
The template "Console Application" was created successfully.
This output indicates that the new project based on the selected template has been created, with all default files ready for development.
Use Case 2: Restore NuGet Packages
Code:
dotnet restore
Motivation:
Restoring NuGet packages is a crucial step during the build process of a .NET project. Packages are external dependencies that a project relies on, such as libraries that provide additional functionality. By running the dotnet restore
command, developers ensure that all necessary packages are downloaded and installed before building the project. This step is pivotal to avoid build failures caused by missing dependencies. Regular use of package restoration also keeps the project environment consistent across different development setups.
Explanation:
dotnet
: The main command-line interface for .NET Core operations.restore
: A subcommand that acquires and installs all the NuGet dependencies specified in the project’s configuration file.
Example Output:
Restoring packages for [path-to-project-file]...
Installed [package-name] [version]
Package restore completed successfully.
This output signifies that the NuGet packages have been successfully retrieved and installed, setting the stage for subsequent build operations.
Use Case 3: Build and Execute the .NET Project in the Current Directory
Code:
dotnet run
Motivation:
Running a .NET project involves building and executing the application’s code. The dotnet run
command is ideal for both these tasks in a streamlined manner. This is particularly beneficial during the development phase, allowing developers to test and debug their application frequently. Using this command boosts productivity by providing immediate feedback and enables quick identification and correction of potential issues in the code.
Explanation:
dotnet
: The versatile command-line tool for .NET Core projects.run
: A subcommand that builds the project in the current directory and then runs it. If the project is not built, it first compiles the code and resolves any dependencies before execution.
Example Output:
Building...
[Build output: Compilation success details]
Executing...
Hello, World!
This demonstrates the sequence of actions where the build process is completed successfully, and the application is executed to display its output.
Use Case 4: Run a Packaged Dotnet Application
Code:
dotnet path/to/application.dll
Motivation:
Running a packaged .NET application typically occurs in deployment or production environments. The dotnet
command allows the execution of a compiled application, provided the appropriate runtime is available. This use case is significant for situations where you have a pre-built application that should be run on a machine without needing the full development SDK. This ensures efficient resource use and minimizes system setup requirements.
Explanation:
dotnet
: The command-line interface to execute .NET applications.path/to/application.dll
: Specifies the path to the compiled application’s DLL file, which is the entry point for execution.
Example Output:
Executing packaged application...
Application started on http://localhost:5000
This output conveys that the packaged application has started successfully, running and serving requests at the specified address.
Conclusion:
The dotnet
command is an indispensable component of the .NET Core framework, providing comprehensive tools to create, manage, and execute .NET projects. By understanding and using various subcommands, developers can efficiently handle new project setups, manage dependencies, build and run applications, and execute packaged software. These capabilities streamline the development workflow, promoting productivity and aiding in the swift delivery of robust applications.