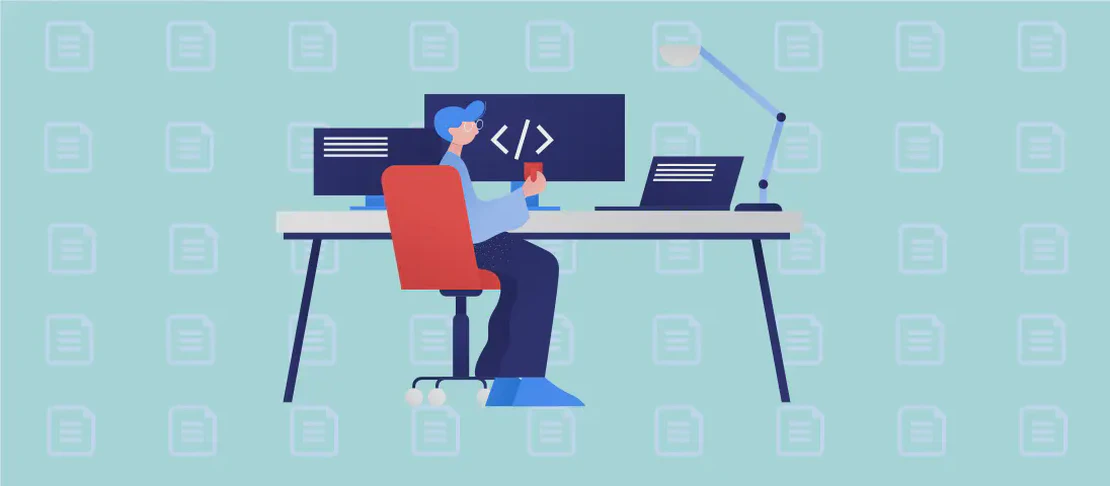
Exploring the 'dotnet ef' Command (with examples)
The dotnet ef
command is a powerful tool provided with the Entity Framework Core, allowing developers to perform design-time development tasks efficiently. This command is integral for managing database migrations, generating database-related code, and exploring different DbContext types available in your application. It streamlines the development process by providing a set of commands to handle tasks such as database updates, code generation for data contexts, and more, enabling a more agile and controlled database development experience.
Use case 1: Update the database to a specified migration
Code:
dotnet ef database update MigrationName
Motivation:
This use case is crucial during the process of continuous integration and deployment. When developers work in a team, they often introduce new migrations to adjust the database schema. Using this command ensures everyone’s local or development database schema is aligned with the latest changes dictated by a specific migration.
Explanation:
dotnet ef database update
: This part of the command is used to apply migrations to the database.MigrationName
: This is a placeholder for the specific name of the migration you wish to apply. By specifying the migration, the database schema is updated to reflect the exact state of the migration.
Example output:
Applying migration 'MigrationName'.
Done.
Use case 2: Drop the database
Code:
dotnet ef database drop
Motivation:
Dropping a database might be necessary during development when you need to reset the database to its initial state. This is especially useful if the migrations or the data in the database are incorrect or corrupted, providing a fresh environment to work with.
Explanation:
dotnet ef database drop
: The command is a straightforward way to remove the current database used by the application, which is defined in the DbContext.
Example output:
Are you sure you want to drop the database 'DatabaseName'? (Type 'y' to confirm) y
Dropping database 'DatabaseName'.
Database 'DatabaseName' dropped successfully.
Use case 3: List available DbContext
types
Code:
dotnet ef dbcontext list
Motivation:
Listing available DbContext types is handy when working on a project with multiple contexts or inheriting a project without clear documentation. It provides a quick overview of all DbContext classes within your project, facilitating easier navigation and understanding of the codebase.
Explanation:
dotnet ef dbcontext list
: This command lists all the DbContext types defined in the application, making it easy to view and manage them.
Example output:
Available DbContext types:
- ApplicationDbContext
- BloggingContext
Use case 4: Generate code for a DbContext
and entity types for a database
Code:
dotnet ef dbcontext scaffold "Server=myServerAddress;Database=myDataBase" Microsoft.EntityFrameworkCore.SqlServer
Motivation:
Generating a DbContext and entity classes from an existing database is useful when working with legacy systems or databases created outside of Entity Framework Core. It helps quickly integrate an existing database into a new or existing .NET project without manually creating models.
Explanation:
dotnet ef dbcontext scaffold
: This command starts the process of generating DbContext and entity classes."Server=myServerAddress;Database=myDataBase"
: This argument is the connection string for the database you want to reverse-engineer.Microsoft.EntityFrameworkCore.SqlServer
: This specifies the provider being used, essential for configuring Entity Framework Core to work with SQL Server.
Example output:
Scaffolded successfully.
To use the new DbContext, add it to the 'Startup.cs' file.
Use case 5: Add a new migration
Code:
dotnet ef migrations add InitialCreate
Motivation:
Adding a new migration is one of the first steps in creating a versioned set of database schema changes. This is important for tracking the evolution of the database schema and ensuring that database updates are applied consistently across different environments.
Explanation:
dotnet ef migrations add
: This part of the command begins the process of creating a new migration.InitialCreate
: This is the name of the migration being created, typically indicative of the changes introduced like ‘InitialCreate’.
Example output:
Migration 'InitialCreate' added.
Use case 6: Remove the last migration
Code:
dotnet ef migrations remove
Motivation:
Occasionally, a migration might need to be removed if changes are not finalized or incorrect. This command allows a developer to roll back the last added migration, removing it and affecting code changes, maintaining a clean project structure.
Explanation:
dotnet ef migrations remove
: This command targets the most recent migration added, removing its scripts and associated changes.
Example output:
Reverting migration 'MigrationName'.
Done.
Use case 7: List available migrations
Code:
dotnet ef migrations list
Motivation:
Listing available migrations offers a complete view of all migrations applied to the project. This is useful for understanding the sequence of changes that have been applied over time and is especially helpful in diagnosing issues related to missing or incomplete migrations.
Explanation:
dotnet ef migrations list
: This command outputs a list of all migrations present in the project, whether applied or pending.
Example output:
Available migrations:
- 20231001234321_InitialCreate
- 20231002094511_AddBlogTable
Use case 8: Generate an SQL script from migrations range
Code:
dotnet ef migrations script InitialCreate AddBlogTable
Motivation:
Generating an SQL script from a range of migrations is essential for situations where database changes need to be reviewed or applied externally. This is common in environments where direct code execution isn’t possible, or for integrations with other systems.
Explanation:
dotnet ef migrations script
: Starts the process to create an SQL script.InitialCreate
: This indicates the starting migration in the range.AddBlogTable
: Represents the ending migration in the range to be scripted.
Example output:
BEGIN TRANSACTION;
CREATE TABLE [dbo].[Blogs] (
[BlogId] int NOT NULL IDENTITY,
[Url] nvarchar(max) NOT NULL,
CONSTRAINT [PK_Blogs] PRIMARY KEY ([BlogId])
);
COMMIT;
Conclusion:
Exploring the various functionalities of the dotnet ef
command highlights its versatility and necessity in managing database tasks in a .NET application. From updating database schemas with specific migrations to generating entity classes from existing databases, this command simplifies many complex tasks, ensuring efficient and consistent database management throughout the application lifecycle. Whether you are tasked with maintaining data integrity, generating SQL scripts, or understanding the current DbContext architecture, dotnet ef
provides tools to streamline the process, enhance productivity, and maintain organizational alignment in the application development process.