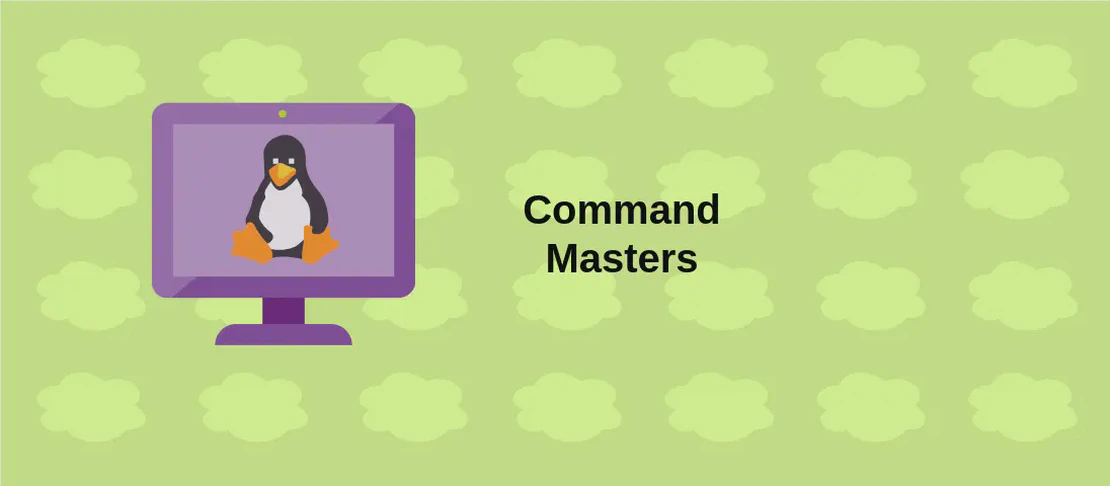
How to use the command 'dotnet publish' (with examples)
The dotnet publish
command is an essential tool in the .NET ecosystem, designed to prepare applications for deployment. This command packages a .NET application and its dependencies into a directory, making it ready to run on a target system, whether it’s a server, cloud provider, or any other hosting platform. By utilizing various options, developers have control over how the application is published, allowing for optimizations and specific configurations according to their deployment needs.
Compile a .NET project in release mode
Code:
dotnet publish --configuration Release path/to/project_file
Motivation:
Compiling a .NET project in release mode is crucial for optimizing the application’s performance and reducing its size. Release mode builds are fully optimized, which means they generally run faster and are more efficient compared to debug builds. This mode is typically used for production-ready applications, where efficiency and performance are paramount.
Explanation:
--configuration Release
: Specifies the build configuration. By default, this is set to Debug, but changing it to Release ensures that the build is optimized for performance and doesn’t include debugging symbols.path/to/project_file
: This parameter indicates the location of the .NET project file that you wish to publish.
Example Output:
Upon running the command, the output will typically indicate that the project is being compiled and published to a specified directory, often showing the path where the files are deployed. Any additional optimizations or warnings/errors will be displayed as well.
Publish the .NET Core runtime with your application for the specified runtime
Code:
dotnet publish --self-contained true --runtime runtime_identifier path/to/project_file
Motivation:
Publishing the .NET Core runtime with your application ensures that the application is self-sufficient and does not rely on a pre-installed version of .NET Core on the host system. This can be particularly useful in environments where you have no control over what runtimes are available or where installing a runtime is not feasible.
Explanation:
--self-contained true
: This option indicates that the application should be published with the .NET Core runtime included, creating a self-contained application.--runtime runtime_identifier
: Specifies the target runtime for the application. The runtime identifier (RID) determines the platform the application is intended to run on, e.g., win-x64, linux-x64.path/to/project_file
: Points to the location of the project file to be published.
Example Output:
The command will output a directory containing both the application and the necessary .NET Core runtime files, packaged together, and indicate the completion of the publishing process.
Package the application into a platform-specific single-file executable
Code:
dotnet publish --runtime runtime_identifier -p:PublishSingleFile=true path/to/project_file
Motivation:
Creating a single-file executable simplifies the deployment process as it consolidates all necessary files into one executable. This reduces the complexity associated with distributing multiple files and ensures that all required dependencies are packaged within the binary.
Explanation:
--runtime runtime_identifier
: Designates the platform the application will run on, such as win-x64 or linux-x64.-p:PublishSingleFile=true
: This property tells the publish process to bundle the application and its dependencies into a single executable file.path/to/project_file
: Refers to the location of the .NET project file.
Example Output:
The outcome of this command will be a single executable file located in the specified output directory. It simplifies the deployment by reducing it to one easily-distributable file.
Trim unused libraries to reduce the deployment size of an application
Code:
dotnet publish --self-contained true --runtime runtime_identifier -p:PublishTrimmed=true path/to/project_file
Motivation:
Trimming unused libraries is a valuable optimization technique that minimizes the size of the application release by removing unnecessary code. This is particularly useful for improving load times and reducing storage space, which can be critical in resource-sensitive environments like cloud deployments.
Explanation:
--self-contained true
: Indicates that the application is published with all necessary components, including the runtime.--runtime runtime_identifier
: Specifies the target platform.-p:PublishTrimmed=true
: Activates code trimming, which analyzes the application and excludes any unused library parts.path/to/project_file
: Identifies the path to the project being published.
Example Output:
The output will be a reduced-sized deployment package with unnecessary binaries and libraries removed. Information about the files and their sizes will be printed, indicating what was trimmed away.
Compile a .NET project without restoring dependencies
Code:
dotnet publish --no-restore path/to/project_file
Motivation:
Skipping dependency restoration is advantageous when you are confident that all dependencies are up to date. By bypassing this step, you can save time and resources during the build process, particularly in Continuous Integration/Continuous Deployment (CI/CD) environments where build time is essential.
Explanation:
--no-restore
: This flag skips the automatic restoration of dependencies before publishing. It’s useful when dependencies are pre-installed or restored in a previous step.path/to/project_file
: Indicates which project is to be published.
Example Output:
The command will proceed with publishing the project directly without any attempt to restore dependencies, displaying build progress and completion messages.
Specify the output directory
Code:
dotnet publish --output path/to/directory path/to/project_file
Motivation:
Specifying an output directory allows developers to control exactly where the published files are placed, providing clarity and organization during the deployment process. This is particularly useful in situations where a specific directory structure is required by deployment scripts or for organizational purposes.
Explanation:
--output path/to/directory
: Sets a custom directory where the published files will be placed. This directory can be anything specified by the developer.path/to/project_file
: The path to the project file that is being published.
Example Output:
The application files will be built and placed in the specified directory, ensuring they can be easily located and deployed as needed.
Conclusion:
The dotnet publish
command offers a flexible and efficient way to prepare .NET applications for deployment, covering a range of scenarios from performance optimization to platform-specific distributions. By understanding each option and use case, developers can streamline their deployment process, ensuring applications are ready for production with the right configuration.