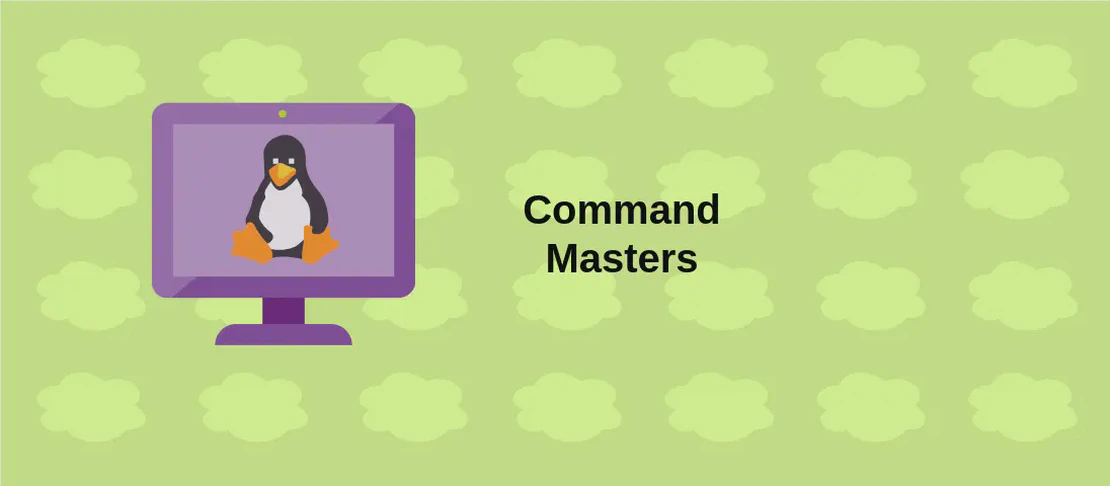
How to Restore Dependencies with 'dotnet restore' (with examples)
The dotnet restore
command is an essential tool in the .NET ecosystem, responsible for restoring the dependencies and tools required by a .NET project. Whenever a new project is created, or source control is pulled into a fresh environment, restoring dependencies is vital to ensure that you have all the required packages to build and run your project successfully. This command interacts with NuGet, the package manager for .NET, to download all necessary dependencies as listed in the project file.
Use case 1: Restore Dependencies for a .NET Project or Solution in the Current Directory
Code:
dotnet restore
Motivation:
When working within a .NET development environment, a typical scenario involves updating or pulling a project from a version control repository such as Git. The source code you pull may not come with the required binary dependencies included. Therefore, a straightforward dotnet restore
is essential to ensure that your development environment possesses all necessary dependencies before you attempt to build and run the project.
Explanation:
dotnet restore
: This command, when issued without any additional arguments, will locate the.csproj
or.sln
file in the current directory and proceed to restore all necessary dependencies and tools specified in those files.
Example Output:
Restoring packages for C:\MyProject\MyProject.csproj...
Generating MSBuild file C:\MyProject\obj\MyProject.csproj.nuget.g.props.
Generating MSBuild file C:\MyProject\obj\MyProject.csproj.nuget.g.targets.
Restore completed in 2.14 sec for C:\MyProject\MyProject.csproj.
Use case 2: Restore Dependencies for a .NET Project or Solution in a Specific Location
Code:
dotnet restore path/to/project_or_solution
Motivation:
When multiple projects reside within a single repository, each with distinct sets of dependencies, it may be necessary to specify which project or solution you intend to restore. This is particularly useful in build pipelines or scripts where operations must be performed on specific projects.
Explanation:
dotnet restore path/to/project_or_solution
: This restores dependencies for a project or solution file located at a particular path rather than the current directory. Providing the path allows for targeted operation on the specified project.
Example Output:
Restoring packages for D:\Projects\AnotherApp\AnotherApp.csproj...
Generating MSBuild file D:\Projects\AnotherApp\obj\AnotherApp.csproj.nuget.g.props.
Generating MSBuild file D:\Projects\AnotherApp\obj\AnotherApp.csproj.nuget.g.targets.
Restore completed in 1.82 sec for D:\Projects\AnotherApp\AnotherApp.csproj.
Use case 3: Restore Dependencies Without Caching the HTTP Requests
Code:
dotnet restore --no-cache
Motivation:
There are situations where you might need to ensure a fresh copy of the package is downloaded, ignoring any cached versions. Perhaps packages have been updated, or there are discrepancies between what’s in your cache versus the latest available packages. This is why running restore operations without cached HTTP requests can be a vital troubleshooting tool.
Explanation:
--no-cache
: This argument ensures that the restore operation bypasses the cached HTTP requests. By doing this, all the packages are downloaded afresh from their respective sources.
Example Output:
Restoring packages for C:\NoCacheProject\NoCacheProject.csproj...
Restored package (NoCachePackage) from https://api.nuget.org/v3/index.json at 10:45 AM
Restore completed in 3.44 sec for C:\NoCacheProject\NoCacheProject.csproj.
Use case 4: Force All Dependencies to Be Resolved Even If the Last Restore Was Successful
Code:
dotnet restore --force
Motivation:
When you suspect that the state of the system and the dependencies might be out-of-sync due to manual changes or an inconsistent package state, forcing a resolution of all dependencies can help reconcile these discrepancies. This command can be especially helpful in continuous integration (CI) environments where state consistency is critical.
Explanation:
--force
: Forces the restoration process to re-evaluate and resolve all dependencies, regardless of whether the previous restore was deemed successful.
Example Output:
Forcing restore of packages for C:\ForceRestoreProject\ForceRestoreProject.csproj...
Resolve: PackageNameA successfully restored.
Resolve: PackageNameB successfully restored.
Restore completed in 4.65 sec for C:\ForceRestoreProject\ForceRestoreProject.csproj.
Use case 5: Restore Dependencies Using Package Source Failures as Warnings
Code:
dotnet restore --ignore-failed-sources
Motivation:
In some scenarios, not all package sources might be reliable, or might be intermittently unavailable. Ignoring failed package sources can be practical when the necessary packages are available across multiple sources and flexibility exists regarding from where the packages are fetched. This ensures that the restoration process continues as long as existing packages can be resolved, potentially falling back to alternative sources.
Explanation:
--ignore-failed-sources
: Instructs the restore process to continue and treat package source retrieval issues as warnings instead of errors.
Example Output:
Warning: Some packages could not be retrieved from https://nonresponsive.nuget.org, continuing from other sources.
Restore completed in 2.95 sec for C:\IgnoreFailedSourcesProject\IgnoreFailedSourcesProject.csproj.
Use case 6: Restore Dependencies With a Specific Verbosity Level
Code:
dotnet restore --verbosity quiet|minimal|normal|detailed|diagnostic
Motivation:
Depending on the context or the user, different levels of verbosity in command output are useful. For debugging purposes, a more verbose output might be necessary to identify where a problem lies. Conversely, in automated scripts or CI pipelines, reduced verbosity can declutter logs and make them easier to review.
Explanation:
--verbosity [level]
: Adjusts the verbosity of the output from the restore process to match the need, ranging fromquiet
(least amount of information) todiagnostic
(most detailed information).
Example Output for normal
verbosity:
Restoring packages for C:\VerboseProject\VerboseProject.csproj...
Restore operation started
Package PackageNameA restored
Package PackageNameB restored
Restore completed in 2.58 sec for C:\VerboseProject\VerboseProject.csproj.
Conclusion:
The dotnet restore
command is indispensable for managing project dependencies effectively in the .NET environment. This article illustrates various use cases where specific arguments and options can be utilized to tailor the restoration process according to the project’s needs, aligning it with different development and operational scenarios.