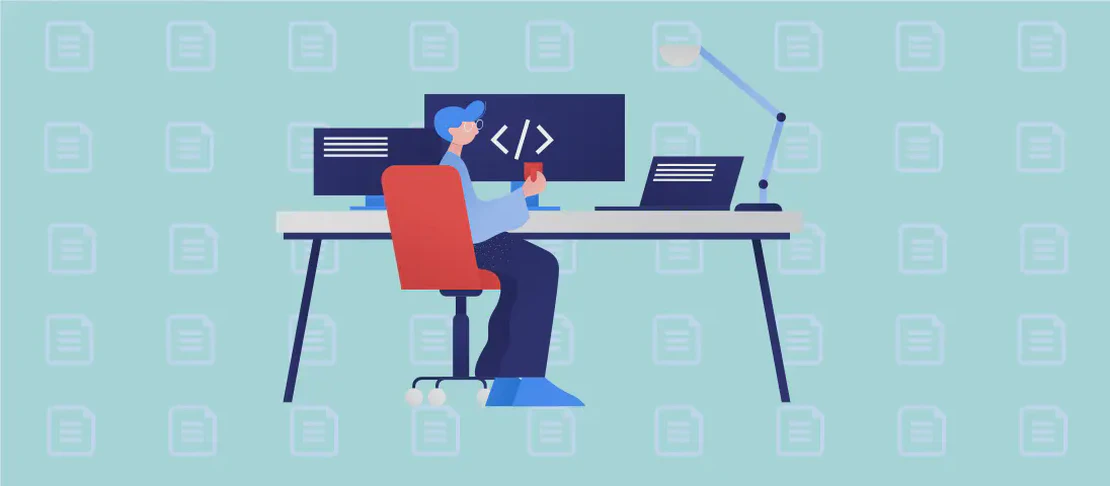
How to use the command 'dotnet run' (with examples)
The dotnet run
command is an essential utility in the .NET ecosystem that enables developers to execute .NET applications directly from the source without needing to perform the compile and launch steps separately. It simplifies the development workflow by automating these processes, thus providing a streamlined experience. This command is versatile enough to support a variety of scenarios such as running applications directly from source code, specifying target frameworks, and even selecting specific architectures and operating systems.
Use case 1: Run the project in the current directory
Code:
dotnet run
Motivation: When building a .NET application, there are scenarios where you might want to quickly test or run the current project without navigating through multiple directories or managing complex build scripts. This use case is particularly useful during ongoing development when continuous integration or manual testing is required.
Explanation: The dotnet run
command executed without any additional arguments assumes that you want to run the .NET project located in the current working directory. It automatically builds the project and launches it.
Example Output: On executing this command, it compiles your .NET application and runs it, displaying output similar to what you’d expect by running the compiled binaries separately:
Hello, World!
Press any key to exit...
The above output reflects a minimal console application that prints “Hello, World!” and waits for user input before exiting.
Use case 2: Run a specific project
Code:
dotnet run --project path/to/file.csproj
Motivation: In larger solution environments, where multiple projects exist under a common directory or solution file, there might be a specific project you need to execute. This command allows you to specify exactly which project you want to run, thus providing precision and flexibility during development and testing.
Explanation: Here, --project
is an argument that indicates you are specifying a path to a particular .csproj
file. By giving the path path/to/file.csproj
, you’re telling the dotnet run
command to execute this specific project.
Example Output: Depending on the project, the output may be:
Running 'SampleProject'...
Welcome to SampleProject!
Press any key to exit...
This output suggests the sample project executed successfully, providing a custom message defined within the project’s code.
Use case 3: Run the project with specific arguments
Code:
dotnet run -- arg1=foo arg2=bar
Motivation: During development, it is often necessary to run an application with various input parameters to test different configurations or functionalities. This is especially useful in command-line applications where changing input arguments modifies the application’s behavior.
Explanation: The --
argument is used to signify the end of dotnet options and the beginning of application arguments. arg1=foo
and arg2=bar
are typical example arguments that your application could be designed to accept and process, affecting its functionality or output.
Example Output: If the application’s code is designed to process and display these arguments, the output might look like:
Processing arguments...
arg1: foo
arg2: bar
Done!
This indicates the application received and handled the input arguments as intended.
Use case 4: Run the project using a target framework moniker
Code:
dotnet run --framework net7.0
Motivation: In modern software development, applications can target multiple versions of the .NET framework. This use case is critical when you need to verify the compatibility or behavior of your project under different framework versions.
Explanation: The --framework net7.0
argument specifies which version of the .NET framework should be used to run the project. In this example, net7.0
indicates the application should run using the .NET 7.0 framework.
Example Output: Executing this command would typically yield output such as:
Running on .NET 7.0
Hello from .NET 7.0
Press any key to exit...
The output shows a successful execution under the specified framework, implying the application is working as expected with .NET 7.0.
Use case 5: Specify architecture and OS
Code:
dotnet run --arch x64 --os linux
Motivation: With the rise of cross-platform development, ensuring that an application behaves correctly across various systems is crucial. This feature allows developers to test their .NET applications on different architectures and operating systems without changing the development environment.
Explanation: The --arch x64
and --os linux
arguments instruct the dotnet run
command to execute the application as if it’s running on a 64-bit Linux environment. These options have been made available since .NET 6, enhancing the capability to simulate diverse deployment scenarios.
Example Output: The successful execution might produce output akin to:
Simulating Linux x64 environment...
Application running on Linux architecture: x64
Operation completed successfully.
Such responses confirm that your application can handle conditions expected on a Linux x64 platform, prompting potential adjustments or affirmations for cross-platform compatibility.
Conclusion:
The dotnet run
command is a versatile and powerful tool within the .NET Core suite, fundamentally aiding developers in minimizing repetitive tasks associated with building and running applications. Its ability to handle various scenarios from running projects in specific directories to testing on different frameworks and architectures makes it indispensable for .NET developers aiming for efficient and effective software development workflows.