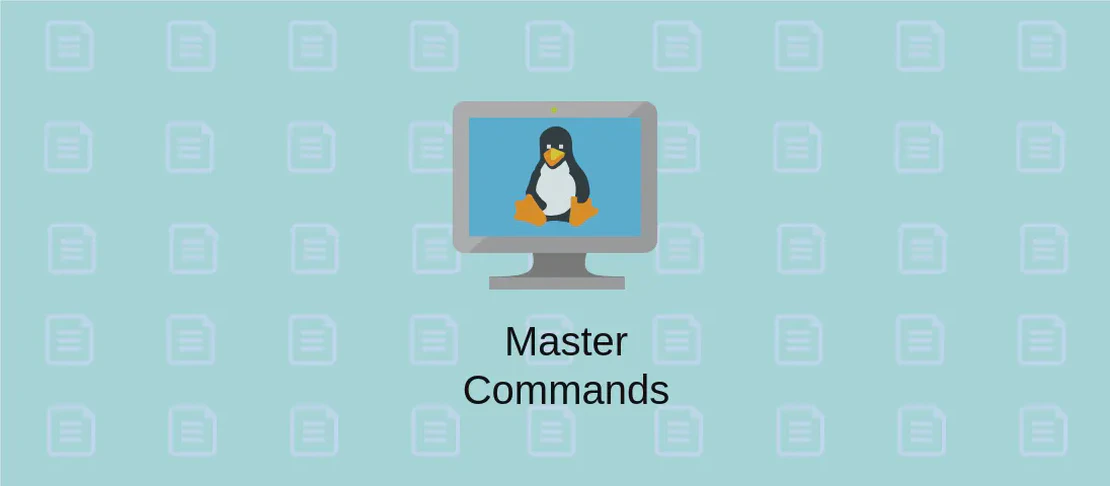
Managing .NET Tools with 'dotnet tool' (with examples)
The ‘dotnet tool’ command is a multifaceted command within the .NET ecosystem that provides developers with capabilities to manage .NET CLI tools with ease. These tools, available on NuGet, are commonly utilized to extend the functionality of .NET development environments globally or locally. The command enables users to install, update, uninstall, list, and search for CLI tools, enhancing productivity by facilitating a seamless integration and management of tools in development workflows.
Install a Global Tool
Code:
dotnet tool install --global dotnetsay
Motivation: Installing a global tool ensures that it is available across all .NET projects on your system, making it incredibly convenient for frequently used tools like ‘dotnetsay’, a playful tool that outputs messages, which can be beneficial for testing scripting capabilities or displaying notifications.
Explanation: The command dotnet tool install
signals the initiation of a tool installation. The --global
argument specifies that the tool should be installed for the entire system, rather than being scoped to a specific project. Finally, dotnetsay
is the name of the tool to install.
Example Output:
You can invoke the tool using the following command: dotnetsay
Tool 'dotnetsay' (version '3.0.0') was successfully installed.
Install Tools Defined in the Local Tool Manifest
Code:
dotnet tool restore
Motivation: This command is essential when working with a project that has predefined tools listed in a local tool manifest file. It ensures that all necessary tools are installed, enabling smooth project setup and execution without manual intervention.
Explanation: The command dotnet tool restore
reads the tool manifest file located within the project directory and installs each tool listed therein. This process replicates the environment the project creator intended for all collaborators.
Example Output:
Tool 'exampletool' (version '1.0.0') was restored.
Tool 'anothertool' (version '2.1.5') was restored.
Update a Specific Global Tool
Code:
dotnet tool update --global tool_name
Motivation: Regularly updating tools can introduce new features and fixes. Updating a global tool ensures that you have the latest improvements and security patches. For example, if ’tool_name’ is a tool you frequently rely on, it’s wise to keep it up-to-date.
Explanation: The dotnet tool update
command initiates the update process for a specified tool. The --global
flag indicates that the operation is on a system-wide tool. tool_name
should be replaced with the actual name of the tool you wish to update.
Example Output:
Tool 'tool_name' was successfully updated from version '1.0.0' to version '1.2.0'.
Uninstall a Global Tool
Code:
dotnet tool uninstall --global tool_name
Motivation: Uninstalling unused or outdated tools helps maintain a clean working environment, reducing clutter and potential version conflicts. If a tool like ’tool_name’ is no longer needed, removing it ensures efficiency.
Explanation: The dotnet tool uninstall
command begins the removal process for a specified tool. The --global
flag specifies that the tool to be removed is installed system-wide. tool_name
represents the name of the tool to uninstall.
Example Output:
Tool 'tool_name' (version '1.2.0') was successfully uninstalled.
List Installed Global Tools
Code:
dotnet tool list --global
Motivation: Regularly reviewing installed tools can help in managing and auditing tool usage. Listing tools gives a clear overview of all globally installed tools, helping in managing versions and identifying outdated or unused tools.
Explanation: The dotnet tool list
command is used to fetch a list of all installed tools. The --global
argument restricts the listing to tools that are installed across the entire system.
Example Output:
Package Id Version Commands
-------------------------------------------------------
dotnetsay 3.0.0 dotnetsay
exampletool 1.5.7 example
Search Tools in NuGet
Code:
dotnet tool search search_term
Motivation: When looking for new tools to enhance development capabilities, searching the NuGet repository via the CLI can quickly yield potential candidates. For example, using ‘search_term’ can help pinpoint tools that match a specific functionality or need.
Explanation: The dotnet tool search
command interacts with NuGet to search for tools. search_term
is the keyword or phrase that the search is based on, potentially representing a function, framework, or tool name.
Example Output:
NuGet package source 'https://api.nuget.org/v3/index.json'
ID Version Description
---------------------------------------------------------------
exampletool 1.0.0 An example tool for demonstration.
utilitytool 2.1.3 A utility tool to ease developer tasks.
Display Help
Code:
dotnet tool --help
Motivation: Understanding the available options and syntax for commands can enhance usage efficiency. Using the help option provides detailed guidance on command usage, benefiting both newcomers and experienced users trying to recall more obscure options.
Explanation: The dotnet tool --help
command outputs detailed information about the ‘dotnet tool’ usage, including subcommands, arguments, and examples, acting as a reference guide.
Example Output:
Usage: dotnet tool [options] [command]
Commands:
install Install a tool
uninstall Uninstall a tool
update Update a tool
Use "dotnet tool [command] --help" for more information about a command.
Conclusion:
Mastering the ‘dotnet tool’ command can significantly enhance a developer’s ability to manage .NET CLI tools efficiently. By understanding and utilizing these use cases, developers can maintain an optimal set of tools tailored to their specific needs, ensuring a robust and productive development environment. Whether it’s installing necessary global tools, managing updates, or searching for new utilities, ‘dotnet tool’ offers a comprehensive set of commands to empower the .NET development process.