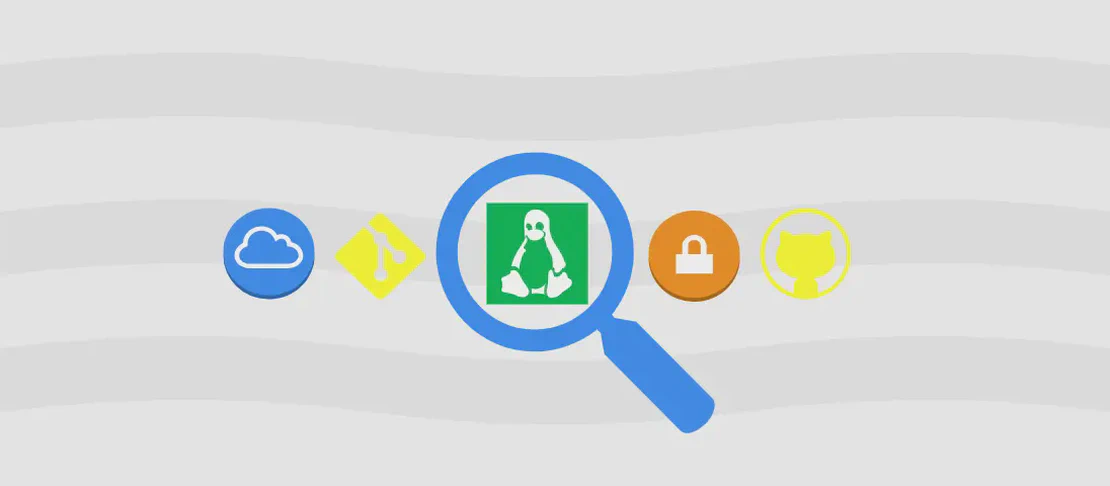
How to use the command 'echo' (with examples)
The echo
command is a built-in command in most operating systems that allows you to print text to the console. It is commonly used in shell scripts and command-line interfaces.
Use case 1: Print a text message
Code:
echo "Hello World"
Motivation: This use case is useful when you want to display a simple text message on the console. It can be used for informational purposes or to provide feedback to the user.
Explanation:
The echo
command is followed by the text message you want to print. In this case, the text message is “Hello World”. Quotes are optional for simple text messages, but they are necessary if the text contains spaces or special characters.
Example Output:
Hello World
Use case 2: Print a message with environment variables
Code:
echo "My path is $PATH"
Motivation: This use case can be helpful when you need to display the value of an environment variable. It allows you to include the value of the environment variable in the output message.
Explanation:
The echo
command is used to print the text message “My path is " followed by the value of the $PATH
environment variable. The $PATH
is a special variable that stores a list of directories where executable files are located.
Example Output:
My path is /usr/local/bin:/usr/bin:/bin
Use case 3: Print a message without the trailing newline
Code:
echo -n "Hello World"
Motivation: This use case can be useful when you want to print text without a trailing newline character. It can be used to concatenate multiple lines of output into a single line.
Explanation:
The -n
option is used with the echo
command to suppress the trailing newline character. When this option is used, the text message is printed without moving to the next line.
Example Output:
Hello World%
(Note: The %
represents the terminal prompt)
Use case 4: Append a message to the file
Code:
echo "Hello World" >> file.txt
Motivation: This use case is helpful when you want to append a text message to a file without overwriting its content. It allows you to continuously add new content to an existing file.
Explanation:
The >>
symbol is used to redirect the output of the echo
command to a file. In this case, the message “Hello World” is appended to the file.txt
file.
Example Output:
(file.txt)
Line 1: ...
Line 2: ...
Line 3: Hello World
Use case 5: Enable interpretation of backslash escapes
Code:
echo -e "Column 1\tColumn 2"
Motivation: This use case is useful when you want to print special characters, such as tab or newline, using backslash escapes. It allows you to control the formatting of the output message.
Explanation:
The -e
option is used with the echo
command to enable the interpretation of backslash escapes. In this case, the backslash escape \t
is used to represent a tab character in the output.
Example Output:
Column 1 Column 2
Use case 6: Print the exit status of the last executed command
Code:
echo $?
Motivation: This use case can be helpful when you want to check the exit status of the last executed command. It allows you to determine if the command was successful or if an error occurred.
Explanation:
The $?
is a special variable that stores the exit status of the last executed command. By using echo $?
, you can print the exit status to the console.
Example Output:
0
(Note: The output might be different depending on the previous command executed)
Conclusion
The echo
command is a versatile command for printing text to the console. It can be used in various scenarios, such as displaying messages, working with environment variables, redirecting output to files, and controlling the formatting of the output. Understanding and utilizing the different use cases of the echo
command can greatly enhance your command-line experience.