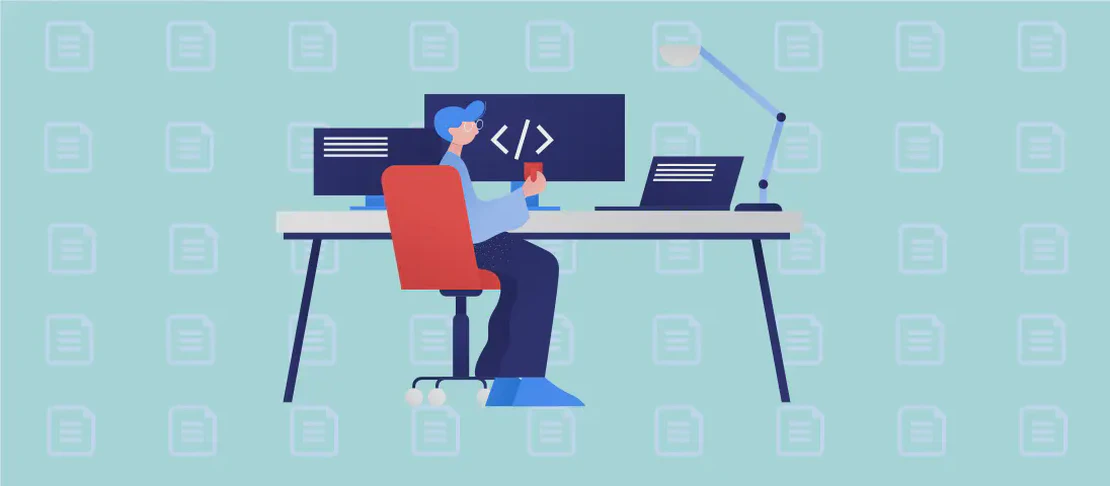
How to use the command `egrep` (with examples)
The egrep
command is a powerful tool used in Unix-based systems for text processing. It is designed to search for patterns within files using extended regular expressions, which allow for more complex pattern matching. Unlike simpler search commands, egrep
supports a wider range of metacharacters such as ?
, +
, {}
, ()
, and |
, making it highly versatile for various searching tasks in both single and multi-file contexts.
Use case 1: Search for a pattern within a file
Code:
egrep "search_pattern" path/to/file
Motivation:
When working with large text files, you might want to quickly find specific text patterns without opening the file in an editor. For example, if you’re looking for occurrences of an error message within a log file, egrep
can help locate these instances efficiently.
Explanation:
egrep
: Invokes theegrep
command."search_pattern"
: This is the extended regular expression you are searching for within the file. By using patterns, you can specify exact text to match.path/to/file
: Indicates the file path whereegrep
should search for the pattern.
Example output:
Match 1: This is the line containing search_pattern.
Match 2: Another line that has search_pattern.
Use case 2: Search for a pattern within multiple files
Code:
egrep "search_pattern" path/to/file1 path/to/file2
Motivation:
Sometimes the data you need to search is spread across multiple files. For instance, if you are searching for a configuration setting that may be defined in several configuration files, being able to search through multiple files with a single command is very efficient.
Explanation:
egrep
: Initiates the search function."search_pattern"
: The pattern you’re looking for, supporting extended regular expressions.path/to/file1 path/to/file2
: Paths to multiple files;egrep
searches each file in the order they are listed.
Example output:
file1: Line 3: Example line with search_pattern.
file2: Line 10: search_pattern found here too.
Use case 3: Search stdin
for a pattern
Code:
cat path/to/file | egrep "search_pattern"
Motivation:
When a file or a stream of data is piped through another command, searching for patterns directly in the data’s stream becomes necessary. This method is particularly useful in scripts where data transformations are applied first, and then results are piped to egrep
.
Explanation:
cat path/to/file
: Outputs the contents of the file tostdout
.|
: Pipes the output of thecat
command toegrep
, allowingegrep
to process it.egrep "search_pattern"
: Searches for the specified pattern in the piped data.
Example output:
Stream line with search_pattern.
Use case 4: Print file name and line number for each match
Code:
egrep --with-filename --line-number "search_pattern" path/to/file
Motivation:
In debugging or log analysis, knowing exactly where in the file a match occurs is crucial. This use case helps in pinpointing the exact location of a pattern match by providing both the filename and the line number.
Explanation:
egrep
: Utilizes the extended search capabilities ofegrep
.--with-filename
: Ensures that the output includes the name of each file where the pattern is found.--line-number
: Adds the exact line number within the file, aiding in navigation and editing."search_pattern"
: The search target using regular expressions.path/to/file
: Specifies the file to be searched.
Example output:
path/to/file:5: Here is a line with the search_pattern.
Use case 5: Search for a pattern in all files recursively in a directory, ignoring binary files
Code:
egrep --recursive --binary-files=without-match "search_pattern" path/to/directory
Motivation:
When managing large codebases or datasets, it is often necessary to perform a comprehensive search across all files in a directory tree. By ignoring binary files, you focus the search on text files where the pattern is actually relevant or existent.
Explanation:
egrep
: Executes the search.--recursive
: Recursively searches through directories and their subdirectories.--binary-files=without-match
: Excludes binary files from the search to prevent irrelevant binary data from cluttering the output."search_pattern"
: The expression you are trying to find.path/to/directory
: The root directory where the recursive search should commence.
Example output:
directory/file1.txt:20: Line containing search_pattern in file1.
directory/subdir/file2.txt:13: Another search_pattern match.
Use case 6: Search for lines that do not match a pattern
Code:
egrep --invert-match "search_pattern" path/to/file
Motivation:
There are situations where you need to filter out lines that contain undesirable patterns, such as comments in a configuration file or certain log messages in a log file. egrep
can exclude these and provide a cleaner set of results.
Explanation:
egrep
: Initiates the pattern search.--invert-match
: Instructsegrep
to output lines that do not match the specified pattern."search_pattern"
: The target pattern to exclude from the results.path/to/file
: The file source for the search.
Example output:
Non-matching line 1.
Relevant line without the pattern.
Conclusion:
The egrep
command is an indispensable tool for text processing on Unix-based systems. Its ability to search using extended regular expressions makes it flexible and powerful for various use cases, from simple file searches to complex multi-file operations. By leveraging the different options and switches, such as --with-filename
, --line-number
, --recursive
, and --invert-match
, users can tailor their searches to efficiently extract and manipulate textual data.