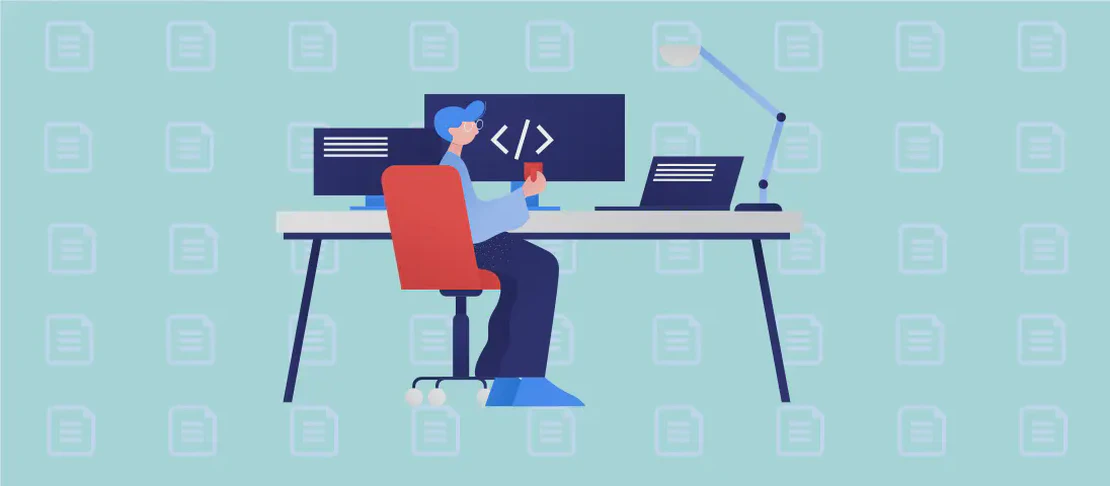
How to use the command 'electron-packager' (with examples)
The ’electron-packager’ command is a tool used to build Electron app executables for Windows, Linux, and macOS. It requires a valid package.json in the application directory. The command provides various options to package the application for different architectures and platforms.
Use case 1: Package an application for the current architecture and platform:
Code:
electron-packager "path/to/app" "app_name"
Motivation: This use case allows you to package the Electron application for the current architecture and platform without specifying any additional options. It is useful when you want to quickly generate an executable for your application without worrying about the target platform.
Explanation:
- “path/to/app”: This argument specifies the path to the Electron application directory that contains the package.json file.
- “app_name”: This argument sets the name of the packaged application.
Example output:
Electron Packager: Creating App Image...
Electron Packager: Application packaged successfully!
Use case 2: Package an application for all architectures and platforms:
Code:
electron-packager "path/to/app" "app_name" --all
Motivation: This use case allows you to package the Electron application for all supported architectures and platforms. It is useful when you want to generate executables for multiple target environments and ensure compatibility.
Explanation:
- “–all”: This option specifies that the application should be packaged for all architectures and platforms, including Windows, Linux, and macOS.
Example output:
Electron Packager: Packaging for Windows x64...
Electron Packager: Packaging for Linux x64...
Electron Packager: Packaging for macOS x64...
Electron Packager: Application packaged successfully for all platforms!
Use case 3: Package an application for 64-bit Linux:
Code:
electron-packager "path/to/app" "app_name" --platform="linux" --arch="x64"
Motivation: This use case allows you to package the Electron application specifically for the 64-bit Linux platform. It is useful when you want to distribute your application as a Linux executable targeting a specific architecture.
Explanation:
- “–platform”: This option specifies the target platform. In this case, it is set to “linux” to package the application for Linux.
- “–arch”: This option specifies the target architecture. In this case, it is set to “x64” to package the application specifically for 64-bit.
Example output:
Electron Packager: Packaging for Linux x64...
Electron Packager: Application packaged successfully for 64-bit Linux!
Use case 4: Package an application for ARM macOS:
Code:
electron-packager "path/to/app" "app_name" --platform="darwin" --arch="arm64"
Motivation: This use case allows you to package the Electron application specifically for the ARM macOS platform. It is useful when you want to distribute your application as a macOS executable targeting the ARM architecture.
Explanation:
- “–platform”: This option specifies the target platform. In this case, it is set to “darwin” to package the application for macOS.
- “–arch”: This option specifies the target architecture. In this case, it is set to “arm64” to package the application specifically for ARM.
Example output:
Electron Packager: Packaging for macOS arm64...
Electron Packager: Application packaged successfully for ARM macOS!
Conclusion:
The ’electron-packager’ command is a versatile tool for packaging Electron applications as executables for different architectures and platforms. It provides options to package for the current architecture and platform, all architectures and platforms, specific Linux architectures, and specific macOS architectures. With its simplicity and flexibility, ’electron-packager’ streamlines the process of distributing your Electron apps to a wide range of users.