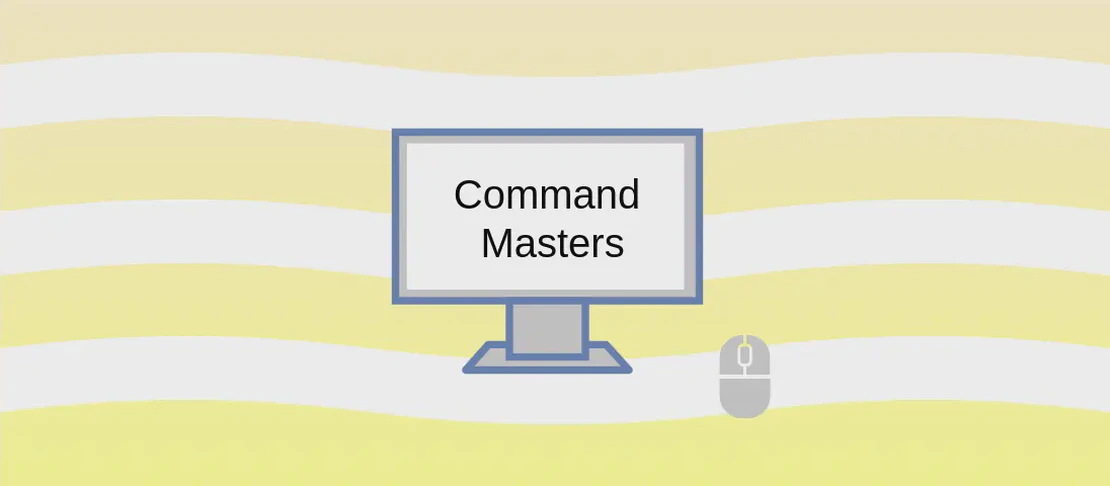
How to Use the Command 'elm' (with examples)
Elm is a functional programming language that compiles to JavaScript, designed for building web applications with a strong emphasis on simplicity and quality tooling. The ’elm’ command facilitates the management and interaction with Elm projects, providing tools for compiling Elm source files, starting an interactive shell, setting up projects, and more. Below are several use cases demonstrating how to effectively use the ’elm’ command.
Use case 1: Initialize an Elm project
Code:
elm init
Motivation:
Initializing an Elm project is often the first step when starting a new Elm application. It sets up the necessary project structure and creates a foundational setup that can be further developed. The elm init
command simplifies this process by automatically generating an elm.json file, which contains all the project settings and dependency declarations.
Explanation:
elm
: Invokes the Elm command-line tool.init
: Directs the tool to initialize a new Elm project, setting up the basic requirements and configurations.
Example Output:
Upon executing elm init
, the following output is typically produced:
Hello! This command will create an elm.json file.
The command will ask if you want to proceed, and upon confirmation, an elm.json
file will be created in the current directory.
Use case 2: Start interactive Elm shell
Code:
elm repl
Motivation: For exploring Elm expressions and testing snippets of Elm code in real-time, the Elm REPL (Read-Eval-Print Loop) is invaluable. It provides an interactive shell where developers can quickly evaluate Elm expressions and ascertain their results, which is particularly useful for learning, debugging, and experimenting with Elm syntax and functions.
Explanation:
elm
: Calls the Elm command-line interface.repl
: Launches the interactive shell where Elm statements can be executed on-the-fly.
Example Output:
Upon entering the command elm repl
in the terminal, you will see:
Elm REPL 0.19.1
Type :help for more information, :exit to exit
>
You can now start typing Elm expressions to immediately see their results.
Use case 3: Compile an Elm file, output to an index.html file
Code:
elm make source
Motivation: Compiling an Elm source file into HTML is a common step in the Elm development workflow, allowing developers to generate a standalone webpage from their Elm code. This is particularly useful for quick tests, demos, or deploying small applications.
Explanation:
elm
: Invokes the Elm command-line utility.make
: Compiles Elm source files.source
: Specifies the input file containing the Elm source code you want to compile.
Example Output:
Running elm make source
outputs:
Success! Compiled 1 module.
An index.html file will be generated containing your compiled Elm application.
Use case 4: Compile an Elm file, output to a JavaScript file
Code:
elm make source --output=destination.js
Motivation: Often in modern web development, integrating Elm into larger JavaScript applications is a desired capability. Compiling Elm code to JavaScript provides a seamless and efficient way to integrate Elm with existing JavaScript tooling and applications, or to use with JavaScript package managers like npm.
Explanation:
elm
: Launches the Elm command-line tool.make
: Instructs Elm to compile the specified source file.source
: Identifies the source Elm file to compile.--output=destination.js
: Defines the output destination and filename for the compiled JavaScript, wheredestination.js
is the name of the target file.
Example Output: Executing the command results in:
Success! Compiled 1 module.
A file named destination.js
is created in the working directory with the compiled JavaScript code.
Use case 5: Start a local web server that compiles Elm files on page load
Code:
elm reactor
Motivation: Elm Reactor is highly useful in the development workflow as it facilitates rapid application prototyping. By starting a local web server that automatically compiles Elm files upon page load, it allows developers to see changes directly in the browser, improving development speed and user feedback loops.
Explanation:
elm
: Invokes the Elm command-line tool.reactor
: Starts a development server which serves Elm code and compiles it in response to web page requests.
Example Output:
Running elm reactor
will show:
Go to <http://localhost:8000> to see your project dashboard.
Visiting the link in a web browser provides an interface for navigating and running Elm files in the project.
Use case 6: Install Elm package from the package repository
Code:
elm install author/package
Motivation: Elm’s package system is an integral part of working with Elm ecosystems. Installing packages allows developers to leverage existing solutions and libraries, accelerating development and encouraging code reuse and sharing.
Explanation:
elm
: Executes the Elm toolchain.install
: Specifies that you wish to add a package to your Elm project.author/package
: Identifies the particular package to install, formatted asauthor/name
.
Example Output:
When you install a package using elm install
, you may see:
Here is my plan:
- Add the author/package to your project's dependencies.
Do you approve? [Y/n]:
After approval, the package gets added to your elm.json dependencies.
Conclusion
The Elm command-line tools provide an essential interface for working with Elm projects, simplifying the workflow for initializing projects, testing code snippets, compiling code to different formats, running a local server, and managing dependencies. In each use case, understanding the commands and their components enhances productivity and facilitates the seamless development of robust web applications in Elm.