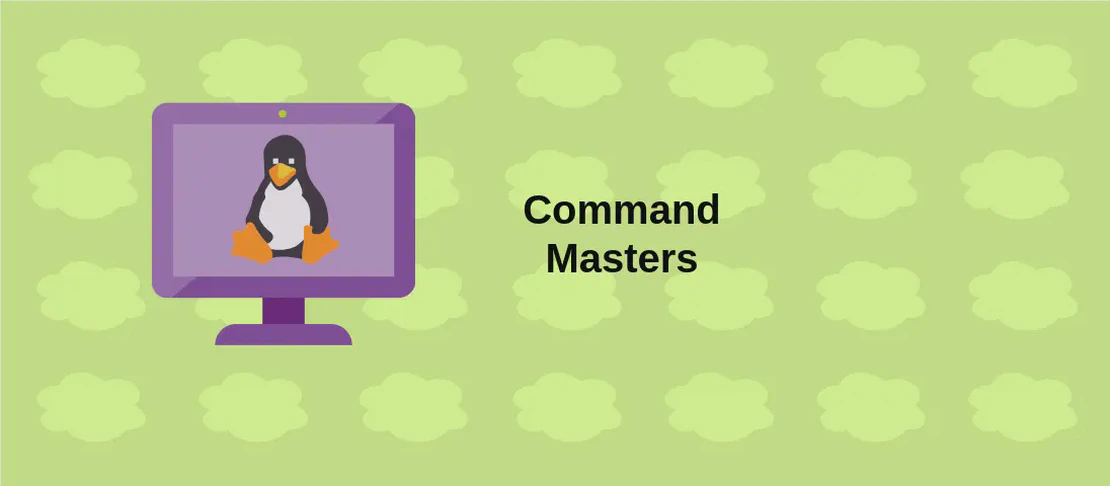
How to Use the Command 'ember' (with Examples)
Ember CLI is a user-friendly command-line interface for creating and managing Ember.js applications. Developed to streamline the development process, Ember CLI offers various commands that help developers efficiently handle different tasks associated with building robust web applications using Ember.js. Below, we explore several use cases of the ’ember’ command, providing detailed explanations and motivations for using each.
Create a New Ember Application
Code:
ember new my_new_app
Motivation:
Creating a new Ember application is the starting point for developers looking to build applications using Ember.js. This command sets up a new project directory with all the necessary boilerplate, dependencies, and configurations required to kickstart your Ember project. It simplifies the initial setup process, allowing developers to focus on writing application-specific code rather than fiddling with configurations.
Explanation:
ember
: The main command-line interface tool for Ember.js.new
: The subcommand used to generate a new project.my_new_app
: The name of the new application directory that will be created.
Example output:
installing app
create .editorconfig
create .ember-cli
create .eslintrc.js
...
Successfully created project my_new_app.
Create a New Ember Addon
Code:
ember addon my_new_addon
Motivation:
Using the ’ember addon’ command is crucial for developers intending to create reusable components, utilities, or libraries that can be shared across multiple Ember.js projects. Addons provide a way to package these elements so they can be easily included in other applications.
Explanation:
ember
: Calls the command-line interface for Ember.js.addon
: Specifies that an Ember addon is to be created rather than a full application.my_new_addon
: The name of the new addon directory to be created.
Example output:
installing addon
create .editorconfig
create .ember-cli
create .eslintrc.js
...
Successfully created project my_new_addon.
Build the Project
Code:
ember build
Motivation:
Building the project is necessary for producing a compiled version of your application that can be deployed or tested in a static environment. This process compiles all your files, runs pre-defined build processes, and outputs them into a ‘dist’ (distribution) directory.
Explanation:
ember
: Invokes the Ember CLI.build
: The subcommand that compiles the application into optimized, production-ready files.
Example output:
Building...
Built project successfully. Stored in "dist/".
Build the Project in Production Mode
Code:
ember build -prod
Motivation:
Building your project in production mode ensures that optimizations for deployment are applied, resulting in smaller, more efficient code. It typically involves minifying JavaScript, stripping debugging information, and other performance improvements.
Explanation:
ember
: Utilizes the Ember.js command-line interface.build
: Specifies a build operation.-prod
: Denotes that the build is specifically for production, applying optimizations like minification.
Example output:
Building (production)...
Built project successfully. Stored in "dist/".
Run the Development Server
Code:
ember serve
Motivation:
Running the development server is essential for real-time testing and development. It serves your application at a local web server address (typically http://localhost:4200/), enabling you to see changes immediately as you develop.
Explanation:
ember
: Calls the command-line interface for Ember.js.serve
: Launches a local development server, providing live reload capabilities.
Example output:
Serving on http://localhost:4200/
Run the Test Suite
Code:
ember test
Motivation:
Testing is a core part of the software development process, and this command facilitates running automated tests across your application. It allows developers to ensure that their codebase remains robust and free from regressions as changes are made.
Explanation:
ember
: Engages the Ember CLI.test
: Executes all configured tests for your application.
Example output:
1..10
# tests 10
# pass 10
# ok
Run a Blueprint to Generate Something like a Route or Component
Code:
ember generate type name
Motivation:
Generating a route, component, or other resources in an Ember application greatly speeds up development by creating all necessary files and boilerplate automatically. It enforces consistency across the codebase by adhering to Ember’s conventions.
Explanation:
ember
: Invokes the CLI tool.generate
: The subcommand used to create new files using blueprints.type
: Specifies the type of resource to generate, such as aroute
orcomponent
.name
: The name of the resource being generated.
Example output (for a component named “my-component”):
installing component
create app/components/my-component.hbs
create app/components/my-component.js
Install an Ember-CLI Addon
Code:
ember install name_of_addon
Motivation:
Installing add-ons extends the functionality of an Ember application without having to write everything from scratch. These add-ons can add features like authentication, UI components, or other utilities and are shared within the Ember community.
Explanation:
ember
: The command-line tool for Ember.js.install
: Subcommand to add new add-ons to your project.name_of_addon
: The name of the Ember CLI add-on you want to install.
Example output:
Installed packages for tooling via npm.
Installed addon package.
Conclusion
Ember CLI greatly facilitates various aspects of Ember.js application development. Its robust command-line interface provides commands for creating applications, running servers, generating components, and more. This command-line tool is designed to make the development process more efficient, consistent, and intuitive, reducing the setup time and allowing developers to focus on creating functional, high-quality web applications.