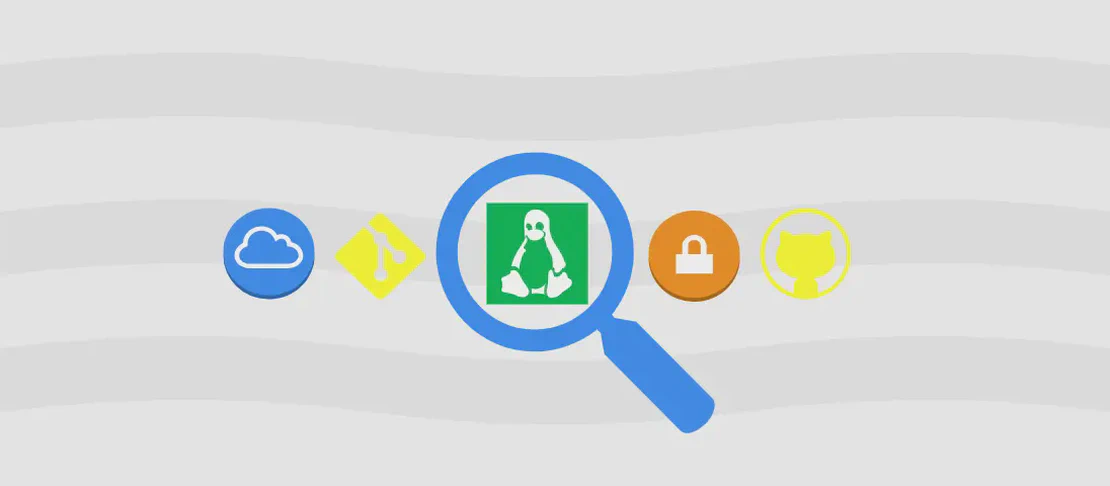
Mastering the Command 'esbuild' (with Examples)
Esbuild is a highly efficient JavaScript bundler and minifier designed for speed. It consolidates multiple JavaScript files into a single file, optimizing them for faster loading by minifying the code and generating source maps for easier debugging. This utility excels in swiftly transforming and serving JavaScript and JSX files, catering to various needs from development to production environments.
Use Case 1: Bundle a JavaScript Application and Print to stdout
Code:
esbuild --bundle path/to/file.js
Motivation: When working with a JavaScript application involving multiple files, combining them into a single executable file simplifies deployment and reduces load times. Displaying the bundled code directly to stdout
is helpful for quick checks or testing purposes.
Explanation:
--bundle
: Instructs esbuild to process all imported modules and their dependencies into one consolidated file.path/to/file.js
: The entry point file for the bundling process, whose imports will be recursively analyzed and compiled together.
Example Output: The output would be the bundled JavaScript code printed directly to the terminal, providing a merged view of all dependencies involved in the application.
Use Case 2: Bundle a JSX Application from stdin
Code:
esbuild --bundle --outfile=path/to/out.js < path/to/file.jsx
Motivation: Sometimes it is necessary to feed input directly from other processes or files using stdin
. This use case is ideal in such scenarios as when JSX files need to be dynamically processed and immediately written to a specified output.
Explanation:
--bundle
: Identifies that the provided JSX file and its imports should be processed into a single output.--outfile=path/to/out.js
: Specifies the location where the bundled file should be saved.< path/to/file.jsx
: Redirects the contents offile.jsx
to esbuild viastdin
, useful for piping content from one command to another.
Example Output:
The result would be a new JS file at path/to/out.js
containing the transformed, bundled content of your JSX application.
Use Case 3: Bundle and Minify a JSX Application with Source Maps in Production Mode
Code:
esbuild --bundle --define:process.env.NODE_ENV=\"production\" --minify --sourcemap path/to/file.js
Motivation: Preparing a web application for a production environment usually involves minifying the code to decrease file size and improve load time while providing a source map for debugging purposes.
Explanation:
--bundle
: Gathers all included modules into a single file.--define:process.env.NODE_ENV=\"production\"
: Replaces occurrences ofprocess.env.NODE_ENV
in the code with “production,” often used to include optimized production code paths.--minify
: Reduces the file size by eliminating whitespace and comments, and shortening variable names.--sourcemap
: Generates source maps to aid in debugging by mapping the minified code back to the original source code.path/to/file.js
: Main file to be bundled alongside its dependencies.
Example Output: The output will be a minified JS file with an accompanying source map file, allowing streamlined debugging even when minification is applied.
Use Case 4: Bundle a JSX Application for a Comma-Separated List of Browsers
Code:
esbuild --bundle --minify --sourcemap --target=chrome58,firefox57,safari11,edge16 path/to/file.jsx
Motivation: Targeting specific browser versions ensures compatibility and uses features supported natively by those browsers, ultimately improving the performance and security of web applications.
Explanation:
--bundle
: Compile and package the dependencies of the JSX file.--minify
: Compresses the code to decrease size.--sourcemap
: Creates maps to troubleshoot issues while inspecting minified code.--target=chrome58,firefox57,safari11,edge16
: Specifies the targeted browser versions, allowing esbuild to optimize the output for those environments.path/to/file.jsx
: Entry file to begin the bundling and minification process.
Example Output: The end product is a bundled JavaScript file optimized for the specified browsers, which should work seamlessly across all of them.
Use Case 5: Bundle a JavaScript Application for Specific Node Version
Code:
esbuild --bundle --platform=node --target=node12 path/to/file.js
Motivation: Deploying a JavaScript application for server environments often calls for targeting a specific Node.js version to ensure package compatibility and leverage Node-specific optimizations.
Explanation:
--bundle
: Consolidates the JavaScript files into a single output file.--platform=node
: Instructs esbuild to generate output intended for Node.js instead of a browser environment.--target=node12
: Indicates that the code will run on Node.js version 12, aligning with its feature set and syntax capabilities.path/to/file.js
: Specifies the entry file to be bundled alongside all its dependencies.
Example Output: Generates a JavaScript file optimized for execution in Node.js version 12, ensuring compatibility with the feature set of that particular version.
Use Case 6: Bundle a JavaScript Application Enabling JSX Syntax in .js Files
Code:
esbuild --bundle app.js --loader:.js=jsx path/to/file.js
Motivation: Sometimes JavaScript files use JSX syntax conventionally used within React projects. This example demonstrates accommodating JSX syntax specifically within .js
files.
Explanation:
--bundle
: Directs esbuild to package all dependencies.app.js
: The entry point that initially loads the other modules.--loader:.js=jsx
: Configures esbuild to handle.js
files containing JSX code.path/to/file.js
: Specifies the source file which may contain JSX to be bundled.
Example Output: A JavaScript bundle that accurately interprets and processes JSX syntax within .js files, ready to be executed or further processed.
Use Case 7: Bundle and Serve a JavaScript Application on an HTTP Server
Code:
esbuild --bundle --serve=port --outfile=index.js path/to/file.js
Motivation: During development, it’s invaluable to bundle, serve, and see changes reflected instantly in a browser without the necessity of a separate server configuration.
Explanation:
--bundle
: Consolidates JavaScript files.--serve=port
: Starts an HTTP server on the specified port, serving the bundled file and any changes in real-time.--outfile=index.js
: Specifies output destination for the bundled code.path/to/file.js
: Entry file to start the bundling process.
Example Output: The command outputs a bundled JavaScript file and starts a local server. Any modifications to the files will be served live, enhancing the development process.
Use Case 8: Bundle a List of Files to an Output Directory
Code:
esbuild --bundle --outdir=path/to/output_directory path/to/file1 path/to/file2 ...
Motivation: Bundling multiple JavaScript files into a designated output directory is crucial for organizing and segregating different builds, particularly in larger projects or multitenant applications.
Explanation:
--bundle
: Directs esbuild to package multiple files.--outdir=path/to/output_directory
: Designates the output directory where bundled files are stored.path/to/file1
,path/to/file2
,...
: List of entry files to be bundled separately or together based on references.
Example Output: An organized set of bundled files located within the specified directory path, streamlining the deployment process across environments.
Conclusion
The esbuild
utility presents extensive options for bundling JavaScript and JSX applications, ensuring speed and efficiency across diverse scenarios. Through targeted examples, developers can leverage esbuild for quick bundling, minification, and development server needs, seamlessly incorporating it into both development and production workflows. The outlined use cases facilitate a cohesive understanding of optimizing web applications tailored to specific browser and environment requirements.