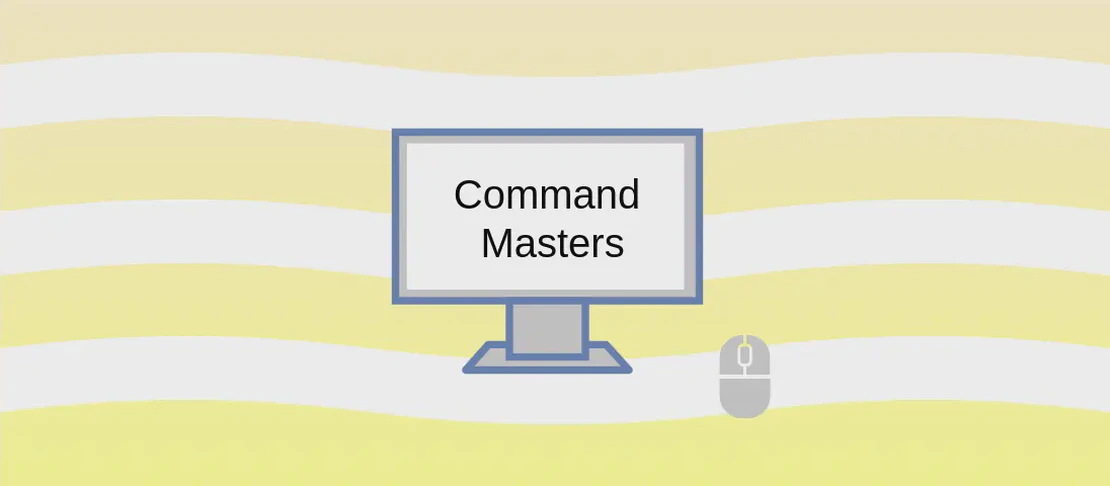
How to Use the ESLint Command (with Examples)
ESLint is a powerful tool for identifying and fixing potential issues in your JavaScript and JSX code. It enforces coding standards and helps maintain code quality by analyzing your code statically. Extensible and configurable, ESLint is essential in various development environments to ensure consistent coding styles, avoid potential bugs, and improve the overall maintainability of codebases.
Use case 1: Creating the ESLint Configuration File
Code:
eslint --init
Motivation:
Setting up ESLint is the first step toward integrating it into your development workflow. By creating a configuration file with the eslint --init
command, you can customize the rules and environments ESLint will enforce. This step is crucial for aligning ESLint’s checks with the specific style guide your team follows or adapting to the project’s unique needs. Configuring ESLint helps ensure that all developers on the project adhere to the same coding standards.
Explanation:
eslint
: The command to run ESLint.--init
: This option initializes an interactive process where ESLint asks a series of questions. Based on your responses, ESLint generates a configuration file (.eslintrc
) tailored to your project’s specific requirements. The configuration may include extending popular style guides, such as Airbnb, Google, or StandardJS, choosing between JavaScript or JSON format for the config file.
Example Output:
You can also create a new configuration file by answering a few questions about your project.
? How would you like to use ESLint? (Use arrow keys)
❯ To check syntax only
To check syntax and find problems
To check syntax, find problems, and enforce code style
Use case 2: Linting One or More Files
Code:
eslint path/to/file1.js path/to/file2.js ...
Motivation:
Linting specific files or directories is a common need for developers who want to ensure that portions of their code adhere to established coding standards. This approach can be useful during development when you are working on certain files or when reviewing changes made to specific parts of the codebase. Running ESLint on individual files helps isolate issues and avoid overwhelming outputs from scanning the entire project, improving focus and efficiency.
Explanation:
eslint
: The command for running ESLint.path/to/file1.js path/to/file2.js
: These are the paths to the JavaScript files you wish to lint. You can specify multiple files or directories, allowing ESLint to check each one for issues based on the configured rules.
Example Output:
/path/to/file1.js
1:1 error Unexpected console statement no-console
✖ 1 problem (1 error, 0 warnings)
Use case 3: Fixing Lint Issues
Code:
eslint --fix
Motivation:
The --fix
option is incredibly powerful because it automates the resolution of problems identified by ESLint where it can. This functionality is especially useful for quickly addressing common stylistic issues or simple errors, significantly reducing the manual effort required to correct minor deviations from the coding standards. This auto-fix feature helps maintain code quality while saving developers time.
Explanation:
eslint
: The main command used to invoke ESLint.--fix
: This option tells ESLint to automatically correct issues where possible. It only applies fixes that ESLint knows are safe to make without further input, such as adjusting spacing, semicolons, quotes, etc.
Example Output:
/path/to/file1.js 2ms
✖ 5 problems (0 errors, 5 warnings)
5 warnings automatically fixed
Use case 4: Linting Using a Specified Configuration File
Code:
eslint -c path/to/config_file path/to/file1.js path/to/file2.js
Motivation:
In projects where multiple configurations are used or when configuring ESLint for different environments or team members, explicitly specifying which ESLint configuration file to use is invaluable. This practice allows developers to switch seamlessly between different linting rules without altering the project’s primary configuration setup, thus facilitating diverse coding environments within the same project.
Explanation:
eslint
: This initiates the ESLint command.-c path/to/config_file
: This option specifies a custom configuration file. It allows you to override the default configuration settings and force ESLint to utilize this file’s settings.path/to/file1.js path/to/file2.js
: These are the paths to files you want ESLint to lint using the specified configuration file.
Example Output:
/path/to/file1.js
1:5 warning Unexpected alert no-alert
✖ 1 problem (0 errors, 1 warning)
0 warnings automatically fixed
Conclusion:
Understanding and effectively using ESLint can greatly enhance a project’s code quality, readability, and maintainability. By utilizing these commands, developers can establish consistent coding practices throughout their projects, effectively identify issues, and automate the correction of coding errors. This guide to ESLint use cases provides a comprehensive foundation for leveraging its utility in any JavaScript development environment.