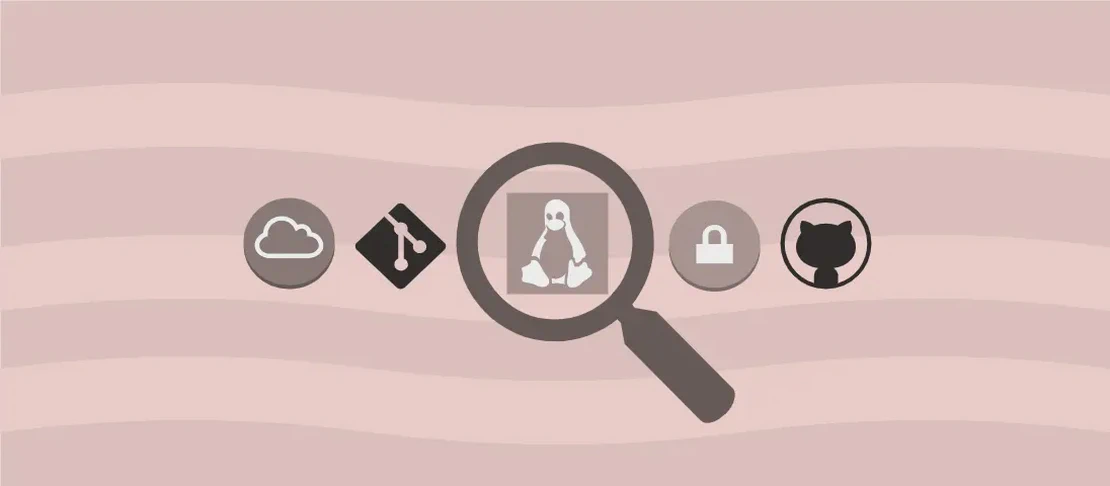
How to use the command 'eval' (with examples)
The eval
command is a powerful built-in shell utility that interprets and executes the arguments passed to it as a single command line in the current shell environment. By leveraging eval
, you can dynamically construct and execute commands, which can be especially useful in scripting and when you want to manipulate or evaluate variables or commands on the fly. It’s important, however, to use eval
judiciously as it can pose security risks if used with untrusted input.
Use case 1: Call echo
with the “foo” argument
Code:
eval "echo foo"
Motivation:
The first use case demonstrates a simple execution of the echo
command using eval
. The primary motivation for using eval
in conjunction with echo
might appear trivial in this case, but it serves as an introductory illustration to show how eval
can process its argument as a full command. This is particularly useful in scripting scenarios where commands need to be constructed dynamically. eval
allows the command to be executed in the current shell context, ensuring that any environment variables or command outputs generated are accessible within the same execution shell.
Explanation:
eval
: This is the command that takes the following string and executes it as if it were a command typed directly into the shell. It allows for the evaluation of complex dynamic command setups."echo foo"
: This is the argument toeval
. Theecho
command is used for outputting text to the terminal, and here it is instructed to printfoo
. Enclosingecho foo
in quotes ensures that it is treated as a single string argument passed toeval
.
Example output:
foo
This output comes from the echo
command inside the eval
execution, printing the string “foo” to the terminal.
Use case 2: Set a variable in the current shell
Code:
eval "foo=bar"
Motivation:
The second use case demonstrates how eval
can be used to define variables within the current shell environment. One of the main motivations for using eval
in this context is its ability to manipulate shell variables dynamically. When you are writing scripts that require variable names or values determined at runtime, eval
can be used to construct the variable declaration strings and execute them. Using eval
, you ensure that the variable modification takes place in the current shell environment, making it accessible immediately without needing subprocesses.
Explanation:
eval
: This command will interpret the provided string as a shell command. The capability ofeval
to interpret strings allows it to execute dynamic constructs, which can be essential for advanced shell scripts."foo=bar"
: This string is evaluated byeval
, which treats it as a shell command to assign the stringbar
to the variablefoo
. The quotes are crucial in ensuring the string is handled correctly as a single command input byeval
.
Example output:
There is no visible output from this command directly, as it performs a variable assignment. However, you can verify the assignment by subsequently using the echo
command:
echo $foo
This would result in:
bar
Indicating that the variable foo
has been correctly assigned the value bar
within the current shell session.
Conclusion:
The eval
command is a versatile tool within the shell that can be leveraged for the dynamic execution of commands in the current shell environment. Through the examples, we explored how to use eval
for executing basic commands and setting variables dynamically. These use cases exemplify the utility of eval
in scripting and command-line operations. However, due to its capability of executing arbitrary code, care should be taken to ensure its arguments are sanitized, especially when dealing with input from untrusted sources.