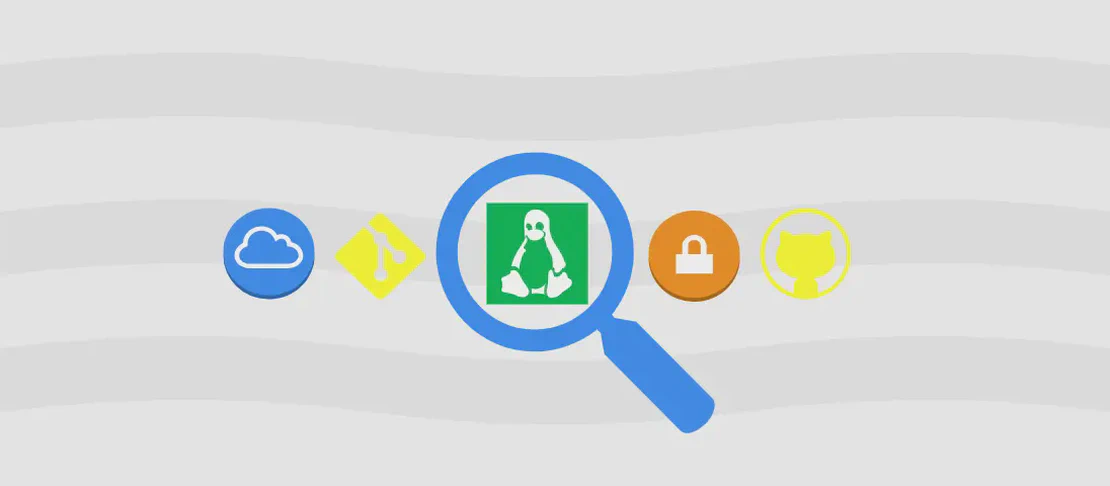
How to use the command 'export' (with examples)
The export
command is a staple of UNIX and Linux environments, enabling users to pass environment variables from the shell to child processes. This command plays a fundamental role in defining how applications access certain operating system features, and it allows users to set or modify those variables in their shell environment. Exporting variables ensures different processes can communicate effectively by sharing configuration details crucial for their operations.
Use case 1: Set an environment variable
Code:
export VARIABLE=value
Motivation:
Setting environment variables is a fundamental task when configuring applications and processes in Unix-like operating systems. For example, when a program requires certain configuration parameters, such as a port number, API keys, or directory locations, these can be efficiently set as environment variables. This approach provides a flexible and centralized way to manage configuration changes without modifying the application code itself, thus promoting separation of concerns and enhancing security.
Explanation:
export
: This command is used to define an environment variable and make it available to child processes spawned from the current shell. By exporting, you ensure that any programs or scripts executed from this point inherit the declared variable and its value.VARIABLE=value
: Here,VARIABLE
is the name of the environment variable you wish to create or modify, andvalue
is the string or numeric value assigned to this variable. For instance, settingDATABASE_URL
to point to a database connection string eases application connections to databases.
Example Output:
When you execute the above command in a terminal, there isn’t a direct output. However, you can verify the export by using echo
:
echo $VARIABLE
Typing the above after setting the variable will show: value
, confirming the environment variable has been correctly set and can be accessed by other commands and processes initiated from this shell.
Use case 2: Append a pathname to the environment variable PATH
Code:
export PATH=$PATH:path/to/append
Motivation:
Appending a directory to the PATH
environment variable is a common practice among developers and system administrators. The PATH
variable contains directories where the system looks for executable files. By adding a new directory, you make the executables in that directory accessible from anywhere in the shell without needing to specify their full path. This is especially useful when installing software that resides in localized folders or when developing scripts and executables that need to be accessed globally across your system.
Explanation:
export
: Similar to the previous use case, the command is used to ensure that the changes toPATH
are available to child processes. This inclusion is crucial as it modifies the environment for any future commands that might require executables located in the newly added directory.PATH=$PATH:path/to/append
: Here,PATH
is being redefined to include existing directories (denoted by$PATH
) and a new directorypath/to/append
.$PATH
is a placeholder that expands to the current value of thePATH
variable, ensuring the existing directories are retained while the new path is appended.
Example Output:
Just like the first example, executing this export will not produce direct terminal output. However, to verify the change, you can type:
echo $PATH
The output will show the original paths along with path/to/append
included at the end, confirming the variable was updated correctly. This extended PATH
ensures that executables in path/to/append
are reachable without specifying their full path.
Conclusion:
The export
command is a powerful asset for managing environment variables, critical for system configurations and application operations. Through examples of setting a simple environment variable and extending the PATH
variable, we’ve illustrated how this command facilitates flexible and consistent shell environments. Understanding and utilizing export
effectively in a shell script or an interactive shell session significantly enhances both the usability and portability of applications and scripts.