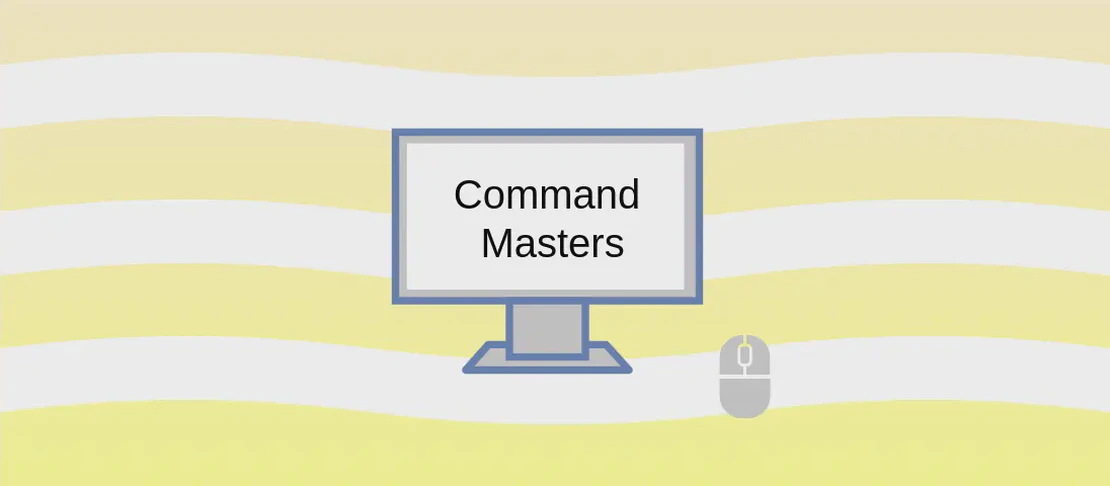
How to use the command 'export' (with examples)
- Linux
- December 17, 2024
The export
command in Unix and Linux systems is a bash shell built-in utility that is used to set environment variables, affecting the current shell and, importantly, any child processes started from it. Exported variables persist across forked processes, providing a way to pass data or configuration settings from the shell to child processes. This command is essential for managing the environment in which programs run, influencing their behavior and accessibility to resources.
Use case 1: Setting an Environment Variable
Code:
export VARIABLE=value
Motivation:
Setting environment variables is crucial in configuring the working environment for applications. For instance, when you need a specific configuration for a program, such as specifying where a program should look for its configuration files or setting the language, you can use an environment variable. Once set, these variables can be accessed by any child process and help standardize settings across different tools or scripts without hardcoding values.
Explanation:
export
: This command is used to set the environment variable so that it is available in all the child processes of the shell.VARIABLE=value
: Here,VARIABLE
is the name of the environment variable you want to create or modify, andvalue
is the string or number you want to assign to this variable.
Example Output:
After executing, there is no visible direct output. However, the environment variable VARIABLE
will now be part of the environment and can be accessed using echo $VARIABLE
in child processes, showing the assigned value
.
Use case 2: Unsetting an Environment Variable
Code:
export -n VARIABLE
Motivation:
There are situations where you need to ensure that certain environment variables do not propagate to child processes. This might include security-sensitive data or cases where different tasks should not interfere with each other’s environment settings. Removing such variables from the environment can prevent undesired behavior and conflicts.
Explanation:
export
: Utilized to manage environment variables for child processes.-n
: This option is specifically used to remove the export property from a variable, effectively unexporting it.VARIABLE
: The name of the environment variable that you want to unexport, making it no longer available to new processes.
Example Output:
No direct output is generated. If you try to access VARIABLE
in a child process afterward, it will not be available, indicating successful unsetting with no inherited context.
Use case 3: Exporting a Function to Child Processes
Code:
export -f FUNCTION_NAME
Motivation:
Functions in a shell script are typically scoped to the script itself. However, there could be instances where functions need to be reusable across multiple scripts or sessions without duplicating code. By exporting functions, you allow child processes to access and use these reusable pieces of logic, promoting code reuse and modularity.
Explanation:
export
: Manages exporting options within a shell context.-f
: The-f
option signals that a shell function is being exported, rather than a variable.FUNCTION_NAME
: This is the name of the function you want to export, allowing any new shell instances or scripts called within the present shell to access it.
Example Output:
As with other export operations, there is no textual output. However, in a new shell session or script, you will be able to call FUNCTION_NAME
, demonstrating its accessibility beyond the original scope.
Use case 4: Appending a Pathname to the Environment Variable PATH
Code:
export PATH=$PATH:path/to/append
Motivation:
Modifying the PATH
environment variable allows users to define common directories where executable files are located, so these can be run without specifying their full path. Appending to PATH
is often required when installing new software or scripts, ensuring that they can be accessed conveniently from the terminal.
Explanation:
export
: Used to modify the environment for the current session and child processes.PATH
: This is a special environment variable in Unix systems that defines a list of paths to search for executable files.=$PATH:path/to/append
:=$PATH
ensures the existing paths remain;path/to/append
is the new directory path being added to the list, separated by a colon:
.
Example Output:
No direct output is seen, but you will be able to execute programs located in path/to/append
without needing to type out the full path, confirming the success of the command.
Use case 5: Displaying a List of Active Exported Variables
Code:
export -p
Motivation:
Viewing the active exported variables is vital for debugging and ensuring the correct environment is set for running programs. You can verify what settings are in effect during a session and ensure that only the intended variables are exported, identifying any discrepancies or errors in the environment setup.
Explanation:
export
: Handles the management of variables and their export status.-p
: This option prints all the exported environment variables in the current shell session, each in a form that is ready to be reused as input to the shell.
Example Output:
The shell displays a list of exported variables, like this:
declare -x VARIABLE="value"
declare -x PATH="/usr/local/bin:/usr/bin:/bin:...:path/to/append"
This output helps assess which variables are exported and available to child processes at any time.
Conclusion
The export
command is a versatile tool in shell environments, providing the means to manage environment variable inheritance efficiently. By using export
, users can ensure a consistent and predictable configuration for their shell sessions and subprocesses, streamline function accessibility, and effectively manage executions paths by adjusting the PATH
variable. Exploring and understanding each use case allows for greater flexibility and control over the command-line environment.