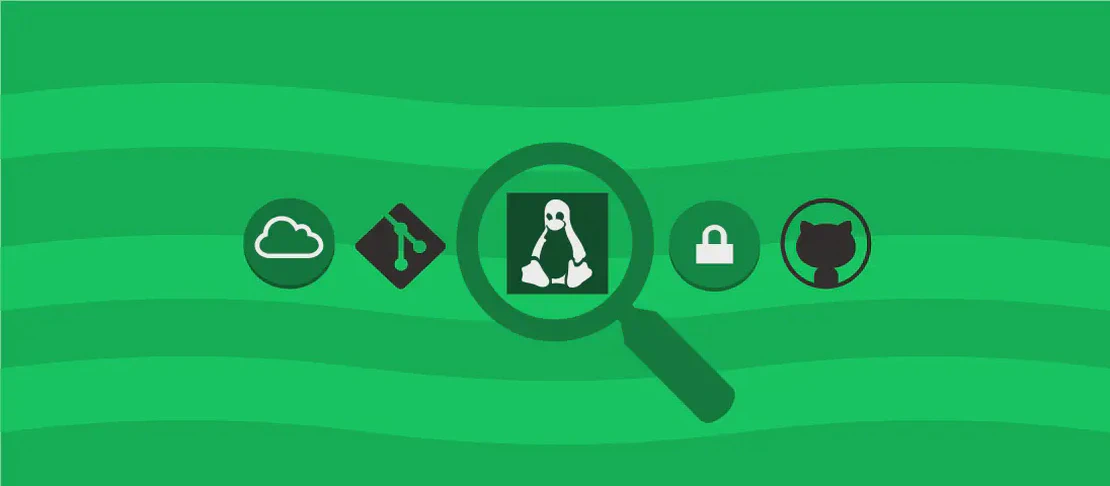
How to Use the Command 'expr' (with Examples)
The expr
command is a tool used in Unix-like systems to evaluate expressions and perform various operations, including mathematical calculations and string manipulations. It is particularly useful in shell scripting, allowing users to handle arithmetic operations and string handling tasks efficiently. The command is part of the GNU Core Utilities package and provides functionalities such as calculating the length of a string, extracting substrings, pattern matching, indexing, and logical and mathematical expressions.
Use case 1: Get the length of a specific string
Code:
expr length "hello"
Motivation:
Determining the length of a string is a common task in programming and scripting, often required when validating input or formatting output. By using the expr
command, one can quickly and easily retrieve the number of characters in a specific string, which is essential for various text processing tasks.
Explanation:
expr
: Invokes theexpr
command.length
: Specifies that the operation is to calculate the length of the string."hello"
: The string whose length is to be calculated.
Example Output:
5
Use case 2: Get the substring of a string with a specific length
Code:
expr substr "hello world" 7 5
Motivation:
Extracting a specific part of a string is often necessary when handling text, such as parsing command output or user input. By utilizing the expr
command for substring extraction, users can quickly retrieve the desired portion of a string based on given starting position and length.
Explanation:
expr
: Invokes theexpr
command.substr
: Specifies the operation to extract a substring."hello world"
: The original string from which the substring will be extracted.7
: The position (1-based index) from which to start the extraction.5
: The number of characters to extract.
Example Output:
world
Use case 3: Match a specific substring against an anchored pattern
Code:
expr match "hello world" '^hello'
Motivation:
To validate or search for patterns within strings, users might need to identify whether a specific pattern appears at the start of a string. expr match
provides a straightforward way to perform such anchored pattern matching, which is essential for tasks like input validation and data analysis.
Explanation:
expr
: Invokes theexpr
command.match
: Specifies the operation to match a pattern."hello world"
: The string in which to perform the pattern matching.'^hello'
: An anchored pattern (using the caret^
to denote the start) to be matched.
Example Output:
5
Use case 4: Get the first char position from a specific set in a string
Code:
expr index "chimpanzee" "aeiou"
Motivation:
Checking for the first occurrence of any character from a set is useful in many situations, such as finding the position of vowels in a word or locating delimiter characters. The expr index
function allows users to determine the position of the first occurrence of given characters quickly.
Explanation:
expr
: Invokes theexpr
command.index
: Indicates the operation to find the position of characters."chimpanzee"
: The string in which to search."aeiou"
: A set of characters whose first occurrence’s position needs to be identified in the target string.
Example Output:
2
Use case 5: Calculate a specific mathematical expression
Code:
expr 15 + 27
Motivation:
Performing arithmetic calculations directly in shell scripts is a common requirement, ranging from simple calculations to complex numeric logic. By using expr
, users can execute basic arithmetic operations, essential for automating tasks that require numeric data processing.
Explanation:
expr
: Invokes theexpr
command.15
: The first number in the arithmetic expression.+
: The arithmetic operator for addition.27
: The second number in the arithmetic expression.
Example Output:
42
Use case 6: Get the first expression if its value is non-zero and not null otherwise get the second one
Code:
expr 0 \| 5
Motivation:
Evaluating logical conditions and returning appropriate values based on operands can be crucial in conditional scripting. Using expr
with the logical OR operator provides an efficient way to determine which non-zero value should be selected, supporting decision-making logic in scripts.
Explanation:
expr
: Invokes theexpr
command.0
: The first expression to evaluate.\|
: A logical OR operator (\
is used to escape the pipe character in the shell).5
: The second expression to evaluate if the first expression is zero or null.
Example Output:
5
Use case 7: Get the first expression if both expressions are non-zero and not null otherwise get zero
Code:
expr 3 \& 4
Motivation:
Logical AND evaluations, particularly in scripting, play an integral role in determining the conditions under which specific blocks of code should execute. Implementing such logic using expr
enables the conditional processing based on multiple criteria being satisfied simultaneously.
Explanation:
expr
: Invokes theexpr
command.3
: The first expression for logical evaluation.\&
: A logical AND operator (\
is used to escape the ampersand character in the shell).4
: The second expression for evaluation.
Example Output:
3
Conclusion:
The expr
command is a powerful utility within Unix-like operating systems, allowing users to perform a wide array of string manipulations and arithmetic operations. Its versatility in handling both numeric and textual data makes it a valuable tool for shell scripting, facilitating efficient data processing and logic implementation with minimal scripting effort. Whether for extracting substrings, calculating string lengths, performing arithmetic, or taking logical decisions, expr
has diverse applications that can simplify many aspects of system administration and programming tasks.