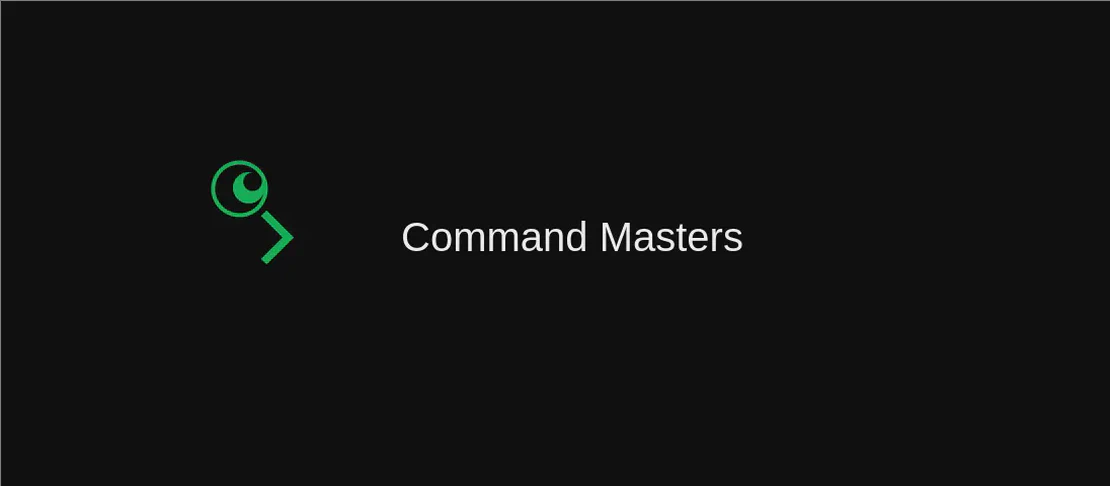
How to use the command 'exrex' (with examples)
The ’exrex’ command is a tool that generates all possible or random matching strings for a given regular expression. It can also simplify regular expressions. This tool is useful for various purposes, including testing regular expressions, generating test data, or exploring different string patterns.
Use case 1: Generate all possible strings that match a regular expression
Code:
exrex 'regular_expression'
Motivation: One might want to generate all possible strings that match a specific regular expression for testing or validation purposes.
Explanation: This command takes a regular expression as an argument and generates all possible strings that match the given expression.
Example output:
For the regular expression ab*c
, the command exrex 'ab*c'
will generate the following strings:
ac
abc
abbc
abbbc
...
Use case 2: Generate a random string that matches a regular expression
Code:
exrex --random 'regular_expression'
Motivation: Generating a random string that satisfies a regular expression can be useful for creating random data for testing or simulation purposes.
Explanation:
The --random
flag generates a single random string that matches the given regular expression.
Example output:
For the regular expression [0-9]{3}-[0-9]{3}-[0-9]{4}
, the command exrex --random '[0-9]{3}-[0-9]{3}-[0-9]{4}'
might generate a random phone number such as 987-654-3210
.
Use case 3: Generate a specific number of strings that match a regular expression
Code:
exrex --max-number 100 'regular_expression'
Motivation: Generating a limited number of strings that match a regular expression can be useful when working with large or complex regular expressions and only a subset of matches is required.
Explanation:
The --max-number
flag specifies the maximum number of strings to generate that match the given regular expression.
Example output:
For the regular expression [A-Za-z]{10}
, the command exrex --max-number 5 '[A-Za-z]{10}'
will generate five random strings with ten alphabetic characters each, such as GlPmuDAgED
.
Use case 4: Generate all possible strings that match a regular expression, joined by a custom delimiter string
Code:
exrex --delimiter ", " 'regular_expression'
Motivation: In some cases, it is helpful to generate all possible strings that match a regular expression and join them together with a custom delimiter for further processing or displaying.
Explanation:
The --delimiter
flag specifies the delimiter string to be used when joining the generated strings.
Example output:
For the regular expression [0-9]{2}
, the command exrex --delimiter ", " '[0-9]{2}'
will generate all possible two-digit numbers and join them with a comma and a space delimiter, resulting in the output 00, 01, 02, 03, ..., 99
.
Use case 5: Print count of all possible strings that match a regular expression
Code:
exrex --count 'regular_expression'
Motivation: Obtaining the count of all possible strings that match a regular expression can be helpful to estimate the number of matches or understand the complexity of the regular expression.
Explanation:
The --count
flag prints the count of all possible strings that match the given regular expression.
Example output:
For the regular expression [A-Za-z]{3}
, the command exrex --count '[A-Za-z]{3}'
will output 26^3 = 17,576
, indicating that there are 17,576 possible three-letter combinations using uppercase and lowercase letters.
Use case 6: Simplify a regular expression
Code:
exrex --simplify 'ab|ac'
Motivation: Regular expressions can sometimes become complex and hard to read. Simplifying a regular expression allows for easier understanding and maintenance.
Explanation:
The --simplify
flag simplifies the given regular expression.
Example output:
For the regular expression ab|ac
, the command exrex --simplify 'ab|ac'
will output a[b|c]
, which represents a choice between ab
and ac
in a simplified form.
Additional use cases
In addition to the main use cases provided above, the ’exrex’ command can also be used for more playful purposes, such as printing eyes or a boat.
Code:
exrex '[oO0](_)[oO0]'
Explanation:
The regular expression [oO0](_)[oO0]
matches patterns like o_o
or 0_0
, which resemble eyes.
Code:
exrex '( {20}(\| *\\|-{22}|\|)|\.={50}| ( ){0,5}\\\.| {12}~{39})'
Explanation:
The regular expression ( {20}(\| *\\|-{22}|\|)|\.={50}| ( ){0,5}\\\.| {12}~{39})
matches patterns that resemble the shape of a boat when displayed.
Conclusion
The ’exrex’ command is a versatile tool for generating and simplifying regular expressions. Whether it is for testing, data generation, or exploring different string patterns, ’exrex’ provides a convenient way to work with regular expressions and their matches.