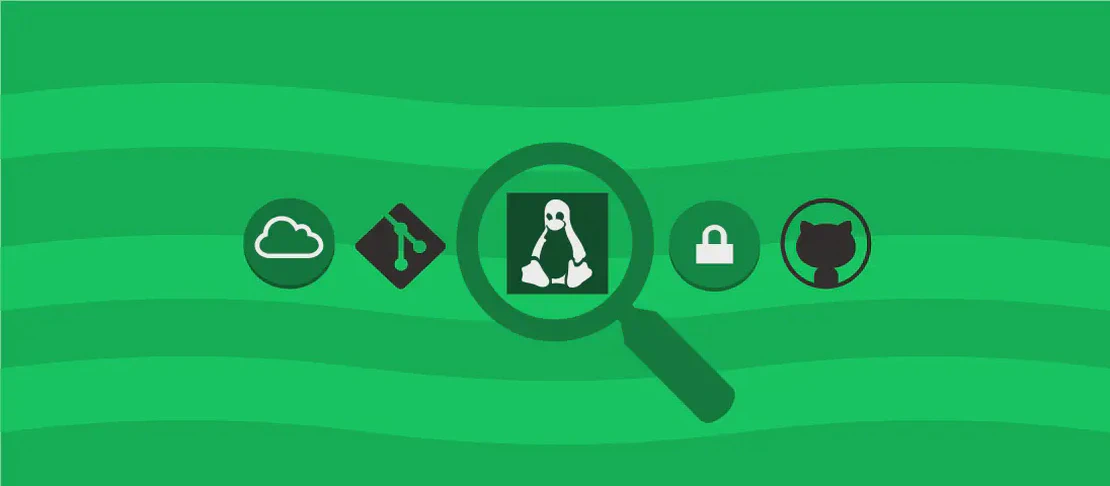
Discovering Fastmod: A Powerhouse for Code Replacements (with examples)
Fastmod is a command-line tool designed to facilitate the process of finding and replacing code patterns across entire codebases with remarkable speed and efficiency. Built as a faster alternative to the codemod tool, it excels in executing these replacements using regular expressions matched by the Rust regex crate. One of its significant advantages is its ability to respect .ignore
and .gitignore
files, ensuring that undesired parts of your project are not unintentionally altered. Here’s an exploration of various use cases where fastmod can significantly enhance coding productivity:
Use case 1: Replace a Regex Pattern in All Files of the Current Directory
Code:
fastmod regex_pattern replacement
Motivation:
Using the first example is beneficial when you need to apply changes across a large codebase uniformly. Unlike manual edits in each file, this command automates and simplifies the process of updating code patterns in all files within the current directory, respecting the .gitignore
and .ignore
files. This can be particularly useful when fixing bugs, refactoring code, or applying new code standards throughout your project.
Explanation:
fastmod
: The command invoked to perform the pattern replacement.regex_pattern
: Represents a regular expression matching the text you want to replace.replacement
: The new text that will replace the found occurrences of the regex pattern.
Example Output:
Upon execution, the command will scan through files in the current directory and replace occurrences of regex_pattern
with replacement
, outputting the files that were modified.
Use case 2: Replace a Regex Pattern in Case-Insensitive Mode in Specific Files or Directories
Code:
fastmod --ignore-case regex_pattern replacement -- path/to/file path/to/directory ...
Motivation:
This use case is advantageous when you need to ensure changes are applied irrespective of the case sensitivity of the text. This feature is crucial when dealing with codebases that might have variations in text casing, ensuring consistency without manually checking and altering each variation.
Explanation:
--ignore-case
: Ensures that the regex pattern matches text regardless of case.regex_pattern
: The case-insensitive regular expression pattern to be replaced.replacement
: The replacement text.--
: Indicates the end of options and the start of positional arguments, followed by specific file or directory paths where the operation should be applied.
Example Output:
Fastmod will traverse the specified files and directories, applying the case-insensitive regex replacement, and then display the list of modified files.
Use case 3: Replace a Regex Pattern in a Specific Directory in Files Filtered with a Case-Insensitive Glob Pattern
Code:
fastmod regex replacement --dir path/to/directory --iglob '**/*.{js,json}'
Motivation:
When you have a targeted replacement in mind for a specific type of file format (e.g., JavaScript or JSON files) within a directory, this command proves extremely efficient. It combines precision in targeting a file type with the power of regex replacement.
Explanation:
regex
: The regex pattern to replace.replacement
: The text to use for replacement.--dir
: Sets the specific directory for the operation.path/to/directory
: The directory in which files are to be examined.--iglob
: Uses a glob pattern for case-insensitive file searching, such as files ending in.js
or.json
.
Example Output:
The command selectively applies the regex replacement to files matching the glob pattern in the specified directory and then provides feedback on the changes.
Use case 4: Replace for an Exact String in .js or JSON Files
Code:
fastmod --fixed-strings exact_string replacement --extensions json,js
Motivation:
Exact string replacement is beneficial when working with known literals, where regex might introduce complexity or errors. This example serves those needing a straightforward swap in specific file types like .js
or .json
.
Explanation:
--fixed-strings
: Disables regex interpretation, treating patterns as literal strings.exact_string
: The precise string to be replaced.replacement
: The string that will replaceexact_string
.--extensions
: Specifies file types to apply the replacement.
Example Output:
Fastmod will replace the exact string in specified file types and list the files that experienced changes.
Use case 5: Replace for an Exact String Without Prompt for a Confirmation
Code:
fastmod --accept-all --fixed-strings exact_string replacement
Motivation:
When performing high-confidence, repetitive replacements across a project, the need for confirmation on each change can be cumbersome. This use case allows for rapid, automatic changes without prompts, suited for quick edits when time is crucial.
Explanation:
--accept-all
: Automatically accepts all changes without requiring user confirmation.--fixed-strings
: Handles the pattern as a non-regex string.exact_string
: The original string intended for replacement.replacement
: The new string to be used.
Example Output:
Fastmod performs an instant replacement across applicable files without additional prompts, showing completion upon finishing the task.
Use case 6: Replace for an Exact String Without Prompt for a Confirmation, Printing Changed Files
Code:
fastmod --accept-all --print-changed-files --fixed-strings exact_string replacement
Motivation:
This variation is ideal when unsupervised automatic replacement is needed, but with an audit trail of updates. Developers can keep track of what files were modified post-execution.
Explanation:
--accept-all
: Bypasses confirmation requests, allowing for swift execution.--print-changed-files
: Outputs a catalog of files that were altered, facilitating transparency and traceability.--fixed-strings
: Ensures the text is regarded as an exact string.
Example Output:
The tool replaces the string swiftly in the specified parts of the codebase, yielding a list of modified files, offering clarity and accountability for the operation conducted.
Conclusion:
Fastmod emerges as an essential tool for developers needing efficient, large-scale codebase modifications. Its capacity to tackle straightforward and complex pattern replacements with various options caters to diverse project requirements, ultimately saving time and reducing errors. Whether dealing with regex or exact string replacements, accounting for case sensitives or file types, fastmod provides the capability and speed modern code editors need.